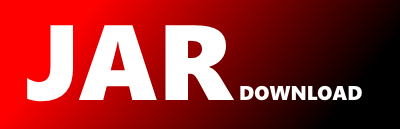
com.arm.mbed.cloud.sdk.deviceupdate.model.UpdateCampaign Maven / Gradle / Ivy
// This file was generated by the Pelion SDK foundation code generator.
// This model class was autogenerated on Fri Apr 05 11:47:42 BST 2019. Feel free to change its contents as you wish.
package com.arm.mbed.cloud.sdk.deviceupdate.model;
import java.util.Date;
import java.util.Map;
import com.arm.mbed.cloud.sdk.annotations.Internal;
import com.arm.mbed.cloud.sdk.annotations.Preamble;
import com.arm.mbed.cloud.sdk.common.SdkModel;
import com.arm.mbed.cloud.sdk.common.listing.ListOptions;
import com.arm.mbed.cloud.sdk.common.listing.filtering.FilterMarshaller;
import com.arm.mbed.cloud.sdk.common.listing.filtering.Filters;
/**
* Model for an update campaign.
*/
@Preamble(description = "Model for an update campaign.")
@SuppressWarnings("PMD.CyclomaticComplexity")
public class UpdateCampaign extends AbstractUpdateCampaign {
/**
* Serialisation Id.
*/
private static final long serialVersionUID = -116737460021164L;
/**
* Filter encoder/decoder.
*/
// TODO add some field mapping if necessary i.e Device#FILTER_MAPPING.
private static final FilterMarshaller FILTERS_MARSHALLER = new FilterMarshaller(null);
/**
* Internal constructor.
*
*
* Note: Should not be used. Use {@link #UpdateCampaign()} instead.
*
* @param deviceFilterHelper
* Helper for creating the device filter string. This helper can be used instead of setting device filter
* directly. This allows the campaign filter to be created in a way which is similar to the device
* listing filter.
* @param autostopReason
* Text description of why a campaign failed to start or why a campaign stopped.
* @param createdAt
* The time the update campaign was created.
* @param description
* An optional description of the campaign.
* @param deviceFilter
* The filter for the devices the campaign is targeting at.
* @param finished
* The campaign finish timestamp.
* @param id
* The campaign ID.
* @param name
* The campaign name.
* @param phase
* The current phase of the campaign.
* @param rootManifestId
* value.
* @param rootManifestUrl
* value.
* @param startedAt
* value.
* @param updatedAt
* The time the object was updated.
* @param when
* The scheduled start time for the campaign. The campaign will start within 1 minute when then start
* time has elapsed.
*/
@Internal
@SuppressWarnings("PMD.CyclomaticComplexity")
public UpdateCampaign(Filters deviceFilterHelper, String autostopReason, Date createdAt, String description,
String deviceFilter, Date finished, String id, String name, String phase,
String rootManifestId, String rootManifestUrl, Date startedAt, Date updatedAt, Date when) {
super(autostopReason, createdAt, description, deviceFilter, finished, id, name, phase, rootManifestId,
rootManifestUrl, startedAt, updatedAt, when);
setDeviceFiltersHelper(deviceFilterHelper);
}
/**
* Internal constructor.
*
*
* Note: Should not be used. Use {@link #UpdateCampaign()} instead.
*
* @param autostopReason
* Text description of why a campaign failed to start or why a campaign stopped.
* @param createdAt
* The time the update campaign was created.
* @param finished
* The campaign finish timestamp.
* @param phase
* The current phase of the campaign.
* @param rootManifestUrl
* value.
* @param startedAt
* value.
* @param updatedAt
* The time the object was updated.
*/
@Internal
public UpdateCampaign(String autostopReason, Date createdAt, Date finished, String phase, String rootManifestUrl,
Date startedAt, Date updatedAt) {
super(autostopReason, createdAt, finished, phase, rootManifestUrl, startedAt, updatedAt);
}
/**
* Internal constructor.
*
*
* Note: Should not be used. Use {@link #UpdateCampaign()} instead.
*
* @param updateCampaign
* an update campaign.
*/
@Internal
public UpdateCampaign(UpdateCampaign updateCampaign) {
this(updateCampaign == null ? (Filters) null : updateCampaign.getDeviceFiltersHelper(),
updateCampaign == null ? (String) null : updateCampaign.autostopReason,
updateCampaign == null ? new Date() : updateCampaign.createdAt,
updateCampaign == null ? (String) null : updateCampaign.description,
updateCampaign == null ? (String) null : updateCampaign.deviceFilter,
updateCampaign == null ? new Date() : updateCampaign.finished,
updateCampaign == null ? (String) null : updateCampaign.id,
updateCampaign == null ? (String) null : updateCampaign.name,
updateCampaign == null ? (String) null : updateCampaign.phase,
updateCampaign == null ? (String) null : updateCampaign.rootManifestId,
updateCampaign == null ? (String) null : updateCampaign.rootManifestUrl,
updateCampaign == null ? new Date() : updateCampaign.startedAt,
updateCampaign == null ? new Date() : updateCampaign.updatedAt,
updateCampaign == null ? new Date() : updateCampaign.when);
}
/**
* Constructor.
*/
public UpdateCampaign() {
this((Filters) null, (String) null, new Date(), (String) null, (String) null, new Date(), (String) null,
(String) null, (String) null, (String) null, (String) null, new Date(), new Date(), new Date());
}
/**
* Constructor.
*
* @param id
* The campaign ID.
*/
public UpdateCampaign(String id) {
this();
setId(id);
}
/**
* Gets the device filter ({@link UpdateCampaign#getDeviceFilter()}) defined as a generic {@link Filters}.
*
*
* This helper can be used instead of getting device filter directly ({@link UpdateCampaign#getDeviceFilter()}) as a
* string. This allows the campaign filter to be seen and defined in a way which is similar to the device listing
* filter.
*
* @return deviceFilterHelper
*/
public Filters getDeviceFiltersHelper() {
return FILTERS_MARSHALLER.decode(getDeviceFilter());
}
/**
* Sets the device filter ({@link UpdateCampaign#setDeviceFilter(String)}) using a generic {@link Filters} object
* defined within {@link ListOptions}.
*
* This helper can be used instead of setting device filter directly. This allows the campaign filter to be created
* in a way which is similar to the device listing filter. It is hence possible to define the filter using the
* DeviceListOptions helpers and then pass it to a campaign using {@link ListOptions#getFilter()}.
*
* @param deviceFilter
* Helper for creating the device filter string. This helper can be used instead of setting device filter
* directly. This allows the campaign filter to be created in a way which is similar to the device
* listing filter.
*/
public void setDeviceFiltersHelper(Filters deviceFilter) {
setDeviceFilter(deviceFilter == null ? getDeviceFilter() == null ? (String) null : getDeviceFilter()
: FILTERS_MARSHALLER.encode(deviceFilter));
}
/**
* Sets the device filter ({@link UpdateCampaign#setDeviceFilter(String)}).
*
* Prefer using {@link #setDeviceFiltersHelper(Filters)} or {@link #setDeviceFilterFromJson(String)} to set filters.
*
* @param filter
* filters expressed as a Json hashtable (key,value)
*/
@Internal
public void setDeviceFilterHelper(Map filter) {
setDeviceFiltersHelper(FilterMarshaller.fromJsonObject(filter));
}
/**
* Gets the filter as a "Json object".
*
* @return the filters as a Json object
*/
@Internal
public Map getDeviceFilterHelper() {
return FilterMarshaller.toJsonObject(getDeviceFiltersHelper());
}
/**
* Sets the device filter from a Json string.
*
* @see FilterMarshaller#fromJson(String)
*
* @param jsonString
* Json string defining the device filter to set
*/
public void setDeviceFilterFromJson(String jsonString) {
setDeviceFiltersHelper(FilterMarshaller.fromJson(jsonString));
}
/**
* Gets the device filter as Json String.
*
* @see FilterMarshaller#toJson(Filters)
*
* @return the filters as a Json string
*/
public String getDeviceFilterAsJson() {
return FilterMarshaller.toJson(getDeviceFiltersHelper());
}
/**
* Returns a string representation of the object.
*
*
*
* @see java.lang.Object#toString()
* @return the string representation
*/
@Override
public String toString() {
return "UpdateCampaign [autostopReason=" + autostopReason + ", createdAt=" + createdAt + ", description="
+ description + ", deviceFilter=" + getDeviceFilterAsJson() + ", finished=" + finished + ", id=" + id
+ ", name=" + name + ", phase=" + phase + ", rootManifestId=" + rootManifestId + ", rootManifestUrl="
+ rootManifestUrl + ", startedAt=" + startedAt + ", updatedAt=" + updatedAt + ", when=" + when + "]";
}
/**
* Calculates the hash code of this instance based on field values.
*
*
*
* @see java.lang.Object#hashCode()
* @return hash code
*/
@SuppressWarnings("PMD.UselessOverridingMethod")
@Override
public int hashCode() {
return super.hashCode();
}
/**
* Indicates whether some other object is "equal to" this one.
*
*
*
* @see java.lang.Object#equals(java.lang.Object)
* @param obj
* an object to compare with this instance.
* @return true if this object is the same as the obj argument; false otherwise.
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!super.equals(obj)) {
return false;
}
if (!(obj instanceof UpdateCampaign)) {
return false;
}
final UpdateCampaign other = (UpdateCampaign) obj;
if (!other.canEqual(this)) {
return false;
}
return true;
}
/**
* Checks whether the model is valid or not.
*
*
*
* @see SdkModel#isValid()
* @return true if the model is valid; false otherwise.
*/
@SuppressWarnings("PMD.UselessOverridingMethod")
@Override
public boolean isValid() {
return super.isValid();
}
/**
* Clones this instance.
*
*
*
* @see java.lang.Object#clone()
* @return a cloned instance
*/
@Override
public UpdateCampaign clone() {
return new UpdateCampaign(this);
}
/**
* Method to ensure {@link #equals(Object)} is correct.
*
*
* Note: see this article: canEqual()
*
* @param other
* another object.
* @return true if the other object is an instance of the class in which canEqual is (re)defined, false otherwise.
*/
@Override
protected boolean canEqual(Object other) {
return other instanceof UpdateCampaign;
}
}