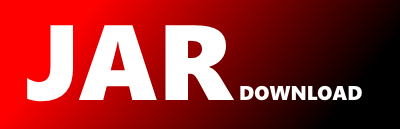
com.arpnetworking.configuration.Configuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metrics-cluster-aggregator Show documentation
Show all versions of metrics-cluster-aggregator Show documentation
(Re)Aggregates host level statistics across clusters and writes both host and cluster statistics to various destinations.
/*
* Copyright 2014 Groupon.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.arpnetworking.configuration;
import java.lang.reflect.Type;
import java.util.NoSuchElementException;
import java.util.Optional;
/**
* Interface for classes which provide configuration.
*
* @author Ville Koskela (ville dot koskela at inscopemetrics dot com)
*/
public interface Configuration {
/**
* Retrieve the value of a particular property by its name.
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
*/
Optional getProperty(String name);
/**
* Retrieve the value of a particular property by its name and if it does
* not exist return the specified default.
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
*/
String getProperty(String name, String defaultValue);
/**
* Retrieve the value of a particular property by its name and if it does
* not exist throw a RuntimeException
.
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
*/
String getRequiredProperty(String name) throws NoSuchElementException;
/**
* Boolean
specific accessor. Anything other than "true",
* ignoring case, is treated as false
.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
*/
Optional getPropertyAsBoolean(String name);
/**
* Boolean
specific accessor. Anything other than "true",
* ignoring case, is treated as false
.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
*/
boolean getPropertyAsBoolean(String name, boolean defaultValue);
/**
* Boolean
specific accessor. Anything other than "true",
* ignoring case, is treated as false
.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
*/
boolean getRequiredPropertyAsBoolean(String name) throws NoSuchElementException;
/**
* Integer
specific accessor.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to an
* Integer
.
*/
Optional getPropertyAsInteger(String name) throws NumberFormatException;
/**
* Integer
specific accessor.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to an
* Integer
.
*/
int getPropertyAsInteger(String name, int defaultValue) throws NumberFormatException;
/**
* Integer
specific accessor.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws NumberFormatException if the value cannot be converted to an
* Integer
.
*/
int getRequiredPropertyAsInteger(String name) throws NoSuchElementException, NumberFormatException;
/**
* Long
specific accessor.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Long
.
*/
Optional getPropertyAsLong(String name) throws NumberFormatException;
/**
* Long
specific accessor.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Long
.
*/
long getPropertyAsLong(String name, long defaultValue) throws NumberFormatException;
/**
* Long
specific accessor.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws NumberFormatException if the value cannot be converted to a
* Long
.
*/
long getRequiredPropertyAsLong(String name) throws NoSuchElementException, NumberFormatException;
/**
* Double
specific accessor.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Double
.
*/
Optional getPropertyAsDouble(String name) throws NumberFormatException;
/**
* Double
specific accessor.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Double
.
*/
double getPropertyAsDouble(String name, double defaultValue) throws NumberFormatException;
/**
* Double
specific accessor.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws NumberFormatException if the value cannot be converted to a
* Double
.
*/
double getRequiredPropertyAsDouble(String name) throws NoSuchElementException, NumberFormatException;
/**
* Float
specific accessor.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Float
.
*/
Optional getPropertyAsFloat(String name) throws NumberFormatException;
/**
* Float
specific accessor.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Float
.
*/
float getPropertyAsFloat(String name, float defaultValue) throws NumberFormatException;
/**
* Float
specific accessor.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws NumberFormatException if the value cannot be converted to a
* Float
.
*/
float getRequiredPropertyAsFloat(String name) throws NoSuchElementException, NumberFormatException;
/**
* Short
specific accessor.
*
* @see Configuration#getProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Short
.
*/
Optional getPropertyAsShort(String name) throws NumberFormatException;
/**
* Short
specific accessor.
*
* @see Configuration#getProperty(String, String)
*
* @param name The name of the property value to retrieve.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or defaultValue
if
* the property name has not been defined.
* @throws NumberFormatException if the value cannot be converted to a
* Short
.
*/
short getPropertyAsShort(String name, short defaultValue) throws NumberFormatException;
/**
* Short
specific accessor.
*
* @see Configuration#getRequiredProperty(String)
*
* @param name The name of the property value to retrieve.
* @return Returns the property value.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws NumberFormatException if the value cannot be converted to a
* Short
.
*/
short getRequiredPropertyAsShort(String name) throws NoSuchElementException, NumberFormatException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param clazz The type of the object to instantiate.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
Optional getPropertyAs(String name, Class extends T> clazz) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param clazz The type of the object to instantiate.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getPropertyAs(String name, Class extends T> clazz, T defaultValue) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param clazz The type of the object to instantiate.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getRequiredPropertyAs(String name, Class extends T> clazz) throws NoSuchElementException, IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param clazz The type of the object to instantiate.
* @return Returns the entire configuration as an instance of T
.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
Optional getAs(Class extends T> clazz) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @see Configuration#getProperty(String)
*
* @param The type to return.
* @param clazz The type of the object to instantiate.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the entire configuration as an instance of T
.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getAs(Class extends T> clazz, T defaultValue) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param clazz The type of the object to instantiate.
* @return Returns the entire configuration as an instance of T
.
* @throws NoSuchElementException throws the RuntimeException
* if the configuration is not available.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getRequiredAs(Class extends T> clazz) throws NoSuchElementException, IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param type The type of the object to instantiate.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
Optional getPropertyAs(String name, Type type) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param type The type of the object to instantiate.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getPropertyAs(String name, Type type, T defaultValue) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param name The name of the property value to retrieve.
* @param type The type of the object to instantiate.
* @return Returns the property value or Optional.absent
if
* the property name has not been defined.
* @throws NoSuchElementException throws the RuntimeException
* if the specified property name is not defined.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getRequiredPropertyAs(String name, Type type) throws NoSuchElementException, IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param type The type of the object to instantiate.
* @return Returns the entire configuration as an instance of T
.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
Optional getAs(Type type) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @see Configuration#getProperty(String)
*
* @param The type to return.
* @param type The type of the object to instantiate.
* @param defaultValue The value to return if the specified property name
* does not exist in the configuration.
* @return Returns the entire configuration as an instance of T
.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getAs(Type type, T defaultValue) throws IllegalArgumentException;
/**
* Generic object accessor.
*
* @param The type to return.
* @param type The type of the object to instantiate.
* @return Returns the entire configuration as an instance of T
.
* @throws NoSuchElementException throws the RuntimeException
* if the configuration is not available.
* @throws IllegalArgumentException if the value cannot be converted to an
* instance of T
.
*/
T getRequiredAs(Type type) throws NoSuchElementException, IllegalArgumentException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy