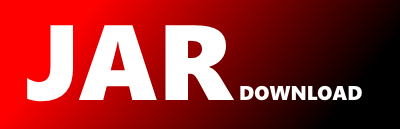
com.aspectran.demo.terminal.TransletInterpreter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aspectran-demo Show documentation
Show all versions of aspectran-demo Show documentation
Aspectran module showcasing a demo application
/*
* Copyright (c) 2008-2020 The Aspectran Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aspectran.demo.terminal;
import com.aspectran.core.activity.ActivityException;
import com.aspectran.core.activity.InstantActivity;
import com.aspectran.core.activity.Translet;
import com.aspectran.core.activity.TransletNotFoundException;
import com.aspectran.core.component.bean.annotation.Bean;
import com.aspectran.core.component.bean.annotation.Component;
import com.aspectran.core.component.bean.annotation.RequestToGet;
import com.aspectran.core.component.bean.annotation.RequestToPost;
import com.aspectran.core.component.bean.annotation.Transform;
import com.aspectran.core.component.bean.aware.ActivityContextAware;
import com.aspectran.core.context.ActivityContext;
import com.aspectran.core.context.expr.token.Token;
import com.aspectran.core.context.expr.token.TokenParser;
import com.aspectran.core.context.rule.DescriptionRule;
import com.aspectran.core.context.rule.ItemRule;
import com.aspectran.core.context.rule.ItemRuleMap;
import com.aspectran.core.context.rule.TransletRule;
import com.aspectran.core.context.rule.type.MethodType;
import com.aspectran.core.context.rule.type.TokenType;
import com.aspectran.core.context.rule.type.TransformType;
import com.aspectran.core.util.StringUtils;
import com.aspectran.core.util.json.JsonWriter;
import com.aspectran.core.util.logging.Log;
import com.aspectran.core.util.logging.LogFactory;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
@Component("/terminal")
@Bean("transletInterpreter")
public class TransletInterpreter implements ActivityContextAware {
private final Log log = LogFactory.getLog(TransletInterpreter.class);
private ActivityContext context;
@Override
public void setActivityContext(ActivityContext context) {
this.context = context;
}
@RequestToGet("/query/@{_translet_}")
@Transform(type = TransformType.TEXT, contentType = "application/json")
public void query(Translet translet) throws IOException {
String transletName = translet.getAttribute("_translet_");
if (StringUtils.isEmpty(transletName)) {
return;
}
TransletRule transletRule = context.getTransletRuleRegistry().getTransletRule(transletName);
if (transletRule == null) {
if (log.isDebugEnabled()) {
log.debug("Translet not found: " + transletName);
}
JsonWriter jsonWriter = new JsonWriter(translet.getResponseAdapter().getWriter());
jsonWriter.beginObject();
jsonWriter.writeName("translet");
jsonWriter.writeNull();
jsonWriter.writeName("request");
jsonWriter.writeNull();
jsonWriter.writeName("response");
jsonWriter.writeNull();
jsonWriter.endObject();
return;
}
ItemRuleMap parameterItemRuleMap = transletRule.getRequestRule().getParameterItemRuleMap();
ItemRuleMap attributeItemRuleMap = transletRule.getRequestRule().getAttributeItemRuleMap();
JsonWriter jsonWriter = new JsonWriter(translet.getResponseAdapter().getWriter());
jsonWriter.beginObject();
jsonWriter.writeName("translet");
jsonWriter.beginObject();
jsonWriter.writeName("name");
jsonWriter.write(transletRule.getName());
if (transletRule.getDescriptionRule() != null) {
String description = DescriptionRule.render(transletRule.getDescriptionRule(), context.getCurrentActivity());
jsonWriter.writeName("description");
jsonWriter.write(description);
}
jsonWriter.endObject();
jsonWriter.writeName("request");
jsonWriter.beginObject();
if (parameterItemRuleMap != null) {
jsonWriter.writeName("parameters");
jsonWriter.beginObject();
jsonWriter.writeName("items");
jsonWriter.write(toListForItems(parameterItemRuleMap.values()));
jsonWriter.writeName("tokens");
jsonWriter.write(toListForTokens(parameterItemRuleMap.values()));
jsonWriter.endObject();
}
if (attributeItemRuleMap != null) {
jsonWriter.writeName("attributes");
jsonWriter.beginObject();
jsonWriter.writeName("items");
jsonWriter.write(toListForItems(attributeItemRuleMap.values()));
jsonWriter.writeName("tokens");
jsonWriter.write(toListForTokens(attributeItemRuleMap.values()));
jsonWriter.endObject();
}
jsonWriter.endObject();
jsonWriter.writeName("response");
jsonWriter.beginObject();
if (transletRule.getResponseRule().getResponse() != null) {
jsonWriter.writeName("contentType");
jsonWriter.writeValue(transletRule.getResponseRule().getResponse().getContentType());
}
jsonWriter.endObject();
jsonWriter.endObject();
}
@RequestToPost("/exec/@{_translet_}")
public void execute(Translet translet) {
String transletName = translet.getAttribute("_translet_");
if (StringUtils.isEmpty(transletName)) {
return;
}
try {
performActivity(transletName);
} catch (ActivityException e) {
log.error("Failed to execute translet: " + transletName, e);
}
}
private void performActivity(String transletName) throws ActivityException {
TransletRule transletRule = context.getTransletRuleRegistry().getTransletRule(transletName);
if (transletRule == null) {
throw new TransletNotFoundException(transletName, MethodType.GET);
}
InstantActivity activity = new InstantActivity(context);
activity.setSessionAdapter(context.getCurrentActivity().getSessionAdapter());
activity.setRequestAdapter(context.getCurrentActivity().getRequestAdapter());
activity.setResponseAdapter(context.getCurrentActivity().getResponseAdapter());
activity.prepare(transletName, transletRule);
activity.perform();
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy