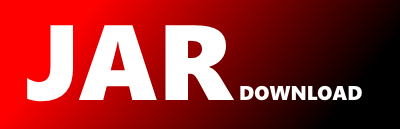
com.arm.mbed.cloud.sdk.connect.adapters.PresubscriptionAdapter Maven / Gradle / Ivy
Go to download
The Mbed Cloud SDK provides a simplified interface to the Mbed Cloud APIs by exposing functionality using conventions and paradigms familiar to Java developers.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy