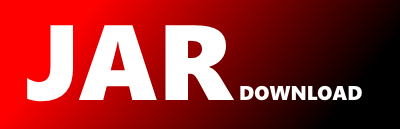
com.arcbees.chosen.client.ChosenOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwtchosen Show documentation
Show all versions of gwtchosen Show documentation
GwtChosen is the GWT port of the Chosen javascript plugin that makes long, unwieldy select boxes much
more user-friendly.
/**
* Copyright 2015 ArcBees Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.arcbees.chosen.client;
import com.arcbees.chosen.client.resources.Resources;
import com.google.gwt.dom.client.Element;
public class ChosenOptions {
private boolean allowSingleDeselect;
private int disableSearchThreshold;
private int maxSelectedOptions;
private String noResultsText;
private String placeholderText;
private String placeholderTextMultiple;
private String placeholderTextSingle;
private Resources resources;
private boolean searchContains;
private boolean singleBackstrokeDelete;
private boolean highlightSearchTerm;
private ResultsFilter resultFilter;
private DropdownPosition dropdownPosition;
private Element dropdownBoundaries;
private DropdownBoundariesProvider dropdownBoundariesProvider;
private int mobileViewportMaxWidth;
private String oneSelectedTextMultipleMobile;
private String manySelectedTextMultipleMobile;
private boolean mobileAnimation;
private int mobileAnimationSpeed;
public ChosenOptions() {
setDefault();
}
public int getDisableSearchThreshold() {
return disableSearchThreshold;
}
public String getManySelectedTextMultipleMobile() {
return manySelectedTextMultipleMobile;
}
public String getOneSelectedTextMultipleMobile() {
return oneSelectedTextMultipleMobile;
}
/**
* Set the number of items needed to show and enable the search input. This option is when the Chosen component is
* used in "multiple select" mode or when a custom ResultFilter is used.
*
* @param disableSearchThreshold number of items needed to show and enable the search input
*/
public ChosenOptions setDisableSearchThreshold(int disableSearchThreshold) {
this.disableSearchThreshold = disableSearchThreshold;
return this;
}
public Element getDropdownBoundaries() {
return dropdownBoundaries;
}
/**
* When {@link com.arcbees.chosen.client.ChosenOptions#dropdownPosition} is set to
* {@link com.arcbees.chosen.client.DropdownPosition#AUTO}, this element will be used to calculate the available
* vertical space below the dropdown.
*/
public ChosenOptions setDropdownBoundaries(Element dropdownBoundaries) {
this.dropdownBoundaries = dropdownBoundaries;
return this;
}
public DropdownBoundariesProvider getDropdownBoundariesProvider() {
return dropdownBoundariesProvider;
}
/**
* See {@link com.arcbees.chosen.client.ChosenOptions#setDropdownBoundaries(com.google.gwt.dom.client.Element)}.
* Useful for cases when the {@link com.arcbees.chosen.client.ChosenOptions#dropdownBoundaries} cannot be defined
* when the Chosen widget is built.
*
* {@link com.arcbees.chosen.client.ChosenOptions#setDropdownBoundaries(com.google.gwt.dom.client.Element)} will
* have priority over this setting.
*/
public ChosenOptions setDropdownBoundariesProvider(DropdownBoundariesProvider dropdownBoundariesProvider) {
this.dropdownBoundariesProvider = dropdownBoundariesProvider;
return this;
}
public DropdownPosition getDropdownPosition() {
return dropdownPosition;
}
/**
* Sets the positioning of the dropdown.
* If set to {@link com.arcbees.chosen.client.DropdownPosition#BELOW}, dropdown will be displayed below the input
* box.
* If set to {@link com.arcbees.chosen.client.DropdownPosition#ABOVE}, dropdown will be displayed above the input
* box.
* If set to {@link com.arcbees.chosen.client.DropdownPosition#AUTO}, dropdown will be displayed below the input
* box only if there's enough vertical space between the input box and the bottom of the
* {@link com.arcbees.chosen.client.ChosenOptions#dropdownBoundaries}. Otherwise, the dropdown will be displayed
* above the input box.
*
* If not set, it will default to {@link com.arcbees.chosen.client.DropdownPosition#BELOW}.
*/
public ChosenOptions setDropdownPosition(DropdownPosition dropdownPosition) {
this.dropdownPosition = dropdownPosition;
return this;
}
public int getMaxSelectedOptions() {
return maxSelectedOptions;
}
public ChosenOptions setMaxSelectedOptions(int maxSelectedOptions) {
this.maxSelectedOptions = maxSelectedOptions;
return this;
}
public int getMobileViewportMaxWidth() {
return mobileViewportMaxWidth;
}
/**
* Set the max width threshold below which the component will consider to be displayed on mobile device.
*
* If you want to disable the mobile layout of the component on every device, set the
* mobileViewportMaxWidth
to -1.
*
* The component is responsive only if you define a viewport in your html file, example:
* <meta name="viewport" content="width=device-width, initial-scale=1">
*
* @param mobileViewportMaxWidth max width threshold below which the component will consider
* to be displayed on mobile device
*/
public ChosenOptions setMobileViewportMaxWidth(int mobileViewportMaxWidth) {
this.mobileViewportMaxWidth = mobileViewportMaxWidth;
return this;
}
/**
* Set the text to use when one option is selected on a mobile multiple select.
*
* {}
can be used in the text to indicate where to put the number of option selected (in this case 1).
*
* Ex:
* options.setOneSelectedTextMultipleMobile("{} country selected");
*/
public void setOneSelectedTextMultipleMobile(String oneSelectedTextMultipleMobile) {
this.oneSelectedTextMultipleMobile = oneSelectedTextMultipleMobile;
}
/**
* Set the text to use when several options are selected on a mobile multiple select.
*
* {}
can be used in the text to indicate where to put the number of option selected.
*
* Ex:
* options.setManySelectedTextMultipleMobile("{} countries selected");
*/
public void setManySelectedTextMultipleMobile(String manySelectedTextMultipleMobile) {
this.manySelectedTextMultipleMobile = manySelectedTextMultipleMobile;
}
public String getNoResultsText() {
return noResultsText;
}
public ChosenOptions setNoResultsText(String noResultsText) {
this.noResultsText = noResultsText;
return this;
}
public String getPlaceholderText() {
return placeholderText;
}
public ChosenOptions setPlaceholderText(String placeholderText) {
this.placeholderText = placeholderText;
return this;
}
public String getPlaceholderTextMultiple() {
return placeholderTextMultiple;
}
public ChosenOptions setPlaceholderTextMultiple(String placeholderTextMultiple) {
this.placeholderTextMultiple = placeholderTextMultiple;
return this;
}
public String getPlaceholderTextSingle() {
return placeholderTextSingle;
}
public ChosenOptions setPlaceholderTextSingle(String placeholderTextSingle) {
this.placeholderTextSingle = placeholderTextSingle;
return this;
}
public Resources getResources() {
return resources;
}
public ChosenOptions setResources(Resources resources) {
this.resources = resources;
return this;
}
/**
* provide the {@code ResultFilter} instance used to filter the data.
*/
public ResultsFilter getResultFilter() {
return resultFilter;
}
public void setResultFilter(ResultsFilter resultFilter) {
this.resultFilter = resultFilter;
}
/**
* Specify if the deselection is allowed on single selects.
*/
public boolean isAllowSingleDeselect() {
return allowSingleDeselect;
}
public ChosenOptions setAllowSingleDeselect(Boolean allowSingleDeselect) {
this.allowSingleDeselect = allowSingleDeselect;
return this;
}
public boolean isHighlightSearchTerm() {
return highlightSearchTerm;
}
public void setHighlightSearchTerm(boolean highlightSearchTerm) {
this.highlightSearchTerm = highlightSearchTerm;
}
public boolean isSearchContains() {
return searchContains;
}
public ChosenOptions setSearchContains(boolean searchContains) {
this.searchContains = searchContains;
return this;
}
public boolean isSingleBackstrokeDelete() {
return singleBackstrokeDelete;
}
public ChosenOptions setSingleBackstrokeDelete(boolean singleBackstrokeDelete) {
this.singleBackstrokeDelete = singleBackstrokeDelete;
return this;
}
public boolean isMobileAnimation() {
return mobileAnimation;
}
public ChosenOptions setMobileAnimation(boolean mobileAnimation) {
this.mobileAnimation = mobileAnimation;
return this;
}
public ChosenOptions setMobileAnimationSpeed(int mobileAnimationSpeed) {
this.mobileAnimationSpeed = mobileAnimationSpeed;
return this;
}
public int getMobileAnimationSpeed() {
return this.mobileAnimationSpeed;
}
private void setDefault() {
allowSingleDeselect = false;
disableSearchThreshold = 0;
searchContains = false;
singleBackstrokeDelete = false;
maxSelectedOptions = -1;
highlightSearchTerm = true;
dropdownPosition = DropdownPosition.BELOW;
mobileViewportMaxWidth = 649;
oneSelectedTextMultipleMobile = "{} item selected";
manySelectedTextMultipleMobile = "{} items selected";
mobileAnimation = true;
mobileAnimationSpeed = 150;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy