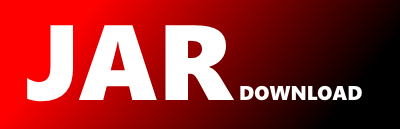
com.atlan.model.assets.ADLSAccount Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 10 18:56:33 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.assets;
import com.atlan.Atlan;
import com.atlan.AtlanClient;
import com.atlan.exception.AtlanException;
import com.atlan.exception.ErrorCode;
import com.atlan.exception.InvalidRequestException;
import com.atlan.exception.NotFoundException;
import com.atlan.model.enums.ADLSAccessTier;
import com.atlan.model.enums.ADLSAccountStatus;
import com.atlan.model.enums.ADLSEncryptionTypes;
import com.atlan.model.enums.ADLSPerformance;
import com.atlan.model.enums.ADLSProvisionState;
import com.atlan.model.enums.ADLSReplicationType;
import com.atlan.model.enums.ADLSStorageKind;
import com.atlan.model.enums.AtlanAnnouncementType;
import com.atlan.model.enums.AtlanConnectorType;
import com.atlan.model.enums.CertificateStatus;
import com.atlan.model.relations.Reference;
import com.atlan.model.relations.UniqueAttributes;
import com.atlan.model.search.FluentSearch;
import com.atlan.model.structs.AzureTag;
import com.atlan.util.StringUtils;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.util.List;
import java.util.Map;
import java.util.SortedSet;
import java.util.concurrent.ThreadLocalRandom;
import javax.annotation.processing.Generated;
import lombok.*;
/**
* Instance of an Azure Data Lake Storage (ADLS) account in Atlan.
*/
@Generated("com.atlan.generators.ModelGeneratorV2")
public class ADLSAccount extends Asset implements IADLSAccount, IADLS, IObjectStore, IAzure, ICatalog, IAsset, IReferenceable, ICloud {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(ADLSAccount.class);
private static final long serialVersionUID = 2L;
public static final String TYPE_NAME = "ADLSAccount";
/**
* Fixed typeName for ADLSAccounts.
*/
String typeName;
/**
* Access tier of this account.
*/
@Attribute
ADLSAccessTier adlsAccountAccessTier;
/**
* Kind of this account.
*/
@Attribute
ADLSStorageKind adlsAccountKind;
/**
* Performance of this account.
*/
@Attribute
ADLSPerformance adlsAccountPerformance;
/**
* Provision state of this account.
*/
@Attribute
ADLSProvisionState adlsAccountProvisionState;
/**
* Unique name of the account for this ADLS asset.
*/
@Attribute
String adlsAccountQualifiedName;
/**
* Replication of this account.
*/
@Attribute
ADLSReplicationType adlsAccountReplication;
/**
* Resource group for this account.
*/
@Attribute
String adlsAccountResourceGroup;
/**
* Secondary location of the ADLS account.
*/
@Attribute
String adlsAccountSecondaryLocation;
/**
* Subscription for this account.
*/
@Attribute
String adlsAccountSubscription;
/**
* Containers that exist within this account.
*/
@Attribute
SortedSet adlsContainers;
/**
* Entity tag for the asset. An entity tag is a hash of the object and represents changes to the contents of an object only, not its metadata.
*/
@Attribute
String adlsETag;
/**
* Type of encryption for this account.
*/
@Attribute
ADLSEncryptionTypes adlsEncryptionType;
/**
* Primary disk state of this account.
*/
@Attribute
ADLSAccountStatus adlsPrimaryDiskState;
/**
* Location of this asset in Azure.
*/
@Attribute
String azureLocation;
/**
* Resource identifier of this asset in Azure.
*/
@Attribute
String azureResourceId;
/**
* Tags that have been applied to this asset in Azure.
*/
@Attribute
List azureTags;
/**
* Tasks to which this asset provides input.
*/
@Attribute
SortedSet inputToAirflowTasks;
/**
* Processes to which this asset provides input.
*/
@Attribute
SortedSet inputToProcesses;
/**
* TBC
*/
@Attribute
SortedSet inputToSparkJobs;
/**
* Tasks from which this asset is output.
*/
@Attribute
SortedSet outputFromAirflowTasks;
/**
* Processes from which this asset is produced as output.
*/
@Attribute
SortedSet outputFromProcesses;
/**
* TBC
*/
@Attribute
SortedSet outputFromSparkJobs;
/**
* Builds the minimal object necessary to create a relationship to a ADLSAccount, from a potentially
* more-complete ADLSAccount object.
*
* @return the minimal object necessary to relate to the ADLSAccount
* @throws InvalidRequestException if any of the minimal set of required properties for a ADLSAccount relationship are not found in the initial object
*/
@Override
public ADLSAccount trimToReference() throws InvalidRequestException {
if (this.getGuid() != null && !this.getGuid().isEmpty()) {
return refByGuid(this.getGuid());
}
if (this.getQualifiedName() != null && !this.getQualifiedName().isEmpty()) {
return refByQualifiedName(this.getQualifiedName());
}
if (this.getUniqueAttributes() != null && this.getUniqueAttributes().getQualifiedName() != null && !this.getUniqueAttributes().getQualifiedName().isEmpty()) {
return refByQualifiedName(this.getUniqueAttributes().getQualifiedName());
}
throw new InvalidRequestException(ErrorCode.MISSING_REQUIRED_RELATIONSHIP_PARAM, TYPE_NAME, "guid, qualifiedName");
}
/**
* Start a fluent search that will return all ADLSAccount assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval. Only active (non-archived) ADLSAccount assets will be included.
*
* @return a fluent search that includes all ADLSAccount assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select() {
return select(Atlan.getDefaultClient());
}
/**
* Start a fluent search that will return all ADLSAccount assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval. Only active (non-archived) ADLSAccount assets will be included.
*
* @param client connectivity to the Atlan tenant from which to retrieve the assets
* @return a fluent search that includes all ADLSAccount assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(AtlanClient client) {
return select(client, false);
}
/**
* Start a fluent search that will return all ADLSAccount assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval.
*
* @param includeArchived when true, archived (soft-deleted) ADLSAccounts will be included
* @return a fluent search that includes all ADLSAccount assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(boolean includeArchived) {
return select(Atlan.getDefaultClient(), includeArchived);
}
/**
* Start a fluent search that will return all ADLSAccount assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval.
*
* @param client connectivity to the Atlan tenant from which to retrieve the assets
* @param includeArchived when true, archived (soft-deleted) ADLSAccounts will be included
* @return a fluent search that includes all ADLSAccount assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(AtlanClient client, boolean includeArchived) {
FluentSearch.FluentSearchBuilder, ?> builder = FluentSearch.builder(client).where(Asset.TYPE_NAME.eq(TYPE_NAME));
if (!includeArchived) {
builder.active();
}
return builder;
}
/**
* Reference to a ADLSAccount by GUID. Use this to create a relationship to this ADLSAccount,
* where the relationship should be replaced.
*
* @param guid the GUID of the ADLSAccount to reference
* @return reference to a ADLSAccount that can be used for defining a relationship to a ADLSAccount
*/
public static ADLSAccount refByGuid(String guid) {
return refByGuid(guid, Reference.SaveSemantic.REPLACE);
}
/**
* Reference to a ADLSAccount by GUID. Use this to create a relationship to this ADLSAccount,
* where you want to further control how that relationship should be updated (i.e. replaced,
* appended, or removed).
*
* @param guid the GUID of the ADLSAccount to reference
* @param semantic how to save this relationship (replace all with this, append it, or remove it)
* @return reference to a ADLSAccount that can be used for defining a relationship to a ADLSAccount
*/
public static ADLSAccount refByGuid(String guid, Reference.SaveSemantic semantic) {
return ADLSAccount._internal().guid(guid).semantic(semantic).build();
}
/**
* Reference to a ADLSAccount by qualifiedName. Use this to create a relationship to this ADLSAccount,
* where the relationship should be replaced.
*
* @param qualifiedName the qualifiedName of the ADLSAccount to reference
* @return reference to a ADLSAccount that can be used for defining a relationship to a ADLSAccount
*/
public static ADLSAccount refByQualifiedName(String qualifiedName) {
return refByQualifiedName(qualifiedName, Reference.SaveSemantic.REPLACE);
}
/**
* Reference to a ADLSAccount by qualifiedName. Use this to create a relationship to this ADLSAccount,
* where you want to further control how that relationship should be updated (i.e. replaced,
* appended, or removed).
*
* @param qualifiedName the qualifiedName of the ADLSAccount to reference
* @param semantic how to save this relationship (replace all with this, append it, or remove it)
* @return reference to a ADLSAccount that can be used for defining a relationship to a ADLSAccount
*/
public static ADLSAccount refByQualifiedName(String qualifiedName, Reference.SaveSemantic semantic) {
return ADLSAccount._internal().uniqueAttributes(UniqueAttributes.builder().qualifiedName(qualifiedName).build()).semantic(semantic).build();
}
/**
* Retrieves a ADLSAccount by one of its identifiers, complete with all of its relationships.
*
* @param id of the ADLSAccount to retrieve, either its GUID or its full qualifiedName
* @return the requested full ADLSAccount, complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the ADLSAccount does not exist or the provided GUID is not a ADLSAccount
*/
@JsonIgnore
public static ADLSAccount get(String id) throws AtlanException {
return get(Atlan.getDefaultClient(), id);
}
/**
* Retrieves a ADLSAccount by one of its identifiers, complete with all of its relationships.
*
* @param client connectivity to the Atlan tenant from which to retrieve the asset
* @param id of the ADLSAccount to retrieve, either its GUID or its full qualifiedName
* @return the requested full ADLSAccount, complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the ADLSAccount does not exist or the provided GUID is not a ADLSAccount
*/
@JsonIgnore
public static ADLSAccount get(AtlanClient client, String id) throws AtlanException {
return get(client, id, true);
}
/**
* Retrieves a ADLSAccount by one of its identifiers, optionally complete with all of its relationships.
*
* @param client connectivity to the Atlan tenant from which to retrieve the asset
* @param id of the ADLSAccount to retrieve, either its GUID or its full qualifiedName
* @param includeRelationships if true, all of the asset's relationships will also be retrieved; if false, no relationships will be retrieved
* @return the requested full ADLSAccount, optionally complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the ADLSAccount does not exist or the provided GUID is not a ADLSAccount
*/
@JsonIgnore
public static ADLSAccount get(AtlanClient client, String id, boolean includeRelationships) throws AtlanException {
if (id == null) {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_GUID, "(null)");
} else if (StringUtils.isUUID(id)) {
Asset asset = Asset.get(client, id, includeRelationships);
if (asset == null) {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_GUID, id);
} else if (asset instanceof ADLSAccount) {
return (ADLSAccount) asset;
} else {
throw new NotFoundException(ErrorCode.ASSET_NOT_TYPE_REQUESTED, id, TYPE_NAME);
}
} else {
Asset asset = Asset.get(client, TYPE_NAME, id, includeRelationships);
if (asset instanceof ADLSAccount) {
return (ADLSAccount) asset;
} else {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_QN, id, TYPE_NAME);
}
}
}
/**
* Restore the archived (soft-deleted) ADLSAccount to active.
*
* @param qualifiedName for the ADLSAccount
* @return true if the ADLSAccount is now active, and false otherwise
* @throws AtlanException on any API problems
*/
public static boolean restore(String qualifiedName) throws AtlanException {
return restore(Atlan.getDefaultClient(), qualifiedName);
}
/**
* Restore the archived (soft-deleted) ADLSAccount to active.
*
* @param client connectivity to the Atlan tenant on which to restore the asset
* @param qualifiedName for the ADLSAccount
* @return true if the ADLSAccount is now active, and false otherwise
* @throws AtlanException on any API problems
*/
public static boolean restore(AtlanClient client, String qualifiedName) throws AtlanException {
return Asset.restore(client, TYPE_NAME, qualifiedName);
}
/**
* Builds the minimal object necessary to create a ADLSAccount.
*
* @param name of the ADLSAccount
* @param connectionQualifiedName unique name of the connection through which the ADLSAccount is accessible
* @return the minimal object necessary to create the ADLSAccount, as a builder
*/
public static ADLSAccountBuilder, ?> creator(String name, String connectionQualifiedName) {
return ADLSAccount._internal().guid("-" + ThreadLocalRandom.current().nextLong(0, Long.MAX_VALUE - 1)).qualifiedName(generateQualifiedName(name, connectionQualifiedName)).name(name).connectionQualifiedName(connectionQualifiedName).connectorType(AtlanConnectorType.ADLS);
}
/**
* Generate a unique ADLSAccount name.
*
* @param name of the ADLSAccount
* @param connectionQualifiedName unique name of the connection through which the ADLSAccount is accessible
* @return a unique name for the ADLSAccount
*/
public static String generateQualifiedName(String name, String connectionQualifiedName) {
return connectionQualifiedName + "/" + name;
}
/**
* Builds the minimal object necessary to update a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the minimal request necessary to update the ADLSAccount, as a builder
*/
public static ADLSAccountBuilder, ?> updater(String qualifiedName, String name) {
return ADLSAccount._internal().guid("-" + ThreadLocalRandom.current().nextLong(0, Long.MAX_VALUE - 1)).qualifiedName(qualifiedName).name(name);
}
/**
* Builds the minimal object necessary to apply an update to a ADLSAccount, from a potentially
* more-complete ADLSAccount object.
*
* @return the minimal object necessary to update the ADLSAccount, as a builder
* @throws InvalidRequestException if any of the minimal set of required properties for ADLSAccount are not found in the initial object
*/
@Override
public ADLSAccountBuilder, ?> trimToRequired() throws InvalidRequestException {
validateRequired(TYPE_NAME, Map.of("qualifiedName", this.getQualifiedName(), "name", this.getName()));
return updater(this.getQualifiedName(), this.getName());
}
/**
* Remove the system description from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeDescription(String qualifiedName, String name) throws AtlanException {
return removeDescription(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the system description from a ADLSAccount.
*
* @param client connectivity to the Atlan tenant on which to remove the asset's description
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeDescription(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (ADLSAccount) Asset.removeDescription(client, updater(qualifiedName, name));
}
/**
* Remove the user's description from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeUserDescription(String qualifiedName, String name) throws AtlanException {
return removeUserDescription(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the user's description from a ADLSAccount.
*
* @param client connectivity to the Atlan tenant on which to remove the asset's description
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeUserDescription(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (ADLSAccount) Asset.removeUserDescription(client, updater(qualifiedName, name));
}
/**
* Remove the owners from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeOwners(String qualifiedName, String name) throws AtlanException {
return removeOwners(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the owners from a ADLSAccount.
*
* @param client connectivity to the Atlan tenant from which to remove the ADLSAccount's owners
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeOwners(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (ADLSAccount) Asset.removeOwners(client, updater(qualifiedName, name));
}
/**
* Update the certificate on a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param certificate to use
* @param message (optional) message, or null if no message
* @return the updated ADLSAccount, or null if the update failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount updateCertificate(String qualifiedName, CertificateStatus certificate, String message) throws AtlanException {
return updateCertificate(Atlan.getDefaultClient(), qualifiedName, certificate, message);
}
/**
* Update the certificate on a ADLSAccount.
*
* @param client connectivity to the Atlan tenant on which to update the ADLSAccount's certificate
* @param qualifiedName of the ADLSAccount
* @param certificate to use
* @param message (optional) message, or null if no message
* @return the updated ADLSAccount, or null if the update failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount updateCertificate(AtlanClient client, String qualifiedName, CertificateStatus certificate, String message) throws AtlanException {
return (ADLSAccount) Asset.updateCertificate(client, _internal(), TYPE_NAME, qualifiedName, certificate, message);
}
/**
* Remove the certificate from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeCertificate(String qualifiedName, String name) throws AtlanException {
return removeCertificate(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the certificate from a ADLSAccount.
*
* @param client connectivity to the Atlan tenant from which to remove the ADLSAccount's certificate
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeCertificate(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (ADLSAccount) Asset.removeCertificate(client, updater(qualifiedName, name));
}
/**
* Update the announcement on a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param type type of announcement to set
* @param title (optional) title of the announcement to set (or null for no title)
* @param message (optional) message of the announcement to set (or null for no message)
* @return the result of the update, or null if the update failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount updateAnnouncement(String qualifiedName, AtlanAnnouncementType type, String title, String message) throws AtlanException {
return updateAnnouncement(Atlan.getDefaultClient(), qualifiedName, type, title, message);
}
/**
* Update the announcement on a ADLSAccount.
*
* @param client connectivity to the Atlan tenant on which to update the ADLSAccount's announcement
* @param qualifiedName of the ADLSAccount
* @param type type of announcement to set
* @param title (optional) title of the announcement to set (or null for no title)
* @param message (optional) message of the announcement to set (or null for no message)
* @return the result of the update, or null if the update failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount updateAnnouncement(AtlanClient client, String qualifiedName, AtlanAnnouncementType type, String title, String message) throws AtlanException {
return (ADLSAccount) Asset.updateAnnouncement(client, _internal(), TYPE_NAME, qualifiedName, type, title, message);
}
/**
* Remove the announcement from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeAnnouncement(String qualifiedName, String name) throws AtlanException {
return removeAnnouncement(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the announcement from a ADLSAccount.
*
* @param client connectivity to the Atlan client from which to remove the ADLSAccount's announcement
* @param qualifiedName of the ADLSAccount
* @param name of the ADLSAccount
* @return the updated ADLSAccount, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeAnnouncement(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (ADLSAccount) Asset.removeAnnouncement(client, updater(qualifiedName, name));
}
/**
* Replace the terms linked to the ADLSAccount.
*
* @param qualifiedName for the ADLSAccount
* @param name human-readable name of the ADLSAccount
* @param terms the list of terms to replace on the ADLSAccount, or null to remove all terms from the ADLSAccount
* @return the ADLSAccount that was updated (note that it will NOT contain details of the replaced terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount replaceTerms(String qualifiedName, String name, List terms) throws AtlanException {
return replaceTerms(Atlan.getDefaultClient(), qualifiedName, name, terms);
}
/**
* Replace the terms linked to the ADLSAccount.
*
* @param client connectivity to the Atlan tenant on which to replace the ADLSAccount's assigned terms
* @param qualifiedName for the ADLSAccount
* @param name human-readable name of the ADLSAccount
* @param terms the list of terms to replace on the ADLSAccount, or null to remove all terms from the ADLSAccount
* @return the ADLSAccount that was updated (note that it will NOT contain details of the replaced terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount replaceTerms(AtlanClient client, String qualifiedName, String name, List terms) throws AtlanException {
return (ADLSAccount) Asset.replaceTerms(client, updater(qualifiedName, name), terms);
}
/**
* Link additional terms to the ADLSAccount, without replacing existing terms linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing terms,
* and a second to append the new terms.
*
* @param qualifiedName for the ADLSAccount
* @param terms the list of terms to append to the ADLSAccount
* @return the ADLSAccount that was updated (note that it will NOT contain details of the appended terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount appendTerms(String qualifiedName, List terms) throws AtlanException {
return appendTerms(Atlan.getDefaultClient(), qualifiedName, terms);
}
/**
* Link additional terms to the ADLSAccount, without replacing existing terms linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing terms,
* and a second to append the new terms.
*
* @param client connectivity to the Atlan tenant on which to append terms to the ADLSAccount
* @param qualifiedName for the ADLSAccount
* @param terms the list of terms to append to the ADLSAccount
* @return the ADLSAccount that was updated (note that it will NOT contain details of the appended terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount appendTerms(AtlanClient client, String qualifiedName, List terms) throws AtlanException {
return (ADLSAccount) Asset.appendTerms(client, TYPE_NAME, qualifiedName, terms);
}
/**
* Remove terms from a ADLSAccount, without replacing all existing terms linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing terms,
* and a second to remove the provided terms.
*
* @param qualifiedName for the ADLSAccount
* @param terms the list of terms to remove from the ADLSAccount, which must be referenced by GUID
* @return the ADLSAccount that was updated (note that it will NOT contain details of the resulting terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeTerms(String qualifiedName, List terms) throws AtlanException {
return removeTerms(Atlan.getDefaultClient(), qualifiedName, terms);
}
/**
* Remove terms from a ADLSAccount, without replacing all existing terms linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing terms,
* and a second to remove the provided terms.
*
* @param client connectivity to the Atlan tenant from which to remove terms from the ADLSAccount
* @param qualifiedName for the ADLSAccount
* @param terms the list of terms to remove from the ADLSAccount, which must be referenced by GUID
* @return the ADLSAccount that was updated (note that it will NOT contain details of the resulting terms)
* @throws AtlanException on any API problems
*/
public static ADLSAccount removeTerms(AtlanClient client, String qualifiedName, List terms) throws AtlanException {
return (ADLSAccount) Asset.removeTerms(client, TYPE_NAME, qualifiedName, terms);
}
/**
* Add Atlan tags to a ADLSAccount, without replacing existing Atlan tags linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param qualifiedName of the ADLSAccount
* @param atlanTagNames human-readable names of the Atlan tags to add
* @throws AtlanException on any API problems
* @return the updated ADLSAccount
*/
public static ADLSAccount appendAtlanTags(String qualifiedName, List atlanTagNames) throws AtlanException {
return appendAtlanTags(Atlan.getDefaultClient(), qualifiedName, atlanTagNames);
}
/**
* Add Atlan tags to a ADLSAccount, without replacing existing Atlan tags linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param client connectivity to the Atlan tenant on which to append Atlan tags to the ADLSAccount
* @param qualifiedName of the ADLSAccount
* @param atlanTagNames human-readable names of the Atlan tags to add
* @throws AtlanException on any API problems
* @return the updated ADLSAccount
*/
public static ADLSAccount appendAtlanTags(AtlanClient client, String qualifiedName, List atlanTagNames) throws AtlanException {
return (ADLSAccount) Asset.appendAtlanTags(client, TYPE_NAME, qualifiedName, atlanTagNames);
}
/**
* Add Atlan tags to a ADLSAccount, without replacing existing Atlan tags linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param qualifiedName of the ADLSAccount
* @param atlanTagNames human-readable names of the Atlan tags to add
* @param propagate whether to propagate the Atlan tag (true) or not (false)
* @param removePropagationsOnDelete whether to remove the propagated Atlan tags when the Atlan tag is removed from this asset (true) or not (false)
* @param restrictLineagePropagation whether to avoid propagating through lineage (true) or do propagate through lineage (false)
* @throws AtlanException on any API problems
* @return the updated ADLSAccount
*/
public static ADLSAccount appendAtlanTags(String qualifiedName, List atlanTagNames, boolean propagate, boolean removePropagationsOnDelete, boolean restrictLineagePropagation) throws AtlanException {
return appendAtlanTags(Atlan.getDefaultClient(), qualifiedName, atlanTagNames, propagate, removePropagationsOnDelete, restrictLineagePropagation);
}
/**
* Add Atlan tags to a ADLSAccount, without replacing existing Atlan tags linked to the ADLSAccount.
* Note: this operation must make two API calls — one to retrieve the ADLSAccount's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param client connectivity to the Atlan tenant on which to append Atlan tags to the ADLSAccount
* @param qualifiedName of the ADLSAccount
* @param atlanTagNames human-readable names of the Atlan tags to add
* @param propagate whether to propagate the Atlan tag (true) or not (false)
* @param removePropagationsOnDelete whether to remove the propagated Atlan tags when the Atlan tag is removed from this asset (true) or not (false)
* @param restrictLineagePropagation whether to avoid propagating through lineage (true) or do propagate through lineage (false)
* @throws AtlanException on any API problems
* @return the updated ADLSAccount
*/
public static ADLSAccount appendAtlanTags(AtlanClient client, String qualifiedName, List atlanTagNames, boolean propagate, boolean removePropagationsOnDelete, boolean restrictLineagePropagation) throws AtlanException {
return (ADLSAccount) Asset.appendAtlanTags(client, TYPE_NAME, qualifiedName, atlanTagNames, propagate, removePropagationsOnDelete, restrictLineagePropagation);
}
/**
* Remove an Atlan tag from a ADLSAccount.
*
* @param qualifiedName of the ADLSAccount
* @param atlanTagName human-readable name of the Atlan tag to remove
* @throws AtlanException on any API problems, or if the Atlan tag does not exist on the ADLSAccount
*/
public static void removeAtlanTag(String qualifiedName, String atlanTagName) throws AtlanException {
removeAtlanTag(Atlan.getDefaultClient(), qualifiedName, atlanTagName);
}
/**
* Remove an Atlan tag from a ADLSAccount.
*
* @param client connectivity to the Atlan tenant from which to remove an Atlan tag from a ADLSAccount
* @param qualifiedName of the ADLSAccount
* @param atlanTagName human-readable name of the Atlan tag to remove
* @throws AtlanException on any API problems, or if the Atlan tag does not exist on the ADLSAccount
*/
public static void removeAtlanTag(AtlanClient client, String qualifiedName, String atlanTagName) throws AtlanException {
Asset.removeAtlanTag(client, TYPE_NAME, qualifiedName, atlanTagName);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static String $default$typeName() {
return TYPE_NAME;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class ADLSAccountBuilder> extends Asset.AssetBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private boolean typeName$set;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String typeName$value;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSAccessTier adlsAccountAccessTier;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSStorageKind adlsAccountKind;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSPerformance adlsAccountPerformance;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSProvisionState adlsAccountProvisionState;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String adlsAccountQualifiedName;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSReplicationType adlsAccountReplication;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String adlsAccountResourceGroup;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String adlsAccountSecondaryLocation;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String adlsAccountSubscription;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList adlsContainers;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String adlsETag;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSEncryptionTypes adlsEncryptionType;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSAccountStatus adlsPrimaryDiskState;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String azureLocation;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String azureResourceId;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList azureTags;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList inputToAirflowTasks;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList inputToProcesses;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList inputToSparkJobs;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList outputFromAirflowTasks;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList outputFromProcesses;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList outputFromSparkJobs;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected B $fillValuesFrom(final C instance) {
super.$fillValuesFrom(instance);
ADLSAccount.ADLSAccountBuilder.$fillValuesFromInstanceIntoBuilder(instance, this);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static void $fillValuesFromInstanceIntoBuilder(final ADLSAccount instance, final ADLSAccount.ADLSAccountBuilder, ?> b) {
b.typeName(instance.typeName);
b.adlsAccountAccessTier(instance.adlsAccountAccessTier);
b.adlsAccountKind(instance.adlsAccountKind);
b.adlsAccountPerformance(instance.adlsAccountPerformance);
b.adlsAccountProvisionState(instance.adlsAccountProvisionState);
b.adlsAccountQualifiedName(instance.adlsAccountQualifiedName);
b.adlsAccountReplication(instance.adlsAccountReplication);
b.adlsAccountResourceGroup(instance.adlsAccountResourceGroup);
b.adlsAccountSecondaryLocation(instance.adlsAccountSecondaryLocation);
b.adlsAccountSubscription(instance.adlsAccountSubscription);
b.adlsContainers(instance.adlsContainers == null ? java.util.Collections.emptySortedSet() : instance.adlsContainers);
b.adlsETag(instance.adlsETag);
b.adlsEncryptionType(instance.adlsEncryptionType);
b.adlsPrimaryDiskState(instance.adlsPrimaryDiskState);
b.azureLocation(instance.azureLocation);
b.azureResourceId(instance.azureResourceId);
b.azureTags(instance.azureTags == null ? java.util.Collections.emptyList() : instance.azureTags);
b.inputToAirflowTasks(instance.inputToAirflowTasks == null ? java.util.Collections.emptySortedSet() : instance.inputToAirflowTasks);
b.inputToProcesses(instance.inputToProcesses == null ? java.util.Collections.emptySortedSet() : instance.inputToProcesses);
b.inputToSparkJobs(instance.inputToSparkJobs == null ? java.util.Collections.emptySortedSet() : instance.inputToSparkJobs);
b.outputFromAirflowTasks(instance.outputFromAirflowTasks == null ? java.util.Collections.emptySortedSet() : instance.outputFromAirflowTasks);
b.outputFromProcesses(instance.outputFromProcesses == null ? java.util.Collections.emptySortedSet() : instance.outputFromProcesses);
b.outputFromSparkJobs(instance.outputFromSparkJobs == null ? java.util.Collections.emptySortedSet() : instance.outputFromSparkJobs);
}
/**
* Fixed typeName for ADLSAccounts.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B typeName(final String typeName) {
this.typeName$value = typeName;
typeName$set = true;
return self();
}
/**
* Access tier of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountAccessTier(final ADLSAccessTier adlsAccountAccessTier) {
this.adlsAccountAccessTier = adlsAccountAccessTier;
return self();
}
/**
* Kind of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountKind(final ADLSStorageKind adlsAccountKind) {
this.adlsAccountKind = adlsAccountKind;
return self();
}
/**
* Performance of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountPerformance(final ADLSPerformance adlsAccountPerformance) {
this.adlsAccountPerformance = adlsAccountPerformance;
return self();
}
/**
* Provision state of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountProvisionState(final ADLSProvisionState adlsAccountProvisionState) {
this.adlsAccountProvisionState = adlsAccountProvisionState;
return self();
}
/**
* Unique name of the account for this ADLS asset.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountQualifiedName(final String adlsAccountQualifiedName) {
this.adlsAccountQualifiedName = adlsAccountQualifiedName;
return self();
}
/**
* Replication of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountReplication(final ADLSReplicationType adlsAccountReplication) {
this.adlsAccountReplication = adlsAccountReplication;
return self();
}
/**
* Resource group for this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountResourceGroup(final String adlsAccountResourceGroup) {
this.adlsAccountResourceGroup = adlsAccountResourceGroup;
return self();
}
/**
* Secondary location of the ADLS account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountSecondaryLocation(final String adlsAccountSecondaryLocation) {
this.adlsAccountSecondaryLocation = adlsAccountSecondaryLocation;
return self();
}
/**
* Subscription for this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsAccountSubscription(final String adlsAccountSubscription) {
this.adlsAccountSubscription = adlsAccountSubscription;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsContainer(final IADLSContainer adlsContainer) {
if (this.adlsContainers == null) this.adlsContainers = new java.util.ArrayList();
this.adlsContainers.add(adlsContainer);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsContainers(final java.util.Collection extends IADLSContainer> adlsContainers) {
if (adlsContainers == null) {
throw new java.lang.NullPointerException("adlsContainers cannot be null");
}
if (this.adlsContainers == null) this.adlsContainers = new java.util.ArrayList();
this.adlsContainers.addAll(adlsContainers);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearAdlsContainers() {
if (this.adlsContainers != null) this.adlsContainers.clear();
return self();
}
/**
* Entity tag for the asset. An entity tag is a hash of the object and represents changes to the contents of an object only, not its metadata.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsETag(final String adlsETag) {
this.adlsETag = adlsETag;
return self();
}
/**
* Type of encryption for this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsEncryptionType(final ADLSEncryptionTypes adlsEncryptionType) {
this.adlsEncryptionType = adlsEncryptionType;
return self();
}
/**
* Primary disk state of this account.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B adlsPrimaryDiskState(final ADLSAccountStatus adlsPrimaryDiskState) {
this.adlsPrimaryDiskState = adlsPrimaryDiskState;
return self();
}
/**
* Location of this asset in Azure.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B azureLocation(final String azureLocation) {
this.azureLocation = azureLocation;
return self();
}
/**
* Resource identifier of this asset in Azure.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B azureResourceId(final String azureResourceId) {
this.azureResourceId = azureResourceId;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B azureTag(final AzureTag azureTag) {
if (this.azureTags == null) this.azureTags = new java.util.ArrayList();
this.azureTags.add(azureTag);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B azureTags(final java.util.Collection extends AzureTag> azureTags) {
if (azureTags == null) {
throw new java.lang.NullPointerException("azureTags cannot be null");
}
if (this.azureTags == null) this.azureTags = new java.util.ArrayList();
this.azureTags.addAll(azureTags);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearAzureTags() {
if (this.azureTags != null) this.azureTags.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToAirflowTask(final IAirflowTask inputToAirflowTask) {
if (this.inputToAirflowTasks == null) this.inputToAirflowTasks = new java.util.ArrayList();
this.inputToAirflowTasks.add(inputToAirflowTask);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToAirflowTasks(final java.util.Collection extends IAirflowTask> inputToAirflowTasks) {
if (inputToAirflowTasks == null) {
throw new java.lang.NullPointerException("inputToAirflowTasks cannot be null");
}
if (this.inputToAirflowTasks == null) this.inputToAirflowTasks = new java.util.ArrayList();
this.inputToAirflowTasks.addAll(inputToAirflowTasks);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearInputToAirflowTasks() {
if (this.inputToAirflowTasks != null) this.inputToAirflowTasks.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToProcess(final ILineageProcess inputToProcess) {
if (this.inputToProcesses == null) this.inputToProcesses = new java.util.ArrayList();
this.inputToProcesses.add(inputToProcess);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToProcesses(final java.util.Collection extends ILineageProcess> inputToProcesses) {
if (inputToProcesses == null) {
throw new java.lang.NullPointerException("inputToProcesses cannot be null");
}
if (this.inputToProcesses == null) this.inputToProcesses = new java.util.ArrayList();
this.inputToProcesses.addAll(inputToProcesses);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearInputToProcesses() {
if (this.inputToProcesses != null) this.inputToProcesses.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToSparkJob(final ISparkJob inputToSparkJob) {
if (this.inputToSparkJobs == null) this.inputToSparkJobs = new java.util.ArrayList();
this.inputToSparkJobs.add(inputToSparkJob);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B inputToSparkJobs(final java.util.Collection extends ISparkJob> inputToSparkJobs) {
if (inputToSparkJobs == null) {
throw new java.lang.NullPointerException("inputToSparkJobs cannot be null");
}
if (this.inputToSparkJobs == null) this.inputToSparkJobs = new java.util.ArrayList();
this.inputToSparkJobs.addAll(inputToSparkJobs);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearInputToSparkJobs() {
if (this.inputToSparkJobs != null) this.inputToSparkJobs.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromAirflowTask(final IAirflowTask outputFromAirflowTask) {
if (this.outputFromAirflowTasks == null) this.outputFromAirflowTasks = new java.util.ArrayList();
this.outputFromAirflowTasks.add(outputFromAirflowTask);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromAirflowTasks(final java.util.Collection extends IAirflowTask> outputFromAirflowTasks) {
if (outputFromAirflowTasks == null) {
throw new java.lang.NullPointerException("outputFromAirflowTasks cannot be null");
}
if (this.outputFromAirflowTasks == null) this.outputFromAirflowTasks = new java.util.ArrayList();
this.outputFromAirflowTasks.addAll(outputFromAirflowTasks);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearOutputFromAirflowTasks() {
if (this.outputFromAirflowTasks != null) this.outputFromAirflowTasks.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromProcess(final ILineageProcess outputFromProcess) {
if (this.outputFromProcesses == null) this.outputFromProcesses = new java.util.ArrayList();
this.outputFromProcesses.add(outputFromProcess);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromProcesses(final java.util.Collection extends ILineageProcess> outputFromProcesses) {
if (outputFromProcesses == null) {
throw new java.lang.NullPointerException("outputFromProcesses cannot be null");
}
if (this.outputFromProcesses == null) this.outputFromProcesses = new java.util.ArrayList();
this.outputFromProcesses.addAll(outputFromProcesses);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearOutputFromProcesses() {
if (this.outputFromProcesses != null) this.outputFromProcesses.clear();
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromSparkJob(final ISparkJob outputFromSparkJob) {
if (this.outputFromSparkJobs == null) this.outputFromSparkJobs = new java.util.ArrayList();
this.outputFromSparkJobs.add(outputFromSparkJob);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B outputFromSparkJobs(final java.util.Collection extends ISparkJob> outputFromSparkJobs) {
if (outputFromSparkJobs == null) {
throw new java.lang.NullPointerException("outputFromSparkJobs cannot be null");
}
if (this.outputFromSparkJobs == null) this.outputFromSparkJobs = new java.util.ArrayList();
this.outputFromSparkJobs.addAll(outputFromSparkJobs);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearOutputFromSparkJobs() {
if (this.outputFromSparkJobs != null) this.outputFromSparkJobs.clear();
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "ADLSAccount.ADLSAccountBuilder(super=" + super.toString() + ", typeName$value=" + this.typeName$value + ", adlsAccountAccessTier=" + this.adlsAccountAccessTier + ", adlsAccountKind=" + this.adlsAccountKind + ", adlsAccountPerformance=" + this.adlsAccountPerformance + ", adlsAccountProvisionState=" + this.adlsAccountProvisionState + ", adlsAccountQualifiedName=" + this.adlsAccountQualifiedName + ", adlsAccountReplication=" + this.adlsAccountReplication + ", adlsAccountResourceGroup=" + this.adlsAccountResourceGroup + ", adlsAccountSecondaryLocation=" + this.adlsAccountSecondaryLocation + ", adlsAccountSubscription=" + this.adlsAccountSubscription + ", adlsContainers=" + this.adlsContainers + ", adlsETag=" + this.adlsETag + ", adlsEncryptionType=" + this.adlsEncryptionType + ", adlsPrimaryDiskState=" + this.adlsPrimaryDiskState + ", azureLocation=" + this.azureLocation + ", azureResourceId=" + this.azureResourceId + ", azureTags=" + this.azureTags + ", inputToAirflowTasks=" + this.inputToAirflowTasks + ", inputToProcesses=" + this.inputToProcesses + ", inputToSparkJobs=" + this.inputToSparkJobs + ", outputFromAirflowTasks=" + this.outputFromAirflowTasks + ", outputFromProcesses=" + this.outputFromProcesses + ", outputFromSparkJobs=" + this.outputFromSparkJobs + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class ADLSAccountBuilderImpl extends ADLSAccount.ADLSAccountBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ADLSAccountBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected ADLSAccount.ADLSAccountBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSAccount build() {
return new ADLSAccount(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected ADLSAccount(final ADLSAccount.ADLSAccountBuilder, ?> b) {
super(b);
if (b.typeName$set) this.typeName = b.typeName$value;
else this.typeName = ADLSAccount.$default$typeName();
this.adlsAccountAccessTier = b.adlsAccountAccessTier;
this.adlsAccountKind = b.adlsAccountKind;
this.adlsAccountPerformance = b.adlsAccountPerformance;
this.adlsAccountProvisionState = b.adlsAccountProvisionState;
this.adlsAccountQualifiedName = b.adlsAccountQualifiedName;
this.adlsAccountReplication = b.adlsAccountReplication;
this.adlsAccountResourceGroup = b.adlsAccountResourceGroup;
this.adlsAccountSecondaryLocation = b.adlsAccountSecondaryLocation;
this.adlsAccountSubscription = b.adlsAccountSubscription;
java.util.SortedSet adlsContainers = new java.util.TreeSet();
if (b.adlsContainers != null) adlsContainers.addAll(b.adlsContainers);
adlsContainers = java.util.Collections.unmodifiableSortedSet(adlsContainers);
this.adlsContainers = adlsContainers;
this.adlsETag = b.adlsETag;
this.adlsEncryptionType = b.adlsEncryptionType;
this.adlsPrimaryDiskState = b.adlsPrimaryDiskState;
this.azureLocation = b.azureLocation;
this.azureResourceId = b.azureResourceId;
java.util.List azureTags;
switch (b.azureTags == null ? 0 : b.azureTags.size()) {
case 0:
azureTags = java.util.Collections.emptyList();
break;
case 1:
azureTags = java.util.Collections.singletonList(b.azureTags.get(0));
break;
default:
azureTags = java.util.Collections.unmodifiableList(new java.util.ArrayList(b.azureTags));
}
this.azureTags = azureTags;
java.util.SortedSet inputToAirflowTasks = new java.util.TreeSet();
if (b.inputToAirflowTasks != null) inputToAirflowTasks.addAll(b.inputToAirflowTasks);
inputToAirflowTasks = java.util.Collections.unmodifiableSortedSet(inputToAirflowTasks);
this.inputToAirflowTasks = inputToAirflowTasks;
java.util.SortedSet inputToProcesses = new java.util.TreeSet();
if (b.inputToProcesses != null) inputToProcesses.addAll(b.inputToProcesses);
inputToProcesses = java.util.Collections.unmodifiableSortedSet(inputToProcesses);
this.inputToProcesses = inputToProcesses;
java.util.SortedSet inputToSparkJobs = new java.util.TreeSet();
if (b.inputToSparkJobs != null) inputToSparkJobs.addAll(b.inputToSparkJobs);
inputToSparkJobs = java.util.Collections.unmodifiableSortedSet(inputToSparkJobs);
this.inputToSparkJobs = inputToSparkJobs;
java.util.SortedSet outputFromAirflowTasks = new java.util.TreeSet();
if (b.outputFromAirflowTasks != null) outputFromAirflowTasks.addAll(b.outputFromAirflowTasks);
outputFromAirflowTasks = java.util.Collections.unmodifiableSortedSet(outputFromAirflowTasks);
this.outputFromAirflowTasks = outputFromAirflowTasks;
java.util.SortedSet outputFromProcesses = new java.util.TreeSet();
if (b.outputFromProcesses != null) outputFromProcesses.addAll(b.outputFromProcesses);
outputFromProcesses = java.util.Collections.unmodifiableSortedSet(outputFromProcesses);
this.outputFromProcesses = outputFromProcesses;
java.util.SortedSet outputFromSparkJobs = new java.util.TreeSet();
if (b.outputFromSparkJobs != null) outputFromSparkJobs.addAll(b.outputFromSparkJobs);
outputFromSparkJobs = java.util.Collections.unmodifiableSortedSet(outputFromSparkJobs);
this.outputFromSparkJobs = outputFromSparkJobs;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static ADLSAccount.ADLSAccountBuilder, ?> _internal() {
return new ADLSAccount.ADLSAccountBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSAccount.ADLSAccountBuilder, ?> toBuilder() {
return new ADLSAccount.ADLSAccountBuilderImpl().$fillValuesFrom(this);
}
/**
* Access tier of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSAccessTier getAdlsAccountAccessTier() {
return this.adlsAccountAccessTier;
}
/**
* Kind of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSStorageKind getAdlsAccountKind() {
return this.adlsAccountKind;
}
/**
* Performance of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSPerformance getAdlsAccountPerformance() {
return this.adlsAccountPerformance;
}
/**
* Provision state of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSProvisionState getAdlsAccountProvisionState() {
return this.adlsAccountProvisionState;
}
/**
* Unique name of the account for this ADLS asset.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAdlsAccountQualifiedName() {
return this.adlsAccountQualifiedName;
}
/**
* Replication of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSReplicationType getAdlsAccountReplication() {
return this.adlsAccountReplication;
}
/**
* Resource group for this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAdlsAccountResourceGroup() {
return this.adlsAccountResourceGroup;
}
/**
* Secondary location of the ADLS account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAdlsAccountSecondaryLocation() {
return this.adlsAccountSecondaryLocation;
}
/**
* Subscription for this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAdlsAccountSubscription() {
return this.adlsAccountSubscription;
}
/**
* Containers that exist within this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getAdlsContainers() {
return this.adlsContainers;
}
/**
* Entity tag for the asset. An entity tag is a hash of the object and represents changes to the contents of an object only, not its metadata.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAdlsETag() {
return this.adlsETag;
}
/**
* Type of encryption for this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSEncryptionTypes getAdlsEncryptionType() {
return this.adlsEncryptionType;
}
/**
* Primary disk state of this account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ADLSAccountStatus getAdlsPrimaryDiskState() {
return this.adlsPrimaryDiskState;
}
/**
* Location of this asset in Azure.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAzureLocation() {
return this.azureLocation;
}
/**
* Resource identifier of this asset in Azure.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAzureResourceId() {
return this.azureResourceId;
}
/**
* Tags that have been applied to this asset in Azure.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAzureTags() {
return this.azureTags;
}
/**
* Tasks to which this asset provides input.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getInputToAirflowTasks() {
return this.inputToAirflowTasks;
}
/**
* Processes to which this asset provides input.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getInputToProcesses() {
return this.inputToProcesses;
}
/**
* TBC
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getInputToSparkJobs() {
return this.inputToSparkJobs;
}
/**
* Tasks from which this asset is output.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getOutputFromAirflowTasks() {
return this.outputFromAirflowTasks;
}
/**
* Processes from which this asset is produced as output.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getOutputFromProcesses() {
return this.outputFromProcesses;
}
/**
* TBC
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getOutputFromSparkJobs() {
return this.outputFromSparkJobs;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ADLSAccount)) return false;
final ADLSAccount other = (ADLSAccount) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$typeName = this.getTypeName();
final java.lang.Object other$typeName = other.getTypeName();
if (this$typeName == null ? other$typeName != null : !this$typeName.equals(other$typeName)) return false;
final java.lang.Object this$adlsAccountAccessTier = this.getAdlsAccountAccessTier();
final java.lang.Object other$adlsAccountAccessTier = other.getAdlsAccountAccessTier();
if (this$adlsAccountAccessTier == null ? other$adlsAccountAccessTier != null : !this$adlsAccountAccessTier.equals(other$adlsAccountAccessTier)) return false;
final java.lang.Object this$adlsAccountKind = this.getAdlsAccountKind();
final java.lang.Object other$adlsAccountKind = other.getAdlsAccountKind();
if (this$adlsAccountKind == null ? other$adlsAccountKind != null : !this$adlsAccountKind.equals(other$adlsAccountKind)) return false;
final java.lang.Object this$adlsAccountPerformance = this.getAdlsAccountPerformance();
final java.lang.Object other$adlsAccountPerformance = other.getAdlsAccountPerformance();
if (this$adlsAccountPerformance == null ? other$adlsAccountPerformance != null : !this$adlsAccountPerformance.equals(other$adlsAccountPerformance)) return false;
final java.lang.Object this$adlsAccountProvisionState = this.getAdlsAccountProvisionState();
final java.lang.Object other$adlsAccountProvisionState = other.getAdlsAccountProvisionState();
if (this$adlsAccountProvisionState == null ? other$adlsAccountProvisionState != null : !this$adlsAccountProvisionState.equals(other$adlsAccountProvisionState)) return false;
final java.lang.Object this$adlsAccountQualifiedName = this.getAdlsAccountQualifiedName();
final java.lang.Object other$adlsAccountQualifiedName = other.getAdlsAccountQualifiedName();
if (this$adlsAccountQualifiedName == null ? other$adlsAccountQualifiedName != null : !this$adlsAccountQualifiedName.equals(other$adlsAccountQualifiedName)) return false;
final java.lang.Object this$adlsAccountReplication = this.getAdlsAccountReplication();
final java.lang.Object other$adlsAccountReplication = other.getAdlsAccountReplication();
if (this$adlsAccountReplication == null ? other$adlsAccountReplication != null : !this$adlsAccountReplication.equals(other$adlsAccountReplication)) return false;
final java.lang.Object this$adlsAccountResourceGroup = this.getAdlsAccountResourceGroup();
final java.lang.Object other$adlsAccountResourceGroup = other.getAdlsAccountResourceGroup();
if (this$adlsAccountResourceGroup == null ? other$adlsAccountResourceGroup != null : !this$adlsAccountResourceGroup.equals(other$adlsAccountResourceGroup)) return false;
final java.lang.Object this$adlsAccountSecondaryLocation = this.getAdlsAccountSecondaryLocation();
final java.lang.Object other$adlsAccountSecondaryLocation = other.getAdlsAccountSecondaryLocation();
if (this$adlsAccountSecondaryLocation == null ? other$adlsAccountSecondaryLocation != null : !this$adlsAccountSecondaryLocation.equals(other$adlsAccountSecondaryLocation)) return false;
final java.lang.Object this$adlsAccountSubscription = this.getAdlsAccountSubscription();
final java.lang.Object other$adlsAccountSubscription = other.getAdlsAccountSubscription();
if (this$adlsAccountSubscription == null ? other$adlsAccountSubscription != null : !this$adlsAccountSubscription.equals(other$adlsAccountSubscription)) return false;
final java.lang.Object this$adlsContainers = this.getAdlsContainers();
final java.lang.Object other$adlsContainers = other.getAdlsContainers();
if (this$adlsContainers == null ? other$adlsContainers != null : !this$adlsContainers.equals(other$adlsContainers)) return false;
final java.lang.Object this$adlsETag = this.getAdlsETag();
final java.lang.Object other$adlsETag = other.getAdlsETag();
if (this$adlsETag == null ? other$adlsETag != null : !this$adlsETag.equals(other$adlsETag)) return false;
final java.lang.Object this$adlsEncryptionType = this.getAdlsEncryptionType();
final java.lang.Object other$adlsEncryptionType = other.getAdlsEncryptionType();
if (this$adlsEncryptionType == null ? other$adlsEncryptionType != null : !this$adlsEncryptionType.equals(other$adlsEncryptionType)) return false;
final java.lang.Object this$adlsPrimaryDiskState = this.getAdlsPrimaryDiskState();
final java.lang.Object other$adlsPrimaryDiskState = other.getAdlsPrimaryDiskState();
if (this$adlsPrimaryDiskState == null ? other$adlsPrimaryDiskState != null : !this$adlsPrimaryDiskState.equals(other$adlsPrimaryDiskState)) return false;
final java.lang.Object this$azureLocation = this.getAzureLocation();
final java.lang.Object other$azureLocation = other.getAzureLocation();
if (this$azureLocation == null ? other$azureLocation != null : !this$azureLocation.equals(other$azureLocation)) return false;
final java.lang.Object this$azureResourceId = this.getAzureResourceId();
final java.lang.Object other$azureResourceId = other.getAzureResourceId();
if (this$azureResourceId == null ? other$azureResourceId != null : !this$azureResourceId.equals(other$azureResourceId)) return false;
final java.lang.Object this$azureTags = this.getAzureTags();
final java.lang.Object other$azureTags = other.getAzureTags();
if (this$azureTags == null ? other$azureTags != null : !this$azureTags.equals(other$azureTags)) return false;
final java.lang.Object this$inputToAirflowTasks = this.getInputToAirflowTasks();
final java.lang.Object other$inputToAirflowTasks = other.getInputToAirflowTasks();
if (this$inputToAirflowTasks == null ? other$inputToAirflowTasks != null : !this$inputToAirflowTasks.equals(other$inputToAirflowTasks)) return false;
final java.lang.Object this$inputToProcesses = this.getInputToProcesses();
final java.lang.Object other$inputToProcesses = other.getInputToProcesses();
if (this$inputToProcesses == null ? other$inputToProcesses != null : !this$inputToProcesses.equals(other$inputToProcesses)) return false;
final java.lang.Object this$inputToSparkJobs = this.getInputToSparkJobs();
final java.lang.Object other$inputToSparkJobs = other.getInputToSparkJobs();
if (this$inputToSparkJobs == null ? other$inputToSparkJobs != null : !this$inputToSparkJobs.equals(other$inputToSparkJobs)) return false;
final java.lang.Object this$outputFromAirflowTasks = this.getOutputFromAirflowTasks();
final java.lang.Object other$outputFromAirflowTasks = other.getOutputFromAirflowTasks();
if (this$outputFromAirflowTasks == null ? other$outputFromAirflowTasks != null : !this$outputFromAirflowTasks.equals(other$outputFromAirflowTasks)) return false;
final java.lang.Object this$outputFromProcesses = this.getOutputFromProcesses();
final java.lang.Object other$outputFromProcesses = other.getOutputFromProcesses();
if (this$outputFromProcesses == null ? other$outputFromProcesses != null : !this$outputFromProcesses.equals(other$outputFromProcesses)) return false;
final java.lang.Object this$outputFromSparkJobs = this.getOutputFromSparkJobs();
final java.lang.Object other$outputFromSparkJobs = other.getOutputFromSparkJobs();
if (this$outputFromSparkJobs == null ? other$outputFromSparkJobs != null : !this$outputFromSparkJobs.equals(other$outputFromSparkJobs)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ADLSAccount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final java.lang.Object $typeName = this.getTypeName();
result = result * PRIME + ($typeName == null ? 43 : $typeName.hashCode());
final java.lang.Object $adlsAccountAccessTier = this.getAdlsAccountAccessTier();
result = result * PRIME + ($adlsAccountAccessTier == null ? 43 : $adlsAccountAccessTier.hashCode());
final java.lang.Object $adlsAccountKind = this.getAdlsAccountKind();
result = result * PRIME + ($adlsAccountKind == null ? 43 : $adlsAccountKind.hashCode());
final java.lang.Object $adlsAccountPerformance = this.getAdlsAccountPerformance();
result = result * PRIME + ($adlsAccountPerformance == null ? 43 : $adlsAccountPerformance.hashCode());
final java.lang.Object $adlsAccountProvisionState = this.getAdlsAccountProvisionState();
result = result * PRIME + ($adlsAccountProvisionState == null ? 43 : $adlsAccountProvisionState.hashCode());
final java.lang.Object $adlsAccountQualifiedName = this.getAdlsAccountQualifiedName();
result = result * PRIME + ($adlsAccountQualifiedName == null ? 43 : $adlsAccountQualifiedName.hashCode());
final java.lang.Object $adlsAccountReplication = this.getAdlsAccountReplication();
result = result * PRIME + ($adlsAccountReplication == null ? 43 : $adlsAccountReplication.hashCode());
final java.lang.Object $adlsAccountResourceGroup = this.getAdlsAccountResourceGroup();
result = result * PRIME + ($adlsAccountResourceGroup == null ? 43 : $adlsAccountResourceGroup.hashCode());
final java.lang.Object $adlsAccountSecondaryLocation = this.getAdlsAccountSecondaryLocation();
result = result * PRIME + ($adlsAccountSecondaryLocation == null ? 43 : $adlsAccountSecondaryLocation.hashCode());
final java.lang.Object $adlsAccountSubscription = this.getAdlsAccountSubscription();
result = result * PRIME + ($adlsAccountSubscription == null ? 43 : $adlsAccountSubscription.hashCode());
final java.lang.Object $adlsContainers = this.getAdlsContainers();
result = result * PRIME + ($adlsContainers == null ? 43 : $adlsContainers.hashCode());
final java.lang.Object $adlsETag = this.getAdlsETag();
result = result * PRIME + ($adlsETag == null ? 43 : $adlsETag.hashCode());
final java.lang.Object $adlsEncryptionType = this.getAdlsEncryptionType();
result = result * PRIME + ($adlsEncryptionType == null ? 43 : $adlsEncryptionType.hashCode());
final java.lang.Object $adlsPrimaryDiskState = this.getAdlsPrimaryDiskState();
result = result * PRIME + ($adlsPrimaryDiskState == null ? 43 : $adlsPrimaryDiskState.hashCode());
final java.lang.Object $azureLocation = this.getAzureLocation();
result = result * PRIME + ($azureLocation == null ? 43 : $azureLocation.hashCode());
final java.lang.Object $azureResourceId = this.getAzureResourceId();
result = result * PRIME + ($azureResourceId == null ? 43 : $azureResourceId.hashCode());
final java.lang.Object $azureTags = this.getAzureTags();
result = result * PRIME + ($azureTags == null ? 43 : $azureTags.hashCode());
final java.lang.Object $inputToAirflowTasks = this.getInputToAirflowTasks();
result = result * PRIME + ($inputToAirflowTasks == null ? 43 : $inputToAirflowTasks.hashCode());
final java.lang.Object $inputToProcesses = this.getInputToProcesses();
result = result * PRIME + ($inputToProcesses == null ? 43 : $inputToProcesses.hashCode());
final java.lang.Object $inputToSparkJobs = this.getInputToSparkJobs();
result = result * PRIME + ($inputToSparkJobs == null ? 43 : $inputToSparkJobs.hashCode());
final java.lang.Object $outputFromAirflowTasks = this.getOutputFromAirflowTasks();
result = result * PRIME + ($outputFromAirflowTasks == null ? 43 : $outputFromAirflowTasks.hashCode());
final java.lang.Object $outputFromProcesses = this.getOutputFromProcesses();
result = result * PRIME + ($outputFromProcesses == null ? 43 : $outputFromProcesses.hashCode());
final java.lang.Object $outputFromSparkJobs = this.getOutputFromSparkJobs();
result = result * PRIME + ($outputFromSparkJobs == null ? 43 : $outputFromSparkJobs.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "ADLSAccount(super=" + super.toString() + ", typeName=" + this.getTypeName() + ", adlsAccountAccessTier=" + this.getAdlsAccountAccessTier() + ", adlsAccountKind=" + this.getAdlsAccountKind() + ", adlsAccountPerformance=" + this.getAdlsAccountPerformance() + ", adlsAccountProvisionState=" + this.getAdlsAccountProvisionState() + ", adlsAccountQualifiedName=" + this.getAdlsAccountQualifiedName() + ", adlsAccountReplication=" + this.getAdlsAccountReplication() + ", adlsAccountResourceGroup=" + this.getAdlsAccountResourceGroup() + ", adlsAccountSecondaryLocation=" + this.getAdlsAccountSecondaryLocation() + ", adlsAccountSubscription=" + this.getAdlsAccountSubscription() + ", adlsContainers=" + this.getAdlsContainers() + ", adlsETag=" + this.getAdlsETag() + ", adlsEncryptionType=" + this.getAdlsEncryptionType() + ", adlsPrimaryDiskState=" + this.getAdlsPrimaryDiskState() + ", azureLocation=" + this.getAzureLocation() + ", azureResourceId=" + this.getAzureResourceId() + ", azureTags=" + this.getAzureTags() + ", inputToAirflowTasks=" + this.getInputToAirflowTasks() + ", inputToProcesses=" + this.getInputToProcesses() + ", inputToSparkJobs=" + this.getInputToSparkJobs() + ", outputFromAirflowTasks=" + this.getOutputFromAirflowTasks() + ", outputFromProcesses=" + this.getOutputFromProcesses() + ", outputFromSparkJobs=" + this.getOutputFromSparkJobs() + ")";
}
/**
* Fixed typeName for ADLSAccounts.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTypeName() {
return this.typeName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy