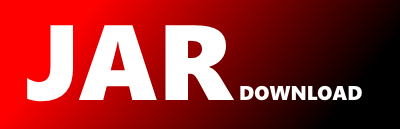
pcap.jdk7.internal.SelectableList Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2021 Pcap Project
* SPDX-License-Identifier: MIT OR Apache-2.0
*/
package pcap.jdk7.internal;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Selectable list.
*
* @param selectable type.
* @since 1.1.0
*/
class SelectableList implements Iterable {
Node head;
/**
* No selectable data.
*
* @since 1.1.0
*/
public SelectableList() {
this.head = null;
}
/**
* Add single selectable data.
*
* @param initial initial head.
* @since 1.1.0
*/
public SelectableList(T initial) {
add(initial);
}
/**
* Add selectable data.
*
* @param data selectable.
* @since 1.1.0
*/
public void add(T data) {
Node newNode = new Node();
newNode.data = data;
newNode.next = head;
this.head = newNode;
}
@Override
public Iterator iterator() {
return new SelectableListIterator(head);
}
private static final class Node {
private T data;
private Node next;
}
private static final class SelectableListIterator implements Iterator {
private Node node;
private SelectableListIterator(Node node) {
this.node = node;
}
@Override
public boolean hasNext() {
return node != null;
}
@Override
public T next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
Node previous = node;
this.node = node.next;
return previous.data;
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy