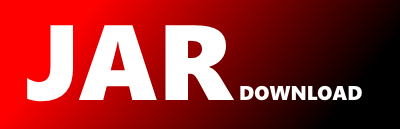
com.atatctech.packages.cache.CacheContainer Maven / Gradle / Ivy
package com.atatctech.packages.cache;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class CacheContainer {
@FunctionalInterface
public interface Filter {
boolean drop(@NotNull CacheKey key, @NotNull Cache> value);
}
protected final Map> cacheMap = new ConcurrentHashMap<>();
protected Filter filter = (key, value) -> false;
public CacheContainer() {
}
synchronized public void abandonOldest(int n) {
List list = cacheMap.keySet().stream().sorted(Comparator.comparing(CacheKey::age)).toList().subList(0, n);
for (CacheKey key : list) cacheMap.remove(key);
}
synchronized public void inspect() {
cacheMap.forEach((k, v) -> {
if (v.hasExpired() || filter.drop(k, v)) cacheMap.remove(k);
});
}
public boolean containsKey(@NotNull CacheKey key) {
return cacheMap.containsKey(key);
}
public boolean containsValue(@NotNull Cache> value) {
return cacheMap.containsValue(value);
}
public boolean contains(@NotNull CacheKey key) {
return containsKey(key);
}
public void put(@NotNull CacheKey key, @NotNull Cache value) {
inspect();
key.rebirth();
cacheMap.put(key, value);
}
public void put(@NotNull Object key, @NotNull Cache value) {
put(new CacheKey(key), value);
}
public @Nullable Cache get(@NotNull CacheKey key) {
inspect();
return cacheMap.get(key);
}
public @Nullable T getCache(@NotNull CacheKey key) {
Cache cache = get(key);
return cache == null ? null : cache.getCache();
}
@Override
public String toString() {
return cacheMap.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy