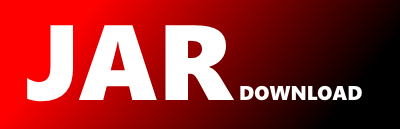
astra.reasoner.util.ContextEvaluateVisitor Maven / Gradle / Ivy
package astra.reasoner.util;
import java.util.HashMap;
import java.util.Map;
import astra.core.Intention;
import astra.formula.Formula;
import astra.formula.FormulaVariable;
import astra.formula.IsDone;
import astra.formula.IsNull;
import astra.formula.Predicate;
import astra.term.ModuleTerm;
import astra.term.NullTerm;
import astra.term.Primitive;
import astra.term.QueryTerm;
import astra.term.Term;
import astra.term.Variable;
import astra.type.Type;
public class ContextEvaluateVisitor extends AbstractEvaluateVisitor {
public static interface Handler {
public Class getType();
public Object handle(LogicVisitor visitor, T object, Intention context);
}
private static Map, Handler extends Formula>> formulaHandlers = new HashMap<>();
private static Map, Handler extends Term>> termHandlers = new HashMap<>();
public static void addFormulaHandler(Handler handler) {
formulaHandlers.put(handler.getType(), handler);
}
public static void addTermHandler(Handler handler) {
termHandlers.put(handler.getType(), handler);
}
@SuppressWarnings("unchecked")
private static Handler getFormulaHandler(Class cls) {
return (Handler) formulaHandlers.get(cls);
}
@SuppressWarnings("unchecked")
private static Handler getTermHandler(Class cls) {
return (Handler) termHandlers.get(cls);
}
Intention intention;
public ContextEvaluateVisitor(Intention intention) {
this(intention, false);
}
public ContextEvaluateVisitor(Intention intention, boolean passByValue) {
super(passByValue);
this.intention = intention;
addFormulaHandler(new Handler() {
public Class getType() { return IsDone.class; }
public Object handle(LogicVisitor visitor, IsDone isDone, Intention intention) {
return intention.isDone() ? Predicate.TRUE : Predicate.FALSE;
}
});
}
@Override
public Object visit(Formula formula) {
Handler handler = getFormulaHandler(formula.getClass());
if (handler == null) {
return super.visit(formula);
}
return handler.handle(this, formula, intention);
}
@Override
public Object visit(Term term) {
Handler handler = getTermHandler(term.getClass());
if (handler == null) {
return super.visit(term);
}
return handler.handle(this, term, intention);
}
static {
addTermHandler(new Handler() {
@Override public Class getType() { return Variable.class; }
@Override public Object handle(LogicVisitor visitor, Variable variable, Intention context) {
Term term = context.getValue(variable);
// System.out.println(variable + " (" + variable.id() + ") " + term);
// NOTE: Have added "|| term instanceof Variable" here to stop situations
// where variables that have been bound to other variables are substituted
// for the original variable.
// Specifically, this was causing a problem when an unbound variable is passed to
// a subgoal, and then the corresponding subgoal variable (which is still unbound)
// is passed to another subgoal:
//
// agent Test {
// module Console C;
//
// rule +!main(list args) {
// !subTest(string X);
// C.println("X="+X);
// }
//
// rule +!subTest(string Y) {
// !subsubTest(Y);
// C.println("Y="+Y);
// }
//
// rule +!subsubTest(string Z) {
// Z = "yo! boyo";
// }
// }
// The error occurs when declaring goal !subsubTest(Y)...
if (term == null || term instanceof Variable) return variable;
return term;
}
});
addTermHandler(new Handler() {
@Override public Class getType() { return ModuleTerm.class; }
@Override public Object handle(LogicVisitor visitor, ModuleTerm term, Intention context) {
Object value = term.evaluate(context);
if (value == null) return new NullTerm();
if (Type.getType(value).equals(Type.LIST) ||
Type.getType(value).equals(Type.FORMULA) ||
Type.getType(value).equals(Type.FUNCTION)) {
return value;
}
return Primitive.newPrimitive(value);
}
});
addTermHandler(new Handler() {
@Override public Class getType() { return QueryTerm.class; }
@Override public Object handle(LogicVisitor visitor, QueryTerm term, Intention context) {
// return Primitive.newPrimitive(context.query((Formula) term.formula().accept(visitor)) == null ? false:true);
return Primitive.newPrimitive(context.query((Formula) term.formula().accept(visitor)) != null);
}
});
addFormulaHandler(new Handler() {
@Override public Class getType() { return IsNull.class; }
@Override public Object handle(LogicVisitor visitor, IsNull isNull, Intention context) {
Term t = isNull.formula();
if (t instanceof Variable) {
Term term = context.getValue((Variable) t);
return (term == null) ? Predicate.TRUE : Predicate.FALSE;
} else if (t instanceof FormulaVariable) {
Term term = context.getValue(((FormulaVariable) t).variable());
return (term == null) ? Predicate.TRUE : Predicate.FALSE;
}
return Predicate.FALSE;
}
});
}
// @SuppressWarnings("unchecked")
// @Override
// public Object visit(CartagoProperty property) {
// Predicate obs_prop = null;
// if (property.target() != null) {
// obs_prop = CartagoAPI.get(context.agent).store().getObservableProperty(property.target(), property.content().predicate());
// } else {
// obs_prop = CartagoAPI.get(context.agent).store().getObservableProperty(property.content().predicate());
// }
//
// if (obs_prop == null) return property;
//
// Map b = Unifier.unify(obs_prop, property.content(), context.agent);
// for (Entry entry : b.entrySet()) {
// context.addVariable(new Variable(entry.getValue().type(), entry.getKey()), entry.getValue());
// }
//
// return (b == null) ? Predicate.FALSE : Predicate.TRUE;
// }
//
// @Override
// public Object visit(AcreFormula formula) {
// return new AcreFormula(
// (Term) formula.cid().accept(this),
// (Term) formula.index().accept(this),
// (Term) formula.type().accept(this),
// (Term) formula.performative().accept(this),
// (Formula) formula.content().accept(this)
// );
// }
// // Step 1: Get an initial list of matching conversations based on the conversation id.
// List conversations = new ArrayList();
// Term cid = (Term) formula.cid().accept(this);
// if (cid instanceof Variable) {
// conversations.addAll(context.getAcreAPI().getConversationManager().getAllConversations().values());
// } else {
// conversations.add(context.getAcreAPI().getConversationManager().getConversationByID(((Primitive) cid).value()));
// }
//
// // Check all possible conversations
// for (Conversation conversation : conversations) {
// Map b = Unifier.unify(new Term[] {formula.type()}, new Term[] {Primitive.newPrimitive(conversation)});
// }
// return null;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy