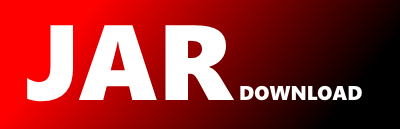
com.arpnetworking.configuration.jackson.JsonNodeUriSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metrics-cluster-aggregator Show documentation
Show all versions of metrics-cluster-aggregator Show documentation
(Re)Aggregates host level statistics across clusters and writes both host and cluster statistics to various destinations.
/*
* Copyright 2015 Groupon.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.arpnetworking.configuration.jackson;
import com.arpnetworking.logback.annotations.LogValue;
import com.arpnetworking.steno.LogValueMapFactory;
import com.fasterxml.jackson.databind.JsonNode;
import net.sf.oval.constraint.NotNull;
import org.apache.http.Header;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.HttpClientConnectionManager;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
/**
* JsonNode
based configuration sourced from a file.
*
* @author Ville Koskela (ville dot koskela at inscopemetrics dot com)
*/
public final class JsonNodeUriSource extends BaseJsonNodeSource {
@Override
public Optional getValue(final String... keys) {
return getValue(getJsonNode(), keys);
}
@LogValue
@Override
public Object toLogValue() {
return LogValueMapFactory.builder(this)
.put("super", super.toLogValue())
.put("uri", _uri)
.put("jsonNode", _jsonNode)
.build();
}
@Override
public String toString() {
return toLogValue().toString();
}
/* package private */ Optional getJsonNode() {
return _jsonNode;
}
private JsonNodeUriSource(final Builder builder) {
super(builder);
_uri = builder._uri;
final List headers = new ArrayList<>(builder._headers);
JsonNode jsonNode = null;
HttpGet request = null;
try {
request = new HttpGet(_uri);
if (!headers.isEmpty()) {
//NOTE: This overrides all headers for this request. If other headers are to be added, use addHeader
//method after this or change this to addHeaders
request.setHeaders(headers.toArray(new Header[headers.size()]));
}
final HttpResponse response = CLIENT.execute(request);
jsonNode = _objectMapper.readTree(response.getEntity().getContent());
} catch (final IOException e) {
throw new RuntimeException(e);
} finally {
if (request != null) {
request.releaseConnection();
}
}
_jsonNode = Optional.ofNullable(jsonNode);
}
private final URI _uri;
private final Optional _jsonNode;
private static final int CONNECTION_TIMEOUT_IN_MILLISECONDS = 3000;
private static final HttpClientConnectionManager CONNECTION_MANAGER = new PoolingHttpClientConnectionManager();
private static final HttpClient CLIENT = HttpClientBuilder.create()
.setConnectionManager(CONNECTION_MANAGER)
.setDefaultRequestConfig(
RequestConfig.copy(RequestConfig.DEFAULT)
.setConnectTimeout(CONNECTION_TIMEOUT_IN_MILLISECONDS)
.build())
.build();
/**
* Builder for JsonNodeUriSource
.
*/
public static final class Builder extends BaseJsonNodeSource.Builder {
/**
* Public constructor.
*/
public Builder() {
super(JsonNodeUriSource::new);
}
/**
* Set the source URI
.
*
* @param value The source URI
.
* @return This Builder
instance.
*/
public Builder setUri(final URI value) {
_uri = value;
return this;
}
/**
* Add a request header for the source.
*
* @param value The Header
value.
* @return This Builder
instance.
*/
public Builder addHeader(final Header value) {
_headers.add(value);
return this;
}
/**
* Add a list of request headers for the source.
*
* @param values The List
of Header
values.
* @return This Builder
instance.
*/
public Builder addHeaders(final List values) {
_headers.addAll(values);
return this;
}
/**
* Overrides the existing headers with a List
of Header
.
*
* @param values A List
of HTTP headers.
* @return This Builder
instance.
*/
public Builder setHeaders(final List values) {
_headers = new ArrayList<>(values);
return this;
}
@Override
protected Builder self() {
return this;
}
@NotNull
private URI _uri;
@NotNull
private List _headers = new ArrayList<>();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy