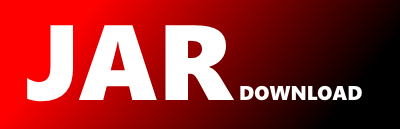
astra.protocol.VickreyAuction.astra Maven / Gradle / Ivy
package astra.protocol;
agent VickreyAuction {
module System system;
module Console console;
module Debug debug;
types auction {
formula created(string, string);
formula available(string, string);
formula attend(string);
formula auction(string);
formula auction(string, funct);
formula auction(string,funct,int);
formula bid(string, int);
}
types auctioneer {
formula participant(string);
formula bid(string, string, int);
formula winner(string, string, int);
}
//***********************************************************************************
// Initiator Rules
//***********************************************************************************
goal +!auction(string id, funct description, config(int timeout)) <: false {
body {
console.println("Starting Auction of: " + id);
foreach(participant(string name)) {
send(cfp, name, auction(id, description, timeout));
}
console.println("Waiting for bids");
system.sleep(timeout);
console.println("Evaluating bids");
int best_bid = -1;
int second_bid = -1;
string winner = "";
foreach (bid(id, string name, int bid)) {
!compare_bids(id, current(name, bid), winner(winner, best_bid), boolean better);
if (better == true) {
second_bid = best_bid;
best_bid = bid;
winner = name;
}
}
if (winner == "") {
console.println("no winner: " + id);
} else {
console.println("winner of: " + id + " is: " + winner + " with bid: " + best_bid + " (paying: "+second_bid+")");
+winner(id, winner, second_bid);
foreach(bid(id, string name, int bid) & (name ~= winner)) {
send(reject-proposal, name, bid(id, bid));
-bid(id, name, bid);
}
send(accept-proposal, winner, bid(id, second_bid));
-bid(id, winner, best_bid);
}
done;
}
rule @message(propose, string sender, bid(id, int price)) {
console.println("received bid: " + price +" from: "+sender);
+bid(id, sender, price);
}
}
rule +!compare_bids(string id, current(string name, int bid), winner(string winner, int best_bid), boolean better) {
if (bid > best_bid) {
better = true;
} else {
better = false;
}
}
//***********************************************************************************
// Participant Rules
//***********************************************************************************
goal +!monitor_for_auctions() <: false {
rule @message(cfp, string sender, auction(string id, funct description, int deadline)) {
+auction(id, description);
!generate_bid(id, description, int bid);
send(propose, sender, bid(id, bid));
}
rule @message(reject-proposal, string sender, bid(string id, int bid)) : auction(id, funct description) {
!failed_bid(id, bid);
-auction(id, description);
}
rule @message(accept-proposal, string sender, bid(string id, int bid)) : auction(id, funct description) {
!successful_bid(id, bid);
-auction(id, description);
}
}
rule +!generate_bid(string id, funct description, int bid) {
bid = 2;
}
rule +!failed_bid(string id, int bid) : auction(id, funct description) {}
rule +!successful_bid(string id, int bid) : auction(id, funct description) {
console.println("I AM WINNER of: " + id + " with bid: " + bid);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy