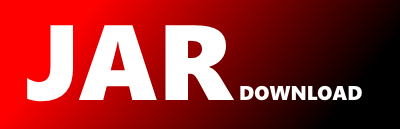
ars.database.repository.WrapQuery Maven / Gradle / Ivy
package ars.database.repository;
import java.util.Map;
import java.util.List;
import java.util.Iterator;
import ars.util.Conditions.Logic;
import ars.database.repository.Query;
/**
* 数据包装查询实现
*
* @author yongqiangwu
*
* @param
* 数据类型
*/
public class WrapQuery implements Query {
private Query query; // 查询对象
public WrapQuery(Query query) {
if (query == null) {
throw new IllegalArgumentException("Illegal query:" + query);
}
this.query = query;
}
public Query getQuery() {
return query;
}
@Override
public Iterator iterator() {
return this.query.iterator();
}
@Override
public Class getModel() {
return this.query.getModel();
}
@Override
public Query empty(String... properties) {
return this.query.empty(properties);
}
@Override
public Query nonempty(String... properties) {
return this.query.nonempty(properties);
}
@Override
public Query eq(String property, Object value) {
return this.query.eq(property, value);
}
@Override
public Query ne(String property, Object value) {
return this.query.ne(property, value);
}
@Override
public Query gt(String property, Object value) {
return this.query.gt(property, value);
}
@Override
public Query ge(String property, Object value) {
return this.query.ge(property, value);
}
@Override
public Query lt(String property, Object value) {
return this.query.lt(property, value);
}
@Override
public Query le(String property, Object value) {
return this.query.le(property, value);
}
@Override
public Query between(String property, Object low, Object high) {
return this.query.between(property, low, high);
}
@Override
public Query start(String property, String... values) {
return this.query.start(property, values);
}
@Override
public Query nstart(String property, String... values) {
return this.query.nstart(property, values);
}
@Override
public Query end(String property, String... values) {
return this.query.end(property, values);
}
@Override
public Query nend(String property, String... values) {
return this.query.nend(property, values);
}
@Override
public Query like(String property, String... values) {
return this.query.like(property, values);
}
@Override
public Query nlike(String property, String... values) {
return this.query.nlike(property, values);
}
@Override
public Query in(String property, Object[] values) {
return this.query.in(property, values);
}
@Override
public Query or(String property, Object[] values) {
return this.query.or(property, values);
}
@Override
public Query not(String property, Object[] values) {
return this.query.not(property, values);
}
@Override
public Query custom(String property, Object value) {
return this.query.custom(property, value);
}
@Override
public Query custom(Map parameters) {
return this.query.custom(parameters);
}
@Override
public Query condition(Logic logic) {
return this.query.condition(logic);
}
@Override
public Query condition(String property, Object value) {
return this.query.condition(property, value);
}
@Override
public Query condition(Map parameters) {
return this.query.condition(parameters);
}
@Override
public Query eqProperty(String property, String other) {
return this.query.eqProperty(property, other);
}
@Override
public Query neProperty(String property, String other) {
return this.query.neProperty(property, other);
}
@Override
public Query ltProperty(String property, String other) {
return this.query.ltProperty(property, other);
}
@Override
public Query leProperty(String property, String other) {
return this.query.leProperty(property, other);
}
@Override
public Query gtProperty(String property, String other) {
return this.query.gtProperty(property, other);
}
@Override
public Query geProperty(String property, String other) {
return this.query.geProperty(property, other);
}
@Override
public Query asc(String... properties) {
return this.query.asc(properties);
}
@Override
public Query desc(String... properties) {
return this.query.desc(properties);
}
@Override
public Query paging(int page, int size) {
return this.query.paging(page, size);
}
@Override
public Query min(String... properties) {
return this.query.min(properties);
}
@Override
public Query max(String... properties) {
return this.query.max(properties);
}
@Override
public Query avg(String... properties) {
return this.query.avg(properties);
}
@Override
public Query sum(String... properties) {
return this.query.sum(properties);
}
@Override
public Query number(String... properties) {
return this.query.number(properties);
}
@Override
public Query group(String... properties) {
return this.query.group(properties);
}
@Override
public Query property(String... properties) {
return this.query.property(properties);
}
@Override
public int count() {
return this.query.count();
}
@Override
public T single() {
return this.query.single();
}
@Override
public List list() {
return this.query.list();
}
@Override
public List> stats() {
return this.query.stats();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy