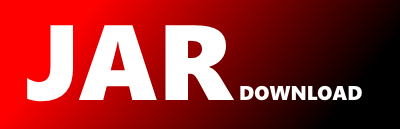
com.artipie.http.rq.multipart.Completion Maven / Gradle / Ivy
/*
* The MIT License (MIT) Copyright (c) 2020-2023 artipie.com
* https://github.com/artipie/artipie/blob/master/LICENSE.txt
*/
package com.artipie.http.rq.multipart;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Subscription;
/**
* Multi-downstream completion handler.
* @param Type of downstream subscriber
* @since 1.0
*/
final class Completion {
/**
* Fake implementation for tests.
* @since 1.0
*/
static final Completion> FAKE = new Completion<>(
new Subscriber
© 2015 - 2025 Weber Informatics LLC | Privacy Policy