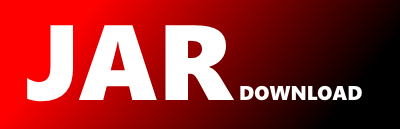
com.asprise.imaging.core.util.TranslationProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-scanner-access-twain Show documentation
Show all versions of java-scanner-access-twain Show documentation
Acquire images from and have total control on almost all kinds of scanners on both Windows 32bit and 64bit.
/**********************************************************************************************
*
* Asprise Scanning and Imaging API - http://asprise.com/document-scanner-image-pdf/java-scanning-api-overview.html
* Copyright (C) 1998-2018. Asprise Inc.
*
* This file is licensed under the GNU Affero General Public License version 3 as published by
* the Free Software Foundation.
*
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*
* You should have received a copy of the GNU Affero General Public License. If not, please
* visit .
*
**********************************************************************************************/
package com.asprise.imaging.core.util;
import com.asprise.imaging.core.Imaging;
import com.asprise.imaging.core.scan.twain.CapOptions;
import com.asprise.imaging.core.util.system.StringUtils;
import com.asprise.imaging.core.util.system.Utils;
import javax.swing.*;
import java.io.*;
import java.nio.charset.Charset;
import java.util.*;
public class TranslationProps {
public static boolean setI18n(JComboBox capOptionsComboBoxModelDriven, Properties props, String propKey) {
CapOptions.CapOptionsComboBoxModel model = (CapOptions.CapOptionsComboBoxModel) capOptionsComboBoxModelDriven.getModel();
String value = getPropValueIfObjectNonNull(model, props, propKey);
if(value == null) {
return false;
}
Map map = StringUtils.propertiesStringToMap(value, "|", "=", false);
boolean updated = false;
for(int i = 0; i < model.getSize(); i++) {
CapOptions.CapOptionItem item = (CapOptions.CapOptionItem) model.getElementAt(i);
String key = String.valueOf(item.getValue());
if(map.containsKey(key)) {
String label = map.get(key);
if(label != null && label.trim().length() > 0) {
item.setLabel(label);
updated = true;
}
}
}
if(updated) {
capOptionsComboBoxModelDriven.repaint();
}
return true;
}
public static boolean setI18n(JComboBox capOptionsComboBoxModelDriven, String formatter) {
if(formatter == null || formatter.trim().length() == 0) {
return false;
}
CapOptions.CapOptionsComboBoxModel model = (CapOptions.CapOptionsComboBoxModel) capOptionsComboBoxModelDriven.getModel();
boolean updated = false;
for(int i = 0; i < model.getSize(); i++) {
CapOptions.CapOptionItem item = (CapOptions.CapOptionItem) model.getElementAt(i);
try {
String label = String.format(formatter, item.getValue());
item.setLabel(label);
} catch (Exception e) {
e.printStackTrace();
}
updated = true;
}
if(updated) {
capOptionsComboBoxModelDriven.repaint();
}
return true;
}
public static boolean setI18n(JMenu menu, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(menu, props, propKey);
if(value == null) {
return false;
}
menu.setName(value);
menu.setText(value);
return true;
}
public static boolean setI18n(AbstractAction action, Properties props, String propKey, String actionValueKey) {
String value = getPropValueIfObjectNonNull(action, props, propKey);
if(value == null) {
return false;
}
action.putValue(actionValueKey, value);
return true;
}
public static boolean setI18n(AbstractAction action, Properties props, String propKey) {
return setI18n(action, props, propKey, Action.NAME);
}
public static boolean setI18n(AbstractAction action, Properties props, String propKey, boolean shortDesc) {
String value = getPropValueIfObjectNonNull(action, props, propKey);
if(value == null) {
return false;
}
action.putValue(shortDesc ? Action.SHORT_DESCRIPTION : Action.NAME, value);
return true;
}
public static boolean setI18n(JButton button, boolean tooltip, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(button, props, propKey);
if(value == null) {
return false;
}
if(tooltip) {
button.setToolTipText(value);
} else {
button.setText(value);
}
return true;
}
public static boolean setI18nTooltip(JComponent comp, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(comp, props, propKey);
if(value == null) {
return false;
}
comp.setToolTipText(value);
return true;
}
public static boolean setI18n(JLabel label, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(label, props, propKey);
if(value == null) {
return false;
}
label.setText(value);
return true;
}
public static boolean setI18n(JDialog dialog, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(dialog, props, propKey);
if(value == null) {
return false;
}
dialog.setTitle(value);
return true;
}
public static boolean setI18n(JCheckBox checkBox, Properties props, String propKey) {
String value = getPropValueIfObjectNonNull(checkBox, props, propKey);
if(value == null) {
return false;
}
checkBox.setText(value);
return true;
}
public static String getPropValueIfObjectNonNull(Object object, Properties props, String propKey) {
if(object == null || props == null || propKey == null) {
return null;
}
String value = props.getProperty(propKey);
if(value == null || value.trim().length() == 0) {
return null;
}
return value;
}
public static String getPropValue(Properties props, String propKey, String defaultValue) {
if(props == null || propKey == null) {
return defaultValue;
}
String value = props.getProperty(propKey);
if(value == null || value.trim().length() == 0) {
return defaultValue;
}
return value;
}
public static Properties combineProps(Properties base, Properties overriding) {
if(overriding == null) {
return base;
}
if(base == null) {
return overriding;
}
Properties props = base == null ? new Properties() : new Properties(base);
Enumeration keyNames = overriding.propertyNames();
while(keyNames.hasMoreElements()) {
Object key = keyNames.nextElement();
if(key instanceof String) {
props.setProperty((String)key, overriding.getProperty((String) key));
}
}
return props;
}
public static Properties loadLangIfAvailable(Properties props, String propertiesFilePrefix) {
if(props == null) {
return null;
}
String lang = props.getProperty("lang");
if("system".equalsIgnoreCase(lang) || "user".equalsIgnoreCase(lang)) {
lang = Imaging.getSystemInfoUserLocale();
if(lang == null || lang.trim().length() == 0) {
lang = Locale.getDefault().getLanguage();
}
}
if(lang != null && lang.toLowerCase().startsWith("en")) {
lang = "";
}
Properties base = null;
if(lang != null) {
lang = lang.trim();
lang = lang.replace('-', '_');
base = TranslationProps.loadProps(propertiesFilePrefix, lang);
if(base == null && lang.contains("_")) {
base = TranslationProps.loadProps(propertiesFilePrefix, lang.substring(0, lang.indexOf('_')));
}
}
return TranslationProps.combineProps(base, props);
}
public static Properties loadProps(String propertiesFilePrefix, String langCode) {
if(langCode != null) {
langCode = langCode.replace('-', '_');
}
Properties prop = new Properties();
String propFileName = propertiesFilePrefix
+ (langCode == null || langCode.trim().length() == 0 ? "" : "_" + langCode.trim())
+ ".properties";
InputStream inputStream = Imaging.class.getClassLoader().getResourceAsStream(propFileName);
if (inputStream != null) {
try {
Utils.readPropertiesFromUtf8(inputStream, prop);
return prop;
} catch (Throwable e) {
e.printStackTrace();
return null;
}
} else {
return null;
}
}
public static List listLangs(String propertiesFilePrefix) {
List langs = new ArrayList();
List files = Utils.searchInCodeSourceRoot(propertiesFilePrefix + "*.properties");
for(int i = 0; files != null && i < files.size(); i++) {
String file = files.get(i);
file = file.substring(0, file.length() - ".properties".length());
file = file.substring(propertiesFilePrefix.length());
if(file.length() > 0 && (file.charAt(0) == '_' || file.charAt(0) == '-')) {
file = file.substring(1);
}
langs.add(file);
}
return langs;
}
public static class Lang {
public String code;
public String name;
public Lang(String code, String name) {
this.code = code;
this.name = name;
}
@Override
public String toString() {
return name;
}
public static List getLangs() {
List langs = new ArrayList();
langs.add(new Lang("en", "English"));
langs.add(new Lang("de", "Deutsch"));
langs.add(new Lang("es", "Español"));
langs.add(new Lang("fr", "Français"));
langs.add(new Lang("it", "Italiano"));
langs.add(new Lang("pt", "Português"));
langs.add(new Lang("ru", "Русский"));
langs.add(new Lang("ja", "日本語"));
langs.add(new Lang("zh", "中文"));
langs.add(new Lang("user", "User"));
return langs;
}
public static int getIndex(List langs, String code) {
if(langs == null || code == null) {
return -1;
}
for(int i = 0; i < langs.size(); i++) {
Lang lang = langs.get(i);
if(code.equalsIgnoreCase(lang.code)) {
return i;
}
}
for(int i = 0; code.length() >= 2 && i < langs.size(); i++) {
Lang lang = langs.get(i);
if(code.substring(0, 2).equalsIgnoreCase(lang.code.substring(0, 2))) {
return i;
}
}
return -1;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy