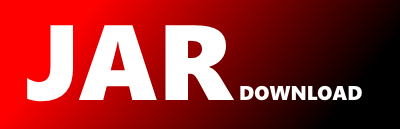
com.arm.mbed.cloud.sdk.deviceupdate.model.CampaignStatisticsEvents Maven / Gradle / Ivy
// This file was generated by the Pelion SDK foundation code generator.
// This is an autogenerated class. Do not modify its content as modifications will be lost at next code generation.
package com.arm.mbed.cloud.sdk.deviceupdate.model;
import com.arm.mbed.cloud.sdk.annotations.Internal;
import com.arm.mbed.cloud.sdk.annotations.Preamble;
import com.arm.mbed.cloud.sdk.annotations.Required;
import com.arm.mbed.cloud.sdk.common.SdkModel;
import java.util.Date;
import java.util.Objects;
/**
* Model for a campaign statistics events.
*/
@Preamble(description = "Model for a campaign statistics events.")
@SuppressWarnings({ "PMD.CyclomaticComplexity", "PMD.AvoidDuplicateLiterals" })
public class CampaignStatisticsEvents implements SdkModel {
/**
* Serialisation Id.
*/
private static final long serialVersionUID = -241276693113161L;
/**
* ID of the associated campaign.
*/
@Required
private String campaignId;
/**
* value.
*/
private final int count;
/**
* value.
*/
private final Date createdAt;
/**
* value.
*/
private final String description;
/**
* value.
*/
private final String eventType;
/**
* value.
*/
private String id;
/**
* value.
*/
private final String summaryStatus;
/**
* value.
*/
@Required
private String summaryStatusId;
/**
* Internal constructor.
*
*
* Constructor based on all fields.
*
* Note: Should not be used. Use {@link #CampaignStatisticsEvents()} instead.
*
* @param campaignId
* ID of the associated campaign.
* @param count
* value.
* @param createdAt
* value.
* @param description
* value.
* @param eventType
* value.
* @param id
* value.
* @param summaryStatus
* value.
* @param summaryStatusId
* value.
*/
@Internal
@SuppressWarnings("PMD.CyclomaticComplexity")
public CampaignStatisticsEvents(String campaignId, int count, Date createdAt, String description, String eventType,
String id, String summaryStatus, String summaryStatusId) {
super();
this.count = count;
this.createdAt = createdAt;
this.description = description;
this.eventType = eventType;
this.summaryStatus = summaryStatus;
setCampaignId(campaignId);
setId(id);
setSummaryStatusId(summaryStatusId);
}
/**
* Internal constructor.
*
*
* Constructor based on a similar object.
*
* Note: Should not be used. Use {@link #CampaignStatisticsEvents()} instead.
*
* @param campaignStatisticsEvents
* a campaign statistics events.
*/
@Internal
public CampaignStatisticsEvents(CampaignStatisticsEvents campaignStatisticsEvents) {
this(campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.campaignId,
campaignStatisticsEvents == null ? 0 : campaignStatisticsEvents.count,
campaignStatisticsEvents == null ? new Date() : campaignStatisticsEvents.createdAt,
campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.description,
campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.eventType,
campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.id,
campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.summaryStatus,
campaignStatisticsEvents == null ? (String) null : campaignStatisticsEvents.summaryStatusId);
}
/**
* Constructor.
*/
public CampaignStatisticsEvents() {
this((String) null, 0, new Date(), (String) null, (String) null, (String) null, (String) null, (String) null);
}
/**
* Constructor.
*
*
* Constructor based on object identifier.
*
*
* @param id
* value.
*/
public CampaignStatisticsEvents(String id) {
this();
setId(id);
}
/**
* Internal constructor.
*
*
* Constructor based on read-only fields.
*
* Note: Should not be used. Use {@link #CampaignStatisticsEvents()} instead.
*
* @param count
* value.
* @param createdAt
* value.
* @param description
* value.
* @param eventType
* value.
* @param summaryStatus
* value.
*/
@Internal
public CampaignStatisticsEvents(int count, Date createdAt, String description, String eventType,
String summaryStatus) {
this((String) null, count, createdAt, description, eventType, (String) null, summaryStatus, (String) null);
}
/**
* Constructor.
*
*
* Constructor based on required fields.
*
*
* @param campaignId
* ID of the associated campaign.
* @param summaryStatusId
* value.
*/
public CampaignStatisticsEvents(String campaignId, String summaryStatusId) {
this(campaignId, 0, new Date(), (String) null, (String) null, (String) null, (String) null, summaryStatusId);
}
/**
* Gets id of the associated campaign.
*
* @return campaignId
*/
public String getCampaignId() {
return campaignId;
}
/**
* Sets id of the associated campaign.
*
* @param campaignId
* ID of the associated campaign.
*/
@Required
public void setCampaignId(String campaignId) {
this.campaignId = campaignId;
}
/**
* Checks whether campaignId value is valid.
*
* @return true if the value is valid; false otherwise.
*/
@SuppressWarnings("PMD.UselessParentheses")
public boolean isCampaignIdValid() {
return campaignId != null;
}
/**
* Gets value.
*
* @return count
*/
public int getCount() {
return count;
}
/**
* Gets value.
*
* @return createdAt
*/
public Date getCreatedAt() {
return createdAt;
}
/**
* Gets value.
*
* @return description
*/
public String getDescription() {
return description;
}
/**
* Gets value.
*
* @return eventType
*/
public String getEventType() {
return eventType;
}
/**
* Gets value.
*
* @return id
*/
@Override
public String getId() {
return id;
}
/**
* Sets value.
*
* @param id
* value.
*/
@Override
public void setId(String id) {
this.id = id;
}
/**
* Sets value.
*
*
* Similar to {@link #setId(String)}
*
* @param campaignStatisticsEventsId
* value.
*/
@Internal
public void setCampaignStatisticsEventsId(String campaignStatisticsEventsId) {
setId(campaignStatisticsEventsId);
}
/**
* Gets value.
*
* @return summaryStatus
*/
public String getSummaryStatus() {
return summaryStatus;
}
/**
* Gets value.
*
* @return summaryStatusId
*/
public String getSummaryStatusId() {
return summaryStatusId;
}
/**
* Sets value.
*
* @param summaryStatusId
* value.
*/
@Required
public void setSummaryStatusId(String summaryStatusId) {
this.summaryStatusId = summaryStatusId;
}
/**
* Checks whether summaryStatusId value is valid.
*
* @return true if the value is valid; false otherwise.
*/
@SuppressWarnings("PMD.UselessParentheses")
public boolean isSummaryStatusIdValid() {
return summaryStatusId != null;
}
/**
* Returns a string representation of the object.
*
*
*
* @see java.lang.Object#toString()
* @return the string representation
*/
@Override
public String toString() {
return "CampaignStatisticsEvents [campaignId=" + campaignId + ", count=" + count + ", createdAt=" + createdAt
+ ", description=" + description + ", eventType=" + eventType + ", id=" + id + ", summaryStatus="
+ summaryStatus + ", summaryStatusId=" + summaryStatusId + "]";
}
/**
* Calculates the hash code of this instance based on field values.
*
*
*
* @see java.lang.Object#hashCode()
* @return hash code
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((campaignId == null) ? 0 : campaignId.hashCode());
result = prime * result + Objects.hashCode(Integer.valueOf(count));
result = prime * result + ((createdAt == null) ? 0 : createdAt.hashCode());
result = prime * result + ((description == null) ? 0 : description.hashCode());
result = prime * result + ((eventType == null) ? 0 : eventType.hashCode());
result = prime * result + ((id == null) ? 0 : id.hashCode());
result = prime * result + ((summaryStatus == null) ? 0 : summaryStatus.hashCode());
result = prime * result + ((summaryStatusId == null) ? 0 : summaryStatusId.hashCode());
return result;
}
/**
* Method to ensure {@link #equals(Object)} is correct.
*
*
* Note: see this article: canEqual()
*
* @param other
* another object.
* @return true if the other object is an instance of the class in which canEqual is (re)defined, false otherwise.
*/
protected boolean canEqual(Object other) {
return other instanceof CampaignStatisticsEvents;
}
/**
* Indicates whether some other object is "equal to" this one.
*
*
*
* @see java.lang.Object#equals(java.lang.Object)
* @param obj
* an object to compare with this instance.
* @return true if this object is the same as the obj argument; false otherwise.
*/
@Override
@SuppressWarnings({ "PMD.ExcessiveMethodLength", "PMD.NcssMethodCount" })
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CampaignStatisticsEvents)) {
return false;
}
final CampaignStatisticsEvents other = (CampaignStatisticsEvents) obj;
if (!other.canEqual(this)) {
return false;
}
if (campaignId == null) {
if (other.campaignId != null) {
return false;
}
} else if (!campaignId.equals(other.campaignId)) {
return false;
}
if (count != other.count) {
return false;
}
if (createdAt == null) {
if (other.createdAt != null) {
return false;
}
} else if (!createdAt.equals(other.createdAt)) {
return false;
}
if (description == null) {
if (other.description != null) {
return false;
}
} else if (!description.equals(other.description)) {
return false;
}
if (eventType == null) {
if (other.eventType != null) {
return false;
}
} else if (!eventType.equals(other.eventType)) {
return false;
}
if (id == null) {
if (other.id != null) {
return false;
}
} else if (!id.equals(other.id)) {
return false;
}
if (summaryStatus == null) {
if (other.summaryStatus != null) {
return false;
}
} else if (!summaryStatus.equals(other.summaryStatus)) {
return false;
}
if (summaryStatusId == null) {
if (other.summaryStatusId != null) {
return false;
}
} else if (!summaryStatusId.equals(other.summaryStatusId)) {
return false;
}
return true;
}
/**
* Checks whether the model is valid or not.
*
*
*
* @see SdkModel#isValid()
* @return true if the model is valid; false otherwise.
*/
@Override
public boolean isValid() {
return isCampaignIdValid() && isSummaryStatusIdValid();
}
/**
* Clones this instance.
*
*
*
* @see java.lang.Object#clone()
* @return a cloned instance
*/
@Override
public CampaignStatisticsEvents clone() {
return new CampaignStatisticsEvents(this);
}
}