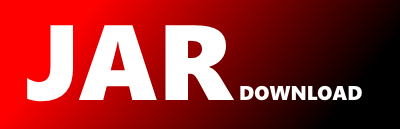
com.askfast.model.DDRRecord Maven / Gradle / Ivy
package com.askfast.model;
import java.util.Collection;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
@JsonIgnoreProperties(ignoreUnknown = true)
public class DDRRecord
{
/**
* status of the communication
*/
public enum CommunicationStatus
{
DELIVERED, RECEIVED, SENT, FINISHED, MISSED, ERROR, UNKNOWN;
@JsonCreator
public static CommunicationStatus fromJson( String name )
{
return valueOf( name.toUpperCase() );
}
}
private String id;
private String adapterId;
AdapterType adapterType;
private String accountId;
private String fromAddress;
private String toAddressString;
private String ddrTypeId;
private Integer quantity;
private Long start;
private Long duration;
Collection sessionKeys;
private CommunicationStatus status;
private Map statusPerAddress;
private Map additionalInfo;
private AccountType accountType;
private Double totalCost = 0.0;
private String parentId;
private Collection childIds;
public DDRRecord(){}
@JsonProperty("_id")
public String getId() {
return id;
}
@JsonProperty("_id")
public void setId(String id) {
this.id = id;
}
public String getAdapterId()
{
return adapterId;
}
public void setAdapterId( String adapterId )
{
this.adapterId = adapterId;
}
public String getAccountId()
{
return accountId;
}
public void setAccountId( String accountId )
{
this.accountId = accountId;
}
public String getFromAddress()
{
return fromAddress;
}
public void setFromAddress( String fromAddress )
{
this.fromAddress = fromAddress;
}
public String getToAddressString() {
return toAddressString;
}
public void setToAddressString(String toAddressString) {
this.toAddressString = toAddressString;
}
public String getDdrTypeId()
{
return ddrTypeId;
}
public void setDdrTypeId( String ddrTypeId )
{
this.ddrTypeId = ddrTypeId;
}
public Integer getQuantity()
{
return quantity != null ? quantity : 0;
}
public void setQuantity( Integer quantity )
{
this.quantity = quantity;
}
public Long getStart()
{
return start;
}
public void setStart( Long start )
{
this.start = start;
}
public CommunicationStatus getStatus() {
return status;
}
public void setStatus(CommunicationStatus status) {
this.status = status;
}
public Map getStatusPerAddress() {
return statusPerAddress;
}
public void setStatusPerAddress(Map statusPerAddress) {
this.statusPerAddress = statusPerAddress;
}
public Long getDuration()
{
return duration;
}
public void setDuration( Long duration )
{
this.duration = duration;
}
public Double getTotalCost() {
return totalCost;
}
public void setTotalCost(Double totalCost) {
this.totalCost = totalCost != null ? totalCost : 0.0;
}
public Map getAdditionalInfo() {
return additionalInfo;
}
public void setAdditionalInfo(Map additionalInfo) {
this.additionalInfo = additionalInfo;
}
public AccountType getAccountType() {
return accountType;
}
public void setAccountType(AccountType accountType) {
this.accountType = accountType;
}
public Collection getSessionKeys() {
return sessionKeys;
}
public void setSessionKeys(Collection sessionKeys) {
this.sessionKeys = sessionKeys;
}
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public Collection getChildIds() {
return childIds;
}
public void setChildIds(Collection childIds) {
this.childIds = childIds;
}
public AdapterType getAdapterType() {
return adapterType;
}
public void setAdapterType(AdapterType adapterType) {
this.adapterType = adapterType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy