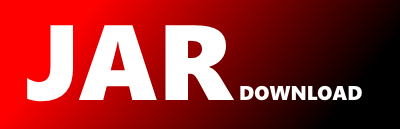
com.askfast.askfastapi.model.MediaProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of askfast-api-java Show documentation
Show all versions of askfast-api-java Show documentation
AskFast Library to use the AskFast system
package com.askfast.askfastapi.model;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonCreator;
public class MediaProperty
{
public enum MediaPropertyKey
{
/**
* defines the timeout associated with the call
*/
TIMEOUT,
/**
* defines if the answer is given via dtmf, text etc
*/
ANSWER_INPUT,
/**
* defines the length of th answer input. Typically dtmf
*/
ANSWER_INPUT_MIN_LENGTH,
ANSWER_INPUT_MAX_LENGTH,
/**
* defines a subtype for the question type.
* E.g. open question with type: audio refers to voicemail
*/
TYPE,
/**
* defines the length of the voicemail to be recorded
*/
VOICE_MESSAGE_LENGTH,
/**
* defines the endpoint where the recording message must be posted to
*/
VOICE_MESSAGE_URL,
/**
* defines the number of times the question should repeat in case of a wrong answer input.
* works only for phonecalls so as to end a call with repeated input errors.
*/
RETRY_LIMIT,
/**
* boolean flag to indicate if a voice mssage start should be indicated by a beep or not
*/
VOICE_MESSAGE_BEEP,
/**
* boolean flag to indicate if the call should terminate when a dtmf is pressed
*/
DTMF_TERMINATE,
/**
* defines the speed at which the TTS is spoken by the TTS engine
*/
TSS_SPEED,
/**
* defines which caller id should be when redirecting a call. If this is set to true
* the caller id of the connected user will be used. If false the adapterid caller id will
* be used. (Default: false)
*/
USE_EXTERNAL_CALLERID,
/**
* Pre-connect message. Gives opertunity to play a message before connecting the redirected call
*/
USE_PRECONNECT,
/**
* The conference room name that has to be used to add a call to.
*/
CONFERENCE_ROOM_NAME,
/**
* The conference starts when this flag is set to true. If not found,
* the conference starts as soon as there are two people in the
* conference
*/
CONFERENCE_START_ON_CONNECT,
/**
* The conference ends when this flag is set to true. If not found, the
* conference ends as soon as all participants leave the conference
*/
CONFERENCE_END_ON_DISCONNECT,
/**
* A valid url must be set against this key. This audio is played when
* participant is waiting in a conference room to be connected to other
* participants
*/
CONFERENCE_WAIT_URL,
/**
* Define how long you want the call to be in Secs
*/
CALL_LENGTH,
/**
* Any time during the conference, if this is set to true. Will exit
* the conference and perform the next sequence of control events
*/
CONFERENCE_EXIT_ON_STAR,
/**
* If this is set to true. Will generate the call transcript and POST it to the
* {@link MediaPropertyKey#TRANSCRIPT_URL}
* accepted values are true & false.
* default is false
*/
TRANSCRIPT,
/**
* The URL to which the transcript is POSTed to. This is used only
* if {@link MediaPropertyKey#TRANSCRIPT} is set to true
*/
TRANSCRIPT_URL,
/**
* The langauge of the recording file.
* Default is en-GB
*/
TRANSCRIPT_LANG,
/**
*
* The type of transcription service to use.
* It is used if transcript is set as true.
* Check for TRANSCRIPTION_SERVICES enum for various models.
* If null, Model1_G is used.
*/
TRANSCRIPT_SERVICE_TYPE,
/**
* Do voice Recording on separate channel
*/
RECORD_FROM_ANSWER_DUAL,
/**
* Do voice recording on single channel when the phone is answered.
*/
RECORD_FROM_ANSWER,
/**
* Start the voice recording when the phone starts ringing
*/
RECORD_FROM_RINGING,
/**
* Start the voice recording when the phone starts ringing.
* Do the voice recording of the calls on separate channel.
*/
RECORD_FROM_RINGING_DUAL,
/**
* Used to record the conference call. Starts recording when
* the conference call starts.
*/
RECORD_FROM_START,
MACHINE_DETECTION;
@JsonCreator
public static MediaPropertyKey fromJson(String name) {
return valueOf(name.toUpperCase());
}
}
public enum MediumType {
EMAIL, BROADSOFT, GTALK, SKYPE, SMS, TWITTER;
@JsonCreator
public static MediumType fromJson(String name) {
return valueOf(name.toUpperCase());
}
}
/**
* The services to select from.
* Model1_G is the default service
* if no model is selected.
*
* Default is NO_TRANSCRIPTION.
* Choose MODEL1_G, if you are unsure.
*
*/
public static enum TRANSCRIPTION_SERVICES {
MODEL1_G, MODEL2_T;
}
private MediumType medium;
private Map properties;
public MediaProperty() {
properties = new HashMap();
}
public MediumType getMedium()
{
return medium;
}
public void setMedium( MediumType medium )
{
this.medium = medium;
}
public Map getProperties()
{
return properties;
}
public void addProperty( MediaPropertyKey key, String value )
{
properties.put( key, value );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy