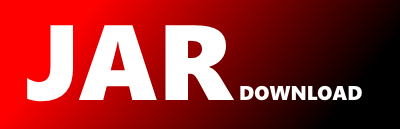
com.arcadedb.query.sql.parser.SuffixIdentifier Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OSuffixIdentifier.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Document;
import com.arcadedb.database.Identifiable;
import com.arcadedb.database.MutableDocument;
import com.arcadedb.database.Record;
import com.arcadedb.exception.CommandExecutionException;
import com.arcadedb.graph.Edge;
import com.arcadedb.graph.Vertex;
import com.arcadedb.query.sql.executor.AggregationContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.Result;
import com.arcadedb.query.sql.executor.ResultInternal;
import com.arcadedb.query.sql.executor.ResultSet;
import java.util.*;
public class SuffixIdentifier extends SimpleNode {
protected Identifier identifier;
protected RecordAttribute recordAttribute;
protected boolean star = false;
public SuffixIdentifier(final int id) {
super(id);
}
public SuffixIdentifier(final Identifier identifier) {
this.identifier = identifier;
}
public SuffixIdentifier(final RecordAttribute attr) {
this.recordAttribute = attr;
}
public void toString(final Map params, final StringBuilder builder) {
if (identifier != null) {
identifier.toString(params, builder);
} else if (recordAttribute != null) {
recordAttribute.toString(params, builder);
} else if (star) {
builder.append("*");
}
}
public Object execute(final Identifiable iCurrentRecord, final CommandContext context) {
if (star) {
return iCurrentRecord;
}
if (identifier != null) {
final String varName = identifier.getStringValue();
if (context != null && varName.startsWith("$") && context.getVariable(varName) != null)
return context.getVariable(varName);
if (iCurrentRecord != null)
return ((Document) iCurrentRecord.getRecord()).get(varName);
return varName;
}
if (recordAttribute != null) {
if ("@rid".equalsIgnoreCase(recordAttribute.name))
return iCurrentRecord.getIdentity();
else if ("@type".equalsIgnoreCase(recordAttribute.name))
return iCurrentRecord.asDocument().getTypeName();
else if ("@cat".equalsIgnoreCase(recordAttribute.name)) {
final Document doc = iCurrentRecord.asDocument();
if (doc instanceof Vertex)
return "v";
else if (doc instanceof Edge)
return "e";
return "d";
}
return ((Document) iCurrentRecord.getRecord()).get(recordAttribute.name);
}
return null;
}
public Object execute(final Result iCurrentRecord, final CommandContext context) {
if (star) {
return iCurrentRecord;
}
if (identifier != null) {
final String varName = identifier.getStringValue();
if (context != null && varName.equalsIgnoreCase("$parent")) {
return context.getParent();
}
if (context != null && (varName.startsWith("$") || varName.startsWith("_$$$")) && context.getVariable(varName) != null) {
final Object result = context.getVariable(varName);
return result;
}
if (iCurrentRecord != null) {
if (iCurrentRecord.hasProperty(varName)) {
return iCurrentRecord.getProperty(varName);
}
if (iCurrentRecord.getMetadataKeys().contains(varName)) {
return iCurrentRecord.getMetadata(varName);
}
if (iCurrentRecord instanceof ResultInternal && ((ResultInternal) iCurrentRecord).getTemporaryProperties().contains(varName)) {
return ((ResultInternal) iCurrentRecord).getTemporaryProperty(varName);
}
}
return null;
}
if (iCurrentRecord != null && recordAttribute != null) {
return recordAttribute.evaluate(iCurrentRecord, context);
}
return null;
}
public Object execute(final Map iCurrentRecord, final CommandContext context) {
if (star) {
final ResultInternal result = new ResultInternal();
if (iCurrentRecord != null) {
for (final Map.Entry x : ((Map) iCurrentRecord).entrySet()) {
result.setProperty("" + x.getKey(), x.getValue());
}
return result;
}
return iCurrentRecord;
}
if (identifier != null) {
final String varName = identifier.getStringValue();
if (context != null && varName.equalsIgnoreCase("$parent")) {
return context.getParent();
}
if (context != null && context.getVariable(varName) != null) {
return context.getVariable(varName);
}
if (iCurrentRecord != null) {
return iCurrentRecord.get(varName);
}
return null;
}
if (recordAttribute != null) {
return iCurrentRecord.get(recordAttribute.name);
}
return null;
}
public Object execute(final Iterable iterable, final CommandContext context) {
if (star) {
return null;
}
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy