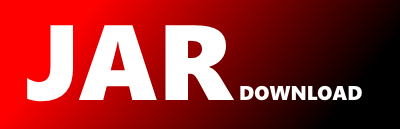
ars.database.repository.EmptyQuery Maven / Gradle / Ivy
package ars.database.repository;
import java.util.Map;
import java.util.List;
import java.util.Iterator;
import java.util.Collections;
import ars.util.Conditions.Logic;
import ars.database.repository.Query;
/**
* 数据查询集合空实现
*
* @author yongqiangwu
*
* @param
* 数据模型
*/
public final class EmptyQuery implements Query {
private static EmptyQuery> instance;
private EmptyQuery() {
}
/**
* 获取数据查询集合实例
*
* @param
* 数据类型
* @return 数据查询集合实例
*/
@SuppressWarnings("unchecked")
public static EmptyQuery instance() {
if (instance == null) {
synchronized (EmptyQuery.class) {
if (instance == null) {
instance = new EmptyQuery();
}
}
}
return (EmptyQuery) instance;
}
@Override
public Class getModel() {
return null;
}
@Override
public Iterator iterator() {
return this.list().iterator();
}
@Override
public Query empty(String... properties) {
return this;
}
@Override
public Query nonempty(String... properties) {
return this;
}
@Override
public Query eq(String property, Object value) {
return this;
}
@Override
public Query ne(String property, Object value) {
return this;
}
@Override
public Query gt(String property, Object value) {
return this;
}
@Override
public Query ge(String property, Object value) {
return this;
}
@Override
public Query lt(String property, Object value) {
return this;
}
@Override
public Query le(String property, Object value) {
return this;
}
@Override
public Query between(String property, Object low, Object high) {
return this;
}
@Override
public Query start(String property, String... values) {
return this;
}
@Override
public Query nstart(String property, String... values) {
return this;
}
@Override
public Query end(String property, String... values) {
return this;
}
@Override
public Query nend(String property, String... values) {
return this;
}
@Override
public Query like(String property, String... values) {
return this;
}
@Override
public Query nlike(String property, String... values) {
return this;
}
@Override
public Query in(String property, Object[] values) {
return this;
}
@Override
public Query or(String property, Object[] values) {
return this;
}
@Override
public Query not(String property, Object[] values) {
return this;
}
@Override
public Query custom(String property, Object value) {
return this;
}
@Override
public Query custom(Map parameters) {
return this;
}
@Override
public Query condition(Logic logic) {
return this;
}
@Override
public Query condition(String property, Object value) {
return this;
}
@Override
public Query condition(Map parameters) {
return this;
}
@Override
public Query eqProperty(String property, String other) {
return this;
}
@Override
public Query neProperty(String property, String other) {
return this;
}
@Override
public Query ltProperty(String property, String other) {
return this;
}
@Override
public Query leProperty(String property, String other) {
return this;
}
@Override
public Query gtProperty(String property, String other) {
return this;
}
@Override
public Query geProperty(String property, String other) {
return this;
}
@Override
public Query asc(String... properties) {
return this;
}
@Override
public Query desc(String... properties) {
return this;
}
@Override
public Query paging(int page, int size) {
return this;
}
@Override
public Query min(String... properties) {
return this;
}
@Override
public Query max(String... properties) {
return this;
}
@Override
public Query avg(String... properties) {
return this;
}
@Override
public Query sum(String... properties) {
return this;
}
@Override
public Query number(String... properties) {
return this;
}
@Override
public Query group(String... properties) {
return this;
}
@Override
public Query property(String... properties) {
return this;
}
@Override
public int count() {
return 0;
}
@Override
public T single() {
return null;
}
@Override
public List list() {
return Collections.emptyList();
}
@Override
public List> stats() {
return Collections.emptyList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy