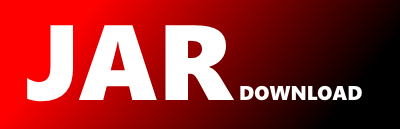
com.aspectran.core.activity.Translet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aspectran Show documentation
Show all versions of aspectran Show documentation
Aspectran is a lightweight Java framework for building Enterprise-ready Web applications.
Also, It will be able to launch as a Console-based and Embedded application.
/**
* Copyright 2008-2016 Juho Jeong
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aspectran.core.activity;
import java.util.Enumeration;
import java.util.Map;
import com.aspectran.core.activity.process.result.ProcessResult;
import com.aspectran.core.activity.request.parameter.FileParameter;
import com.aspectran.core.activity.response.Response;
import com.aspectran.core.adapter.ApplicationAdapter;
import com.aspectran.core.adapter.RequestAdapter;
import com.aspectran.core.adapter.ResponseAdapter;
import com.aspectran.core.adapter.SessionAdapter;
import com.aspectran.core.context.bean.BeanRegistry;
import com.aspectran.core.context.message.MessageSource;
import com.aspectran.core.context.message.NoSuchMessageException;
import com.aspectran.core.context.rule.AspectAdviceRule;
import com.aspectran.core.context.rule.ForwardResponseRule;
import com.aspectran.core.context.rule.RedirectResponseRule;
import com.aspectran.core.context.rule.TransformRule;
import com.aspectran.core.context.rule.type.MethodType;
/**
* The Interface Translet.
*
* Created: 2008. 7. 5. AM 12:35:44
*/
public interface Translet extends BeanRegistry, MessageSource {
/**
* Returns the name of the translet.
*
* @return the translet name
*/
String getTransletName();
/**
* Gets the request http method.
*
* @return the request method
*/
MethodType getRequestMethod();
/**
* Gets the application adapter.
*
* @return the application adapter
*/
ApplicationAdapter getApplicationAdapter();
/**
* Gets the session adapter.
*
* @return the session adapter
*/
SessionAdapter getSessionAdapter();
/**
* Gets the request adapter.
*
* @return the request adapter
*/
RequestAdapter getRequestAdapter();
/**
* Gets the response adapter.
*
* @return the response adapter
*/
ResponseAdapter getResponseAdapter();
/**
* Returns the adaptee object to provide request information.
*
* @param the type of the request adaptee
* @return the request adaptee object
*/
T getRequestAdaptee();
/**
* Returns the adaptee object to provide response information.
*
* @param the type of the response adaptee
* @return the response adaptee object
*/
T getResponseAdaptee();
/**
* Returns the adaptee object to provide session information.
*
* @param the type of the session adaptee
* @return the session adaptee object
*/
T getSessionAdaptee();
/**
* Returns the adaptee object to provide application information.
*
* @param the type of the application adaptee
* @return the application adaptee object
*/
T getApplicationAdaptee();
/**
* Returns the process result.
*
* @return the process result
*/
ProcessResult getProcessResult();
/**
* Returns a action result for the specified action id from the process result,
* or {@code null} if the action does not exist.
*
* @param actionId the specified action id
* @return the action result
*/
Object getProcessResult(String actionId);
/**
* Sets the process result.
*
* @param processResult the new process result
*/
void setProcessResult(ProcessResult processResult);
/**
* Returns the ProcessResult.
* If not yet instantiated then create a new one.
*
* @return the process result
*/
ProcessResult touchProcessResult();
/**
* Returns the process result with the contents name.
* If not yet instantiated then create a new one.
*
* @param contentsName the contents name
* @return the process result
*/
ProcessResult touchProcessResult(String contentsName);
/**
* Returns the process result with the contents name.
* If not yet instantiated then create a new one.
*
* @param contentsName the contents name
* @param initialCapacity the initial capacity of the process result
* @return the process result
*/
ProcessResult touchProcessResult(String contentsName, int initialCapacity);
/**
* Returns the activity's data map.
*
* @return the activity's data map
*/
ActivityDataMap getActivityDataMap();
/**
* Returns the activity's data map.
*
* @param prefill whether data pre-fill.
* @return the activity data map
*/
ActivityDataMap getActivityDataMap(boolean prefill);
/**
* Returns the value of an activity's request parameter as a {@code String},
* or {@code null} if the parameter does not exist.
*
* @param name a {@code String} specifying the name of the parameter
* @return a {@code String} representing the
* single value of the parameter
* @see #getParameterValues
*/
String getParameter(String name);
/**
* Returns an array of {@code String} objects containing all
* of the values the given activity's request parameter has,
* or {@code null} if the parameter does not exist.
*
* @param name a {@code String} specifying the name of the parameter
* @return an array of {@code String} objects
* containing the parameter's values
* @see #getParameter
*/
String[] getParameterValues(String name);
/**
* Returns an {@code Enumeration} of {@code String} objects containing
* the names of the parameters contained in this request.
* If the request has no parameters, the method returns an empty {@code Enumeration}.
*
* @return an {@code Enumeration} of {@code String} objects, each {@code String}
* containing the name of a request parameter;
* or an empty {@code Enumeration} if the request has no parameters
*/
Enumeration getParameterNames();
/**
* Sets the value to the parameter with the given name.
*
* @param name a {@code String} specifying the name of the parameter
* @param value a {@code String} representing the
* single value of the parameter
* @see #setParameter(String, String[])
*/
void setParameter(String name, String value);
/**
* Sets the value to the parameter with the given name.
*
* @param name a {@code String} specifying the name of the parameter
* @param values an array of {@code String} objects
* containing the parameter's values
* @see #setParameter
*/
void setParameter(String name, String[] values);
/**
* Returns a {@code FileParameter} object as a given activity's request parameter name,
* or {@code null} if the parameter does not exist.
*
* @param name a {@code String} specifying the name of the file parameter
* @return a {@code FileParameter} representing the
* single value of the parameter
* @see #getFileParameterValues
*/
FileParameter getFileParameter(String name);
/**
* Returns an array of {@code FileParameter} objects containing all
* of the values the given activity's request parameter has,
* or {@code null} if the parameter does not exist.
*
* @param name a {@code String} specifying the name of the file parameter
* @return an array of {@code FileParameter} objects
* containing the parameter's values
* @see #getFileParameter
*/
FileParameter[] getFileParameterValues(String name);
/**
* Returns an {@code Enumeration} of {@code String} objects containing
* the names of the file parameters contained in this request.
* If the request has no parameters, the method returns an empty {@code Enumeration}.
*
* @return an {@code Enumeration} of {@code String} objects, each {@code String}
* containing the name of a file parameter;
* or an empty {@code Enumeration} if the request has no file parameters
*/
Enumeration getFileParameterNames();
/**
* Sets the {@code FileParameter} object to the file parameter with the given name.
*
* @param name a {@code String} specifying the name of the file parameter
* @param fileParameter a {@code FileParameter} representing the
* single value of the parameter
* @see #setFileParameter(String, FileParameter[])
*/
void setFileParameter(String name, FileParameter fileParameter);
/**
* Sets the value to the file parameter with the given name.
*
* @param name a {@code String} specifying the name of the file parameter
* @param fileParameters an array of {@code FileParameter} objects
* containing the file parameter's values
* @see #setFileParameter
*/
void setFileParameter(String name, FileParameter[] fileParameters);
/**
* Removes the file parameter with the specified name.
*
* @param name a {@code String} specifying the name of the file parameter
* @return the removed file parameters
*/
FileParameter[] removeFileParameter(String name);
/**
* Returns the value of the named attribute as a given type,
* or {@code null} if no attribute of the given name exists.
*
* @param the generic type
* @param name a {@code String} specifying the name of the attribute
* @return an {@code Object} containing the value of the attribute,
* or {@code null} if the attribute does not exist
*/
T getAttribute(String name);
/**
* Stores an attribute in this request.
*
* @param name specifying the name of the attribute
* @param value the {@code Object} to be stored
*/
void setAttribute(String name, Object value);
/**
* Returns an {@code Enumeration} containing the
* names of the attributes available to this request.
* This method returns an empty {@code Enumeration}
* if the request has no attributes available to it.
*
* @return the attribute names
*/
Enumeration getAttributeNames();
/**
* Removes an attribute from this request.
*
* @param name a {@code String} specifying the name of the attribute to remove
*/
void removeAttribute(String name);
/**
* Return a mutable Map of the request parameters,
* with parameter names as map keys and parameter values as map values.
* If the parameter value type is the String then map value will be of type String.
* If the parameter value type is the String array then map value will be of type String array.
*
* @return the parameter map
* @since 1.4.0
*/
Map getParameterMap();
/**
* Fills all parameters to the specified map.
*
* @param parameterMap the parameter map
* @since 2.0.0
*/
void fillPrameterMap(Map parameterMap);
/**
* Return a mutable {@code Map} of the request attributes,
* with attribute names as map keys and attribute value as map value.
*
* @return the attribute map
* @since 2.0.0
*/
Map getAttributeMap();
/**
* Fills all attributes to the specified map.
*
* @param attributeMap the attribute map
* @since 2.0.0
*/
void fillAttributeMap(Map attributeMap);
/**
* Respond immediately, and the remaining jobs will be canceled.
*/
void response();
/**
* Respond immediately, and the remaining jobs will be canceled.
*
* @param response the response
*/
void response(Response response);
/**
* Transformation according to a given rule, and transmits this response.
*
* @param transformRule the transformation rule
*/
void transform(TransformRule transformRule);
/**
* Redirect a client according to the given rule.
*
* @param redirectResponseRule the redirect response rule
*/
void redirect(RedirectResponseRule redirectResponseRule);
/**
* Redirect a client to a new target resource.
* If an intended redirect response rule exists, that may be used.
*
* @param target the target resource
*/
void redirect(String target);
/**
* Redirect a client to a new target resource.
* If {@code immediately} is true, create a new redirect response rule
* and overrided the intended redirect resoponse rule.
*
* @param target the target resource
* @param immediately whether to override the intended redirect response rule
*/
void redirect(String target, boolean immediately);
/**
* Redirect to the other target resouce.
*
* @param target the redirect target
* @param parameters the parameters
*/
void redirect(String target, Map parameters);
/**
* Forward according to a given rule.
*
* @param forwardResponseRule the forward response rule
*/
void forward(ForwardResponseRule forwardResponseRule);
/**
* Forward to the specified translet immediately.
*
* @param transletName the translet name of the target to be forwarded
*/
void forward(String transletName);
/**
* Forward to the specified translet.
*
* @param transletName the translet name
* @param immediately whether forwarding immediately
*/
void forward(String transletName, boolean immediately);
/**
* Returns whether the exception was thrown.
*
* @return true, if is exception raised
*/
boolean isExceptionRaised();
/**
* Returns the raised exception instance.
*
* @return the raised exception instance
*/
Throwable getRaisedException();
/**
* Returns the origin raised exception instance.
*
* @return the origin raised exception instance
*/
Throwable getOriginRaisedException();
/**
* Gets the aspect advice bean.
*
* @param the generic type
* @param aspectId the aspect id
* @return the aspect advice bean
*/
T getAspectAdviceBean(String aspectId);
/**
* Put aspect advice bean.
*
* @param aspectId the aspect id
* @param adviceBean the advice bean
*/
void putAspectAdviceBean(String aspectId, Object adviceBean);
/**
* Gets the before advice result.
*
* @param the generic type
* @param aspectId the aspect id
* @return the before advice result
*/
T getBeforeAdviceResult(String aspectId);
/**
* Gets the after advice result.
*
* @param the generic type
* @param aspectId the aspect id
* @return the after advice result
*/
T getAfterAdviceResult(String aspectId);
/**
* Gets the finally advice result.
*
* @param the generic type
* @param aspectId the aspect id
* @return the finally advice result
*/
T getFinallyAdviceResult(String aspectId);
/**
* Put advice result.
*
* @param aspectAdviceRule the aspect advice rule
* @param adviceActionResult the advice action result
*/
void putAdviceResult(AspectAdviceRule aspectAdviceRule, Object adviceActionResult);
/**
* Returns an interface class for the {@code Translet}.
*
* @return the interface class for the translet
*/
Class extends Translet> getTransletInterfaceClass();
/**
* Returns an implementation class for the {@code Translet}.
*
* @return the implementation class for the translet
*/
Class extends CoreTranslet> getTransletImplementationClass();
/**
* Return whether the given profile is active.
* If active profiles are empty whether the profile should be active by default.
*
* @param profiles the profiles
* @return {@code true} if profile is active, otherwise {@code false}
*/
boolean acceptsProfiles(String... profiles);
/**
* Try to resolve the message. Return default message if no message was found.
*
* @param code the code to lookup up, such as 'calculator.noRateSet'. Users of
* this class are encouraged to base message names on the relevant fully
* qualified class name, thus avoiding conflict and ensuring maximum clarity.
* @param args array of arguments that will be filled in for params within
* the message (params look like "{0}", "{1,date}", "{2,time}" within a message),
* or {@code null} if none.
* @param defaultMessage String to return if the lookup fails
* @return the resolved message if the lookup was successful;
* otherwise the default message passed as a parameter
* @see java.text.MessageFormat
*/
String getMessage(String code, Object[] args, String defaultMessage);
/**
* Try to resolve the message. Treat as an error if the message can't be found.
*
* @param code the code to lookup up, such as 'calculator.noRateSet'
* @param args Array of arguments that will be filled in for params within
* the message (params look like "{0}", "{1,date}", "{2,time}" within a message),
* or {@code null} if none.
* @return the resolved message
* @throws NoSuchMessageException if the message wasn't found
* @see java.text.MessageFormat
*/
String getMessage(String code, Object[] args) throws NoSuchMessageException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy