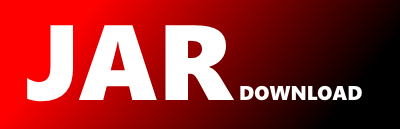
com.athaydes.osgiaas.javac.JavaSnippet Maven / Gradle / Ivy
Show all versions of osgiaas-javac Show documentation
package com.athaydes.osgiaas.javac;
import java.util.Collection;
import static java.util.Collections.emptyList;
/**
* Representation of a Java code snippet.
*
* Instances of types implementing this interface can be used to run
* Java code using the {@link JavacService} implementations.
*
* A trivial implementation of this class is provided with the nested {@link Builder} class.
*/
public interface JavaSnippet {
/**
* @return the executable code. This is the code that will go in the body of the runnable method.
*/
String getExecutableCode();
/**
* @return class imports. Eg. ['java.util.*', 'com.acme']
*/
Collection getImports();
class Builder implements JavaSnippet {
private String code = "";
private Collection imports = emptyList();
private Builder() {
}
public static Builder withCode( String code ) {
Builder b = new Builder();
b.code = code;
return b;
}
public Builder withImports( Collection imports ) {
this.imports = imports;
return this;
}
@Override
public String getExecutableCode() {
return code;
}
@Override
public Collection getImports() {
return imports;
}
}
}