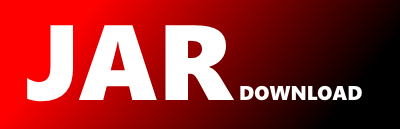
com.askfast.model.DialogRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of askfast-api-java Show documentation
Show all versions of askfast-api-java Show documentation
AskFast Library to use the AskFast system
package com.askfast.model;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import com.askfast.askfastapi.AskFastRestClient;
import com.askfast.askfastapi.model.Question;
import com.fasterxml.jackson.annotation.JsonIgnore;
public class DialogRequest {
private String address;
private Collection addressList;
private Map addressMap;
private Map addressCcMap;
private Map addressBccMap;
private String senderName;
private String subject;
private String url;
private AdapterType adapterType;
private String adapterID;
/**
* A detailed consturctor which can be used to send a broadcast with a
* specific senderName
*
* @param addressMap
* The key value pairs of {toAddress, recipientName}
* @param addressCcMap
* The key value pairs of {ccAddress, recipientName}. Used in
* case of an email adapter only
* @param addressBccMap
* The key value pairs of {bccAddress, recipientName}. Used in
* case of an email adapter only
* @param adapterType
* The type of communication opted for this outbound dialog. The
* first adapter of the given type is chosen if there are
* multiple ones exist. Usually either one of adapterType or
* adapterID is passed, although this constructor ignores the
* adapterType if the adapterID is present. An error is returned
* if its set outside of this constructor.
* @param adapterID
* The id identifying a particular mode of communication. These
* values can be retried from
* ASK-Fast Portal in
* the adapters section.
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
*/
public DialogRequest(Map addressMap, Map addressCcMap,
Map addressBccMap, AdapterType adapterType, String adapterID, String senderName,
String subject, String url) {
this.addressMap = addressMap;
this.addressCcMap = addressCcMap;
this.addressBccMap = addressBccMap;
this.adapterID = adapterID;
//use the adapterType only if the adapterID is null
if (adapterID == null) {
this.adapterType = adapterType;
}
this.senderName = senderName;
this.subject = subject;
this.url = url;
}
/**
* A consturctor which can be used to trigger an outbound request
*
* @param address
* The address of the receipient. E.g. Phonenumber for Call, SMS
* and Email for Email adpater.
* @param adapterType
* The type of communication opted for this outbound dialog. The
* first adapter of the given type is chosen if there are
* multiple ones exist. Usually either one of adapterType or
* adapterID is passed, although this constructor ignores the
* adapterType if the adapterID is present. An error is returned
* if its set outside of this constructor.
* @param adapterId
* The id identifying a particular mode of communication. These
* values can be retried from
* ASK-Fast Portal in
* the adapters section.
* @param senderName
* A senderName can be attached for medium types: SMS, EMAIL. For
* SMS, the length should not exceed 11 charecters.
* @param subject
* Only valid for an email adapter. The subject of the message to
* be sent
* @param url
* This can be one of:
* 1. http(s) endpoint fetching a {@link Question} json as
* response.
* 2. A standard text prefixed by keyword: text://your message.
* This will be transformed to a
* {@link Question#QUESTION_TYPE_COMMENT}
* 3. A unique dialogId as defined in the
* ASK-Fast Portal or
* by method {@link AskFastRestClient#createDialog(Dialog)}
* method
*/
public DialogRequest(String address, AdapterType adapterType, String adapterId, String senderName, String subject,
String url) {
this.address = address;
this.adapterID = adapterId;
//use the adapterType only if the adapterID is null
if (adapterID == null) {
this.adapterType = adapterType;
}
this.senderName = senderName;
this.subject = subject;
this.url = url;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Collection getAddressList() {
return addressList;
}
public void setAddressList(Collection addressList) {
this.addressList = addressList;
}
public Map getAddressMap() {
return addressMap;
}
public void setAddressMap(Map addressMap) {
this.addressMap = addressMap;
}
public Map getAddressCcMap() {
return addressCcMap;
}
public void setAddressCcMap(Map addressCcMap) {
this.addressCcMap = addressCcMap;
}
public Map getAddressBccMap() {
return addressBccMap;
}
public void setAddressBccMap(Map addressBccMap) {
this.addressBccMap = addressBccMap;
}
public String getSenderName() {
return senderName;
}
public void setSenderName(String senderName) {
this.senderName = senderName;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public AdapterType getAdapterType() {
return adapterType;
}
public void setAdapterType(AdapterType adapterType) {
this.adapterType = adapterType;
}
public String getAdapterID() {
return adapterID;
}
public void setAdapterID(String adapterID) {
this.adapterID = adapterID;
}
/**
* Collates all the addresses in the address, addressList, addressMaps
* @return All the addresses as a map
*/
@JsonIgnore
public HashMap getAllAddresses() {
HashMap result = new HashMap();
if (address != null) {
result.put(address, "");
}
if (addressList != null && !addressList.isEmpty()) {
for (String address : addressList) {
result.put(address, "");
}
}
if (addressMap != null && !addressMap.isEmpty()) {
result.putAll(addressMap);
}
if (getAddressCcMap() != null && !addressCcMap.isEmpty()) {
result.putAll(addressCcMap);
}
if (getAddressBccMap() != null && !addressBccMap.isEmpty()) {
result.putAll(addressBccMap);
}
return result;
}
/**
* Just validates the request payload
* @return If this dialogRequest if formatted properly
*/
@JsonIgnore
public boolean isValidRequest() {
if (getAllAddresses().isEmpty() || url == null || (adapterID == null && adapterType == null)) {
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy