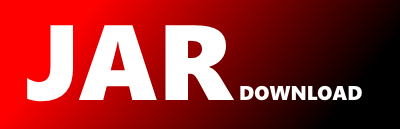
com.arcadedb.query.sql.parser.Statement Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OStatement.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Database;
import com.arcadedb.exception.CommandSQLParsingException;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.InternalExecutionPlan;
import com.arcadedb.query.sql.executor.ResultSet;
import java.util.*;
public class Statement extends SimpleNode {
//only for internal use!!! (caching)
protected Statement originalStatement;
protected String originalStatementAsString;
protected Limit limit = null;
protected Timeout timeout = null;
public static final String CUSTOM_STRICT_SQL = "strictSql";
public Statement(final int id) {
super(id);
}
public void toString(final Map params, final StringBuilder builder) {
throw new UnsupportedOperationException("missing implementation in " + getClass().getSimpleName());
}
public void validate() throws CommandSQLParsingException {
// NO VALIDATION BY DEFAULT
}
@Override
public String toString(final String prefix) {
final StringBuilder builder = new StringBuilder();
toString(null, builder);
return builder.toString();
}
public ResultSet execute(final Database db, final Object[] args) {
return execute(db, args, true);
}
public ResultSet execute(final Database db, final Object[] args, final CommandContext parentContext) {
return execute(db, args, parentContext, true);
}
public ResultSet execute(final Database db, final Map args) {
return execute(db, args, true);
}
public ResultSet execute(final Database db, final Map args, final CommandContext parentContext) {
return execute(db, args, parentContext, true);
}
public ResultSet execute(final Database db, final Object[] args, final boolean usePlanCache) {
return execute(db, args, null, usePlanCache);
}
public ResultSet execute(final Database db, final Object[] args, final CommandContext parentContext, final boolean usePlanCache) {
throw new UnsupportedOperationException();
}
public ResultSet execute(final Database db, final Map args, final boolean usePlanCache) {
return execute(db, args, null, usePlanCache);
}
public ResultSet execute(final Database db, final Map args, final CommandContext parentContext, final boolean usePlanCache) {
throw new UnsupportedOperationException();
}
/**
* creates an execution plan for current statement, with profiling disabled
*
* @param context the context that will be used to execute the statement
*
* @return an execution plan
*/
public InternalExecutionPlan createExecutionPlan(final CommandContext context) {
return createExecutionPlan(context, false);
}
/**
* creates an execution plan for current statement
*
* @param context the context that will be used to execute the statement
* @param profile true to enable profiling, false to disable it
*
* @return an execution plan
*/
public InternalExecutionPlan createExecutionPlan(final CommandContext context, final boolean profile) {
throw new UnsupportedOperationException();
}
public InternalExecutionPlan createExecutionPlanNoCache(final CommandContext context, final boolean profile) {
return createExecutionPlan(context, profile);
}
public Statement copy() {
throw new UnsupportedOperationException("IMPLEMENT copy() ON " + getClass().getSimpleName());
}
public boolean refersToParent() {
throw new UnsupportedOperationException("Implement " + getClass().getSimpleName() + ".refersToParent()");
}
public boolean isIdempotent() {
return false;
}
public boolean isDDL() {
return this instanceof DDLStatement;
}
public boolean executionPlanCanBeCached() {
return false;
}
public String getOriginalStatement() {
if (originalStatementAsString == null)
originalStatementAsString = originalStatement.toString();
return originalStatementAsString;
}
public void setOriginalStatement(final Statement originalStatement) {
if (!originalStatement.equals(this.originalStatement)) {
this.originalStatement = originalStatement;
this.originalStatementAsString = null;
}
}
public Limit getLimit() {
return limit;
}
public void setLimit(final Limit limit) {
if (limit.num.value.longValue() > -1)
this.limit = limit;
}
public Timeout getTimeout() {
return timeout;
}
public void setTimeout(final Timeout timeout) {
if (timeout.val.longValue() > -1)
this.timeout = timeout;
}
}
/* JavaCC - OriginalChecksum=589c4dcc8287f430e46d8eb12b0412c5 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy