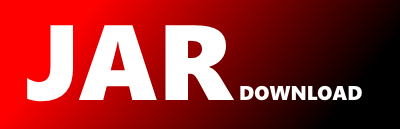
com.askfast.askfastapi.AskFastRestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of askfast-api-java Show documentation
Show all versions of askfast-api-java Show documentation
AskFast Library to use the AskFast system
package com.askfast.askfastapi;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.oltu.oauth2.client.OAuthClient;
import org.apache.oltu.oauth2.client.URLConnectionClient;
import org.apache.oltu.oauth2.client.request.OAuthClientRequest;
import org.apache.oltu.oauth2.client.response.OAuthJSONAccessTokenResponse;
import org.apache.oltu.oauth2.common.message.types.GrantType;
import com.askfast.askfastapi.model.ModifyCall;
import com.askfast.askfastapi.model.Question;
import com.askfast.model.Adapter;
import com.askfast.model.AdapterType;
import com.askfast.model.DDRRecord;
import com.askfast.model.Dialog;
import com.askfast.model.DialogRequest;
import com.askfast.model.Recording;
import com.askfast.model.RestResponse;
import com.askfast.model.Result;
import com.askfast.util.AskFastRestService;
import com.askfast.util.JSONUtil;
import com.askfast.util.JacksonConverter;
import com.squareup.okhttp.OkHttpClient;
import retrofit.RequestInterceptor;
import retrofit.RestAdapter;
import retrofit.RetrofitError;
import retrofit.client.OkClient;
/**
* A client that gives access to the Ask Fast REST API. An accountId and
* accessToken are required to access the REST API. If you do not pass an
* accessToken, make sure to pass a valid refreshToken, as the class tries to
* retrieve a fresh accessToken at the key server.
* This REST client uses
* Retrofit. Make sure to wrap
* every call with a try/catch, as an exception could be thrown when the request
* fails.
*/
public class AskFastRestClient {
public static final String DEFAULT_ENDPOINT = "https://api.ask-fast.com";
public static final String KEYSERVER_PATH = "/keyserver/token";
private String accountId = null;
private String refreshToken = null;
private String accessToken = null;
private String endpoint = null;
/**
* Creates an AskFastRestClient instance. The accessToken will be retrieved
* from the key server using your accountId and refreshToken.
*
* @param accountId
* Your accountId
* @param refreshToken
* Your refreshToken
*/
public AskFastRestClient(final String accountId, final String refreshToken) {
this(accountId, refreshToken, null);
}
/**
* Creates an AskFastRestClient instance. Be sure the accessToken is valid,
* otherwise any request will fail and most likely throw an exception. The
* accessToken is not automatically refreshed.
*
* @param accountId
* Your accountId
* @param refreshToken
* Your refreshToken
* @param accessToken
* The access token
*/
public AskFastRestClient(final String accountId, final String refreshToken, final String accessToken) {
this(accountId, refreshToken, accessToken, null);
}
/**
* Creates an AskFastRestClient instance. Be sure the accessToken is valid,
* otherwise any request will fail and most likely throw an exception. The
* accessToken is not automatically refreshed.
*
* @param accountId
* Your accountId
* @param refreshToken
* Your refreshToken
* @param accessToken
* The access token
* @param endpoint
* The endpoint, the url of API endpoint you wish to use. (The
* default is set to: https://api.ask-fast.com)
*/
public AskFastRestClient(final String accountId, final String refreshToken, final String accessToken,
final String endpoint) {
this.accountId = accountId;
this.refreshToken = refreshToken;
this.accessToken = accessToken;
this.endpoint = endpoint;
if (endpoint == null) {
this.endpoint = DEFAULT_ENDPOINT;
}
}
/**
* Initiate a phone call from a random call adapter.
*
* @param toAddress
* The address which will be called
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startPhoneDialog(String toAddress, String url) {
return this.startDialog(toAddress, AdapterType.CALL, null, null, url);
}
/**
* Initiate an SMS request from a random SMS adapter.
*
* @param toAddress
* The mobile number to which an SMS is to be sent
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startSMSDialog(String toAddress, String senderName, String url) {
return this.startDialog(toAddress, AdapterType.SMS, senderName, null, url);
}
/**
* Initiate an Email request from a random Email adapter.
*
* @param toAddress
* An email address to which an email is to be sent
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters. * @param
* subject Only valid for an email adapter. The subject of the
* message to be sent
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startEmailDialog(String toAddress, String senderName, String subject, String url) {
return this.startDialog(toAddress, AdapterType.EMAIL, senderName, subject, url);
}
/**
* Initiates a dialog request from a given adapterId.
*
* @param toAddress
* The address for which an outbound dialog request is requested
* to be initiated.
* @param adapterId
* Specific adapterId (channel or communication mode) that is
* either owned by you, or that you are a shared user of.
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startDialog(String toAddress, String adapterId, String senderName, String subject, String url) {
AskFastRestService service = getRestService();
return service.startDialog(new DialogRequest(toAddress, null, adapterId, senderName, subject, url));
}
/**
* Initiates a dialog request from a given adapterId. This will pick up the
* first random adapter of the given type, in case of multiple adapters of
* the same type.
*
* @param toAddress
* The address for which an outbound dialog request is requested
* to be initiated.
* @param adapterType
* The type of the communication for which a dialog is initiated.
* E.g. Call, SMS, Email etc
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* The url used to load the dialog. This can also be a dialogId
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startDialog(String toAddress, AdapterType adapterType, String senderName, String subject,
String url) {
AskFastRestService service = getRestService();
return service.startDialog(new DialogRequest(toAddress, adapterType, null, senderName, subject, url));
}
/**
* Initiates a broadcast dialog request from a given adapterId.
*
* @param addressMap
* The key value pairs of {toAddress, recipientName}
* @param addressCcMap
* The key value pairs of {ccAddress, recipientName}. Used in
* case of an email adapter only
* @param addressBccMap
* The key value pairs of {bccAddress, recipientName}. Used in
* case of an email adapter only
* @param adapterType
* The type of communication opted for this outbound dialog. The
* first adapter of the given type is chosen if there are
* multiple ones exist. Usually either one of adapterType or
* adapterID.
* @param adapterID
* The id identifying a particular mode of communication. These
* values can be retried from
* ASK-Fast Portal in
* the adapters section.
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
* @return Result based on a the request. If its a error, this might throw a
* RetrofitError, if it has a {@link RetrofitError#getBody()} try to
* deserialize it to {@link Result} and parse the reason for the
* failure. A login error would however not be caught by this
* Exception type.
*/
public Result startDialog(Map addressMap, Map addressCcMap,
Map addressBccMap, AdapterType adapterType, String adapterID, String senderName, String subject,
String url) {
AskFastRestService service = getRestService();
return service.startDialog(new DialogRequest(addressMap, addressCcMap, addressBccMap, adapterType, adapterID,
senderName, subject, url));
}
/**
* Returns a set of {@link Adapter Adapters}, optionally narrowed down by a
* {@code type}.
*
* @param type
* Optional. Possible values: {@code sms}, {@code call},
* {@code email} or {@code ussd}
*
* @return A set of Adapters, optionally narrowed down by a {@code type}.
*/
public Set getAdapters(String type) {
AskFastRestService service = getRestService();
return asOrderedSet( service.getAdapters(type,true) );
}
/**
* Returns adapters owned by your account and shared with
* your account.
* @param type
* @return
*/
public Set getAllAdapters(String type) {
AskFastRestService service = getRestService();
return asOrderedSet( service.getAdapters(type,false) );
}
protected static Set asOrderedSet( List src ) {
Set ret = new java.util.LinkedHashSet<>();
for(int i=0 ; i< src.size() ; i++) {
ret.add( src.get(i) );
}
return ret;
}
/**
* Returns the corresponding adapter by id
*
* @param adapterId
* The adapterId to be fetched
* @return Return the adapter
*/
public Adapter getAdapter(String adapterId) {
return getRestService().getAdapter(adapterId);
}
/**
* Updates the adapter with the given {@code adapterId}.
*
* @param adapterId
* The id of the adapter
* @param adapter
* The adapter
*
* @return The updated adapter
*/
public Adapter updateAdapter(String adapterId, Adapter adapter) {
AskFastRestService service = getRestService();
return service.updateAdapter(adapterId, adapter);
}
/**
* Buy an adapter.
*
* TODO: specify error behaviour: what happens if you can't buy the adapter?
* what if you're out of credits?
*
* @param adapterId
* The id of the adapter
*/
public void buyAdapter(String adapterId) {
AskFastRestService service = getRestService();
service.buyAdapter(adapterId);
}
/**
* Remove an adapter from the logged in account.
*
* // TODO: describe more specifically per adapter type
*
* @param adapterId
* The The id of the adapter
*/
public void removeAdapter(String adapterId) {
AskFastRestService service = getRestService();
service.removeAdapter(adapterId);
}
/**
* Gets all the free adapters
*
* @param adapterType
* The type of the adapter
* @param address
* The address of adapter looking for
* @return A collection of Adapters
*/
public Set getFreeAdapters(String adapterType, String address) {
AskFastRestService service = getRestService();
return service.getFreeAdapters(adapterType, address);
}
public List getCapableAdapters(String type) {
AskFastRestService service = getRestService();
return service.getCapableAdapters(type);
}
/**
* Create a dialog. The system assigns an id to the dialog.
*
* @param dialog
* The dialog
*
* @return The dialog
*/
public Dialog createDialog(Dialog dialog) {
AskFastRestService service = getRestService();
return service.createDialog(dialog);
}
/**
* Returns the list of dialogs.
*
* @return The list of dialogs
*/
public Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy