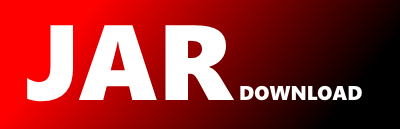
models.ebean.Expression Maven / Gradle / Ivy
/**
* Copyright 2015 Groupon.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package models.ebean;
import com.avaje.ebean.Model;
import com.avaje.ebean.annotation.CreatedTimestamp;
import com.avaje.ebean.annotation.UpdatedTimestamp;
import java.sql.Timestamp;
import java.util.UUID;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Version;
/**
* Data model for expressions.
*
* @author Deepika Misra (deepika at groupon dot com)
*/
// CHECKSTYLE.OFF: MemberNameCheck
@Entity
@Table(name = "expressions", schema = "portal")
public class Expression extends Model {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private Long id;
@Version
@Column(name = "version")
private Long version;
@CreatedTimestamp
@Column(name = "created_at")
private Timestamp createdAt;
@UpdatedTimestamp
@Column(name = "updated_at")
private Timestamp updatedAt;
@Column(name = "uuid")
private UUID uuid;
@Column(name = "cluster")
private String cluster;
@Column(name = "service")
private String service;
@Column(name = "metric")
private String metric;
@Column(name = "script")
private String script;
public Long getId() {
return id;
}
public void setId(final Long value) {
id = value;
}
public Long getVersion() {
return version;
}
public void setVersion(final Long value) {
version = value;
}
public Timestamp getCreatedAt() {
return createdAt;
}
public void setCreatedAt(final Timestamp value) {
createdAt = value;
}
public Timestamp getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(final Timestamp value) {
updatedAt = value;
}
public UUID getUuid() {
return uuid;
}
public void setUuid(final UUID value) {
uuid = value;
}
public String getCluster() {
return cluster;
}
public void setCluster(final String value) {
cluster = value;
}
public String getService() {
return service;
}
public void setService(final String value) {
service = value;
}
public String getMetric() {
return metric;
}
public void setMetric(final String value) {
metric = value;
}
public String getScript() {
return script;
}
public void setScript(final String value) {
script = value;
}
}
// CHECKSTYLE.ON: MemberNameCheck
© 2015 - 2025 Weber Informatics LLC | Privacy Policy