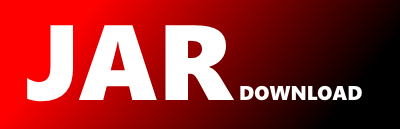
com.artipie.composer.http.PackageMetadataSlice Maven / Gradle / Ivy
/*
* The MIT License (MIT) Copyright (c) 2020-2023 artipie.com
* https://github.com/artipie/artipie/blob/master/LICENSE.txt
*/
package com.artipie.composer.http;
import com.artipie.asto.Content;
import com.artipie.composer.Name;
import com.artipie.composer.Packages;
import com.artipie.composer.Repository;
import com.artipie.http.Headers;
import com.artipie.http.Response;
import com.artipie.http.Slice;
import com.artipie.http.async.AsyncResponse;
import com.artipie.http.rq.RequestLine;
import com.artipie.http.rs.RsWithBody;
import com.artipie.http.rs.StandardRs;
import java.util.Optional;
import java.util.concurrent.CompletionStage;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Slice that serves package metadata.
*
* @since 0.3
*/
public final class PackageMetadataSlice implements Slice {
/**
* RegEx pattern for package metadata path.
* According to docs.
*/
public static final Pattern PACKAGE = Pattern.compile(
"/p2?/(?[^/]+)/(?[^/]+)\\.json$"
);
/**
* RegEx pattern for all packages metadata path.
*/
public static final Pattern ALL_PACKAGES = Pattern.compile("^/packages.json$");
/**
* Repository.
*/
private final Repository repository;
/**
* Ctor.
*
* @param repository Repository.
*/
PackageMetadataSlice(final Repository repository) {
this.repository = repository;
}
@Override
public Response response(
final RequestLine line,
final Headers headers,
final Content body
) {
return new AsyncResponse(
this.packages(line.uri().getPath())
.thenApply(
opt -> opt.map(
packages -> new AsyncResponse(packages.content()
.thenApply(RsWithBody::new)
)
).orElse(StandardRs.NOT_FOUND)
)
);
}
/**
* Builds key to storage value from path.
*
* @param path Resource path.
* @return Key to storage value.
*/
private CompletionStage> packages(final String path) {
final CompletionStage> result;
final Matcher matcher = PACKAGE.matcher(path);
if (matcher.find()) {
result = this.repository.packages(
new Name(
String.format("%s/%s", matcher.group("vendor"), matcher.group("package"))
)
);
} else if (ALL_PACKAGES.matcher(path).matches()) {
result = this.repository.packages();
} else {
throw new IllegalStateException(String.format("Unexpected path: %s", path));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy