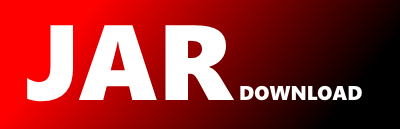
com.atlan.model.assets.Connection Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 10 18:56:32 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.assets;
import com.atlan.Atlan;
import com.atlan.AtlanClient;
import com.atlan.exception.AtlanException;
import com.atlan.exception.ErrorCode;
import com.atlan.exception.InvalidRequestException;
import com.atlan.exception.NotFoundException;
import com.atlan.model.core.AssetMutationResponse;
import com.atlan.model.core.AsyncCreationResponse;
import com.atlan.model.enums.AtlanAnnouncementType;
import com.atlan.model.enums.AtlanConnectionCategory;
import com.atlan.model.enums.AtlanConnectorType;
import com.atlan.model.enums.CertificateStatus;
import com.atlan.model.enums.QueryUsernameStrategy;
import com.atlan.model.fields.AtlanField;
import com.atlan.model.relations.Reference;
import com.atlan.model.relations.UniqueAttributes;
import com.atlan.model.search.FluentSearch;
import com.atlan.util.StringUtils;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.SortedSet;
import java.util.concurrent.ThreadLocalRandom;
import java.util.stream.Collectors;
import javax.annotation.processing.Generated;
import lombok.*;
/**
* Instance of a connection to a data source in Atlan.
*/
@Generated("com.atlan.generators.ModelGeneratorV2")
@SuppressWarnings("cast")
public class Connection extends Asset implements IConnection, IAsset, IReferenceable {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(Connection.class);
private static final long serialVersionUID = 2L;
public static final String TYPE_NAME = "Connection";
/**
* Fixed typeName for Connections.
*/
String typeName;
/**
* Whether using this connection to run queries on the source is allowed (true) or not (false).
*/
@Attribute
Boolean allowQuery;
/**
* Whether using this connection to run preview queries on the source is allowed (true) or not (false).
*/
@Attribute
Boolean allowQueryPreview;
/**
* Type of connection, for example WAREHOUSE, RDBMS, etc.
*/
@Attribute
AtlanConnectionCategory category;
/**
* TBC
*/
@Attribute
SortedSet connectionDbtEnvironments;
/**
* Unique identifier (GUID) for the SSO credentials to use for this connection.
*/
@Attribute
String connectionSSOCredentialGuid;
/**
* Unused. Only the value of connectorType impacts icons.
*/
@Attribute
String connectorIcon;
/**
* Unused. Only the value of connectorType impacts icons.
*/
@Attribute
String connectorImage;
/**
* Credential strategy to use for this connection for queries.
*/
@Attribute
String credentialStrategy;
/**
* Unique identifier (GUID) for the default credentials to use for this connection.
*/
@Attribute
String defaultCredentialGuid;
/**
* Whether this connection has popularity insights (true) or not (false).
*/
@Attribute
Boolean hasPopularityInsights;
/**
* Host name of this connection's source.
*/
@Attribute
String host;
/**
* Whether sample data can be previewed for this connection (true) or not (false).
*/
@Attribute
Boolean isSampleDataPreviewEnabled;
/**
* Number of rows after which results should be uploaded to storage.
*/
@Attribute
Long objectStorageUploadThreshold;
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten, applicable for stream api call made from insight screen
*/
@Attribute
String policyStrategy;
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten. policyStrategyForSamplePreview config is applicable for sample preview call from assets screen
*/
@Attribute
String policyStrategyForSamplePreview;
/**
* Number of days over which popularity is calculated, for example 30 days.
*/
@Attribute
Long popularityInsightsTimeframe;
/**
* Port number to this connection's source.
*/
@Attribute
Integer port;
/**
* Credential strategy to use for this connection for preview queries.
*/
@Attribute
String previewCredentialStrategy;
/**
* Query config for this connection.
*/
@Attribute
String queryConfig;
/**
* Configuration for preview queries.
*/
@Attribute
Map queryPreviewConfig;
/**
* Maximum time a query should be allowed to run before timing out.
*/
@Attribute
Long queryTimeout;
/**
* Username strategy to use for this connection for queries.
*/
@Attribute
QueryUsernameStrategy queryUsernameStrategy;
/**
* Maximum number of rows that can be returned for the source.
*/
@Attribute
Long rowLimit;
/**
* Unused. Only the value of connectorType impacts icons.
*/
@Attribute
String sourceLogo;
/**
* Subcategory of this connection.
*/
@Attribute
String subCategory;
/**
* Whether to upload to S3, GCP, or another storage location (true) or not (false).
*/
@Attribute
Boolean useObjectStorage;
/**
* TBC
*/
@Attribute
Boolean vectorEmbeddingsEnabled;
/**
* TBC
*/
@Attribute
@Date
Long vectorEmbeddingsUpdatedAt;
/**
* Builds the minimal object necessary to create a relationship to a Connection, from a potentially
* more-complete Connection object.
*
* @return the minimal object necessary to relate to the Connection
* @throws InvalidRequestException if any of the minimal set of required properties for a Connection relationship are not found in the initial object
*/
@Override
public Connection trimToReference() throws InvalidRequestException {
if (this.getGuid() != null && !this.getGuid().isEmpty()) {
return refByGuid(this.getGuid());
}
if (this.getQualifiedName() != null && !this.getQualifiedName().isEmpty()) {
return refByQualifiedName(this.getQualifiedName());
}
if (this.getUniqueAttributes() != null && this.getUniqueAttributes().getQualifiedName() != null && !this.getUniqueAttributes().getQualifiedName().isEmpty()) {
return refByQualifiedName(this.getUniqueAttributes().getQualifiedName());
}
throw new InvalidRequestException(ErrorCode.MISSING_REQUIRED_RELATIONSHIP_PARAM, TYPE_NAME, "guid, qualifiedName");
}
/**
* Start a fluent search that will return all Connection assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval. Only active (non-archived) Connection assets will be included.
*
* @return a fluent search that includes all Connection assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select() {
return select(Atlan.getDefaultClient());
}
/**
* Start a fluent search that will return all Connection assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval. Only active (non-archived) Connection assets will be included.
*
* @param client connectivity to the Atlan tenant from which to retrieve the assets
* @return a fluent search that includes all Connection assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(AtlanClient client) {
return select(client, false);
}
/**
* Start a fluent search that will return all Connection assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval.
*
* @param includeArchived when true, archived (soft-deleted) Connections will be included
* @return a fluent search that includes all Connection assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(boolean includeArchived) {
return select(Atlan.getDefaultClient(), includeArchived);
}
/**
* Start a fluent search that will return all Connection assets.
* Additional conditions can be chained onto the returned search before any
* asset retrieval is attempted, ensuring all conditions are pushed-down for
* optimal retrieval.
*
* @param client connectivity to the Atlan tenant from which to retrieve the assets
* @param includeArchived when true, archived (soft-deleted) Connections will be included
* @return a fluent search that includes all Connection assets
*/
public static FluentSearch.FluentSearchBuilder, ?> select(AtlanClient client, boolean includeArchived) {
FluentSearch.FluentSearchBuilder, ?> builder = FluentSearch.builder(client).where(Asset.TYPE_NAME.eq(TYPE_NAME));
if (!includeArchived) {
builder.active();
}
return builder;
}
/**
* Reference to a Connection by GUID. Use this to create a relationship to this Connection,
* where the relationship should be replaced.
*
* @param guid the GUID of the Connection to reference
* @return reference to a Connection that can be used for defining a relationship to a Connection
*/
public static Connection refByGuid(String guid) {
return refByGuid(guid, Reference.SaveSemantic.REPLACE);
}
/**
* Reference to a Connection by GUID. Use this to create a relationship to this Connection,
* where you want to further control how that relationship should be updated (i.e. replaced,
* appended, or removed).
*
* @param guid the GUID of the Connection to reference
* @param semantic how to save this relationship (replace all with this, append it, or remove it)
* @return reference to a Connection that can be used for defining a relationship to a Connection
*/
public static Connection refByGuid(String guid, Reference.SaveSemantic semantic) {
return Connection._internal().guid(guid).semantic(semantic).build();
}
/**
* Reference to a Connection by qualifiedName. Use this to create a relationship to this Connection,
* where the relationship should be replaced.
*
* @param qualifiedName the qualifiedName of the Connection to reference
* @return reference to a Connection that can be used for defining a relationship to a Connection
*/
public static Connection refByQualifiedName(String qualifiedName) {
return refByQualifiedName(qualifiedName, Reference.SaveSemantic.REPLACE);
}
/**
* Reference to a Connection by qualifiedName. Use this to create a relationship to this Connection,
* where you want to further control how that relationship should be updated (i.e. replaced,
* appended, or removed).
*
* @param qualifiedName the qualifiedName of the Connection to reference
* @param semantic how to save this relationship (replace all with this, append it, or remove it)
* @return reference to a Connection that can be used for defining a relationship to a Connection
*/
public static Connection refByQualifiedName(String qualifiedName, Reference.SaveSemantic semantic) {
return Connection._internal().uniqueAttributes(UniqueAttributes.builder().qualifiedName(qualifiedName).build()).semantic(semantic).build();
}
/**
* Retrieves a Connection by one of its identifiers, complete with all of its relationships.
*
* @param id of the Connection to retrieve, either its GUID or its full qualifiedName
* @return the requested full Connection, complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the Connection does not exist or the provided GUID is not a Connection
*/
@JsonIgnore
public static Connection get(String id) throws AtlanException {
return get(Atlan.getDefaultClient(), id);
}
/**
* Retrieves a Connection by one of its identifiers, complete with all of its relationships.
*
* @param client connectivity to the Atlan tenant from which to retrieve the asset
* @param id of the Connection to retrieve, either its GUID or its full qualifiedName
* @return the requested full Connection, complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the Connection does not exist or the provided GUID is not a Connection
*/
@JsonIgnore
public static Connection get(AtlanClient client, String id) throws AtlanException {
return get(client, id, true);
}
/**
* Retrieves a Connection by one of its identifiers, optionally complete with all of its relationships.
*
* @param client connectivity to the Atlan tenant from which to retrieve the asset
* @param id of the Connection to retrieve, either its GUID or its full qualifiedName
* @param includeRelationships if true, all of the asset's relationships will also be retrieved; if false, no relationships will be retrieved
* @return the requested full Connection, optionally complete with all of its relationships
* @throws AtlanException on any error during the API invocation, such as the {@link NotFoundException} if the Connection does not exist or the provided GUID is not a Connection
*/
@JsonIgnore
public static Connection get(AtlanClient client, String id, boolean includeRelationships) throws AtlanException {
if (id == null) {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_GUID, "(null)");
} else if (StringUtils.isUUID(id)) {
Asset asset = Asset.get(client, id, includeRelationships);
if (asset == null) {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_GUID, id);
} else if (asset instanceof Connection) {
return (Connection) asset;
} else {
throw new NotFoundException(ErrorCode.ASSET_NOT_TYPE_REQUESTED, id, TYPE_NAME);
}
} else {
Asset asset = Asset.get(client, TYPE_NAME, id, includeRelationships);
if (asset instanceof Connection) {
return (Connection) asset;
} else {
throw new NotFoundException(ErrorCode.ASSET_NOT_FOUND_BY_QN, id, TYPE_NAME);
}
}
}
/**
* Restore the archived (soft-deleted) Connection to active.
*
* @param qualifiedName for the Connection
* @return true if the Connection is now active, and false otherwise
* @throws AtlanException on any API problems
*/
public static boolean restore(String qualifiedName) throws AtlanException {
return restore(Atlan.getDefaultClient(), qualifiedName);
}
/**
* Restore the archived (soft-deleted) Connection to active.
*
* @param client connectivity to the Atlan tenant on which to restore the asset
* @param qualifiedName for the Connection
* @return true if the Connection is now active, and false otherwise
* @throws AtlanException on any API problems
*/
public static boolean restore(AtlanClient client, String qualifiedName) throws AtlanException {
return Asset.restore(client, TYPE_NAME, qualifiedName);
}
/**
* Determine the connector type from the provided qualifiedName.
*
* @param qualifiedName of the connection
* @return the connector type, or null if the qualifiedName is not for a connected asset
*/
public static AtlanConnectorType getConnectorTypeFromQualifiedName(String qualifiedName) {
return getConnectorTypeFromQualifiedName(qualifiedName.split("/"));
}
/**
* Determine the connector type from the provided qualifiedName.
*
* @param tokens of the qualifiedName, from which to determine the connector type
* @return the connector type, or null if the qualifiedName is not for a connected asset
*/
public static AtlanConnectorType getConnectorTypeFromQualifiedName(String[] tokens) {
if (tokens.length > 1) {
return AtlanConnectorType.fromValue(tokens[1]);
}
return null;
}
/**
* Builds the minimal object necessary to create a connection, using "All Admins" as the default
* set of connection admins.
*
* @param name of the connection
* @param connectorType type of the connection's connector (this determines what logo appears for the assets)
* @return the minimal object necessary to create the connection, as a builder
* @throws AtlanException on any error related to the request, such as an inability to retrieve the existing admins in the system
*/
public static ConnectionBuilder, ?> creator(String name, AtlanConnectorType connectorType) throws AtlanException {
AtlanClient client = Atlan.getDefaultClient();
return creator(client, name, connectorType, List.of(client.getRoleCache().getIdForName("$admin")), null, null);
}
/**
* Builds the minimal object necessary to create a connection.
* Note: at least one of {@code #adminRoles}, {@code #adminGroups}, or {@code #adminUsers} must be
* provided or an InvalidRequestException will be thrown.
*
* @param name of the connection
* @param connectorType type of the connection's connector (this determines what logo appears for the assets)
* @param adminRoles the GUIDs of the roles that can administer this connection
* @param adminGroups the (internal) names of the groups that can administer this connection
* @param adminUsers the (internal) names of the users that can administer this connection
* @return the minimal object necessary to create the connection, as a builder
* @throws InvalidRequestException if no admin has been defined for the connection, or an invalid admin has been defined
* @throws NotFoundException if a non-existent admin has been defined for the connection
* @throws AtlanException on any other error related to the request, such as an inability to retrieve the existing admins in the system
*/
public static ConnectionBuilder, ?> creator(String name, AtlanConnectorType connectorType, List adminRoles, List adminGroups, List adminUsers) throws AtlanException {
return creator(Atlan.getDefaultClient(), name, connectorType, adminRoles, adminGroups, adminUsers);
}
/**
* Builds the minimal object necessary to create a connection.
* Note: at least one of {@code #adminRoles}, {@code #adminGroups}, or {@code #adminUsers} must be
* provided or an InvalidRequestException will be thrown.
*
* @param client connectivity to the Atlan tenant where the connection is intended to be created
* @param name of the connection
* @param connectorType type of the connection's connector (this determines what logo appears for the assets)
* @param adminRoles the GUIDs of the roles that can administer this connection
* @param adminGroups the (internal) names of the groups that can administer this connection
* @param adminUsers the (internal) names of the users that can administer this connection
* @return the minimal object necessary to create the connection, as a builder
* @throws InvalidRequestException if no admin has been defined for the connection, or an invalid admin has been defined
* @throws NotFoundException if a non-existent admin has been defined for the connection
* @throws AtlanException on any other error related to the request, such as an inability to retrieve the existing admins in the system
*/
public static ConnectionBuilder, ?> creator(AtlanClient client, String name, AtlanConnectorType connectorType, List adminRoles, List adminGroups, List adminUsers) throws AtlanException {
boolean adminFound = false;
ConnectionBuilder, ?> builder = Connection._internal().guid("-" + ThreadLocalRandom.current().nextLong(0, Long.MAX_VALUE - 1)).name(name).qualifiedName(generateQualifiedName(connectorType.getValue())).category(connectorType.getCategory()).connectorType(connectorType);
if (adminRoles != null && !adminRoles.isEmpty()) {
for (String roleId : adminRoles) {
client.getRoleCache().getNameForId(roleId);
}
adminFound = true;
builder.adminRoles(adminRoles);
} else {
builder.nullField("adminRoles");
}
if (adminGroups != null && !adminGroups.isEmpty()) {
for (String groupAlias : adminGroups) {
client.getGroupCache().getIdForName(groupAlias);
}
adminFound = true;
builder.adminGroups(adminGroups);
} else {
builder.nullField("adminGroups");
}
if (adminUsers != null && !adminUsers.isEmpty()) {
for (String userName : adminUsers) {
client.getUserCache().getIdForName(userName);
}
adminFound = true;
builder.adminUsers(adminUsers);
} else {
builder.nullField("adminUsers");
}
if (adminFound) {
return builder;
} else {
throw new InvalidRequestException(ErrorCode.NO_CONNECTION_ADMIN);
}
}
/**
* If an asset with the same qualifiedName exists, updates the existing asset. Otherwise, creates the asset.
* No Atlan tags or custom metadata will be changed if updating an existing asset, irrespective of what
* is included in the asset itself when the method is called.
*
* @return details of the created or updated asset
* @throws AtlanException on any error during the API invocation
* @throws NotFoundException if any of the provided connection admins do not actually exist
*/
@Override
public AsyncCreationResponse save() throws AtlanException {
return save(Atlan.getDefaultClient());
}
/**
* If an asset with the same qualifiedName exists, updates the existing asset. Otherwise, creates the asset.
* No Atlan tags or custom metadata will be changed if updating an existing asset, irrespective of what
* is included in the asset itself when the method is called.
*
* @param client connectivity to the Atlan tenant where this connection should be saved
* @return details of the created or updated asset
* @throws AtlanException on any error during the API invocation
* @throws NotFoundException if any of the provided connection admins do not actually exist
*/
@Override
public AsyncCreationResponse save(AtlanClient client) throws AtlanException {
// Validate the provided connection admins prior to attempting to create
// (the cache retrievals will throw errors directly if there are any)
if (adminRoles != null && !adminRoles.isEmpty()) {
for (String roleId : adminRoles) {
client.getRoleCache().getNameForId(roleId);
}
}
if (adminGroups != null && !adminGroups.isEmpty()) {
for (String groupAlias : adminGroups) {
client.getGroupCache().getIdForName(groupAlias);
}
}
if (adminUsers != null && !adminUsers.isEmpty()) {
for (String userName : adminUsers) {
client.getUserCache().getIdForName(userName);
}
}
return client.assets.save(this, false);
}
/**
* If no asset exists, has the same behavior as the {@link #save()} method.
* If an asset does exist, optionally overwrites any Atlan tags. Custom metadata will always
* be entirely ignored using this method.
*
* @param replaceAtlanTags whether to replace Atlan tags during an update (true) or not (false)
* @return details of the created or updated asset
* @throws AtlanException on any error during the API invocation
* @throws NotFoundException if any of the provided connection admins do not actually exist
*/
@Override
public AsyncCreationResponse save(boolean replaceAtlanTags) throws AtlanException {
return save(Atlan.getDefaultClient(), replaceAtlanTags);
}
/**
* If no asset exists, has the same behavior as the {@link #save()} method.
* If an asset does exist, optionally overwrites any Atlan tags. Custom metadata will always
* be entirely ignored using this method.
*
* @param client connectivity to the Atlan tenant where this connection should be saved
* @param replaceAtlanTags whether to replace Atlan tags during an update (true) or not (false)
* @return details of the created or updated asset
* @throws AtlanException on any error during the API invocation
* @throws NotFoundException if any of the provided connection admins do not actually exist
*/
@Override
public AsyncCreationResponse save(AtlanClient client, boolean replaceAtlanTags) throws AtlanException {
// Validate the provided connection admins prior to attempting to create
// (the cache retrievals will throw errors directly if there are any)
if (adminRoles != null && !adminRoles.isEmpty()) {
for (String roleId : adminRoles) {
client.getRoleCache().getNameForId(roleId);
}
}
if (adminGroups != null && !adminGroups.isEmpty()) {
for (String groupAlias : adminGroups) {
client.getGroupCache().getIdForName(groupAlias);
}
}
if (adminUsers != null && !adminUsers.isEmpty()) {
for (String userName : adminUsers) {
client.getUserCache().getIdForName(userName);
}
}
return client.assets.save(this, replaceAtlanTags);
}
/**
* Add the API token configured for the default client as an admin for this Connection.
* This is necessary to allow the API token to manage policies for the connection.
*
* @param impersonationToken a bearer token for an actual user who is already an admin for the Connection, NOT an API token
* @throws AtlanException on any error during API invocation
*/
public AssetMutationResponse addApiTokenAsAdmin(final String impersonationToken) throws AtlanException {
return Asset.addApiTokenAsAdmin(getGuid(), impersonationToken);
}
/**
* Generate a unique connection name.
*
* @param connectorType the name of the type of the connection's connector
* @return a unique name for the connection
*/
public static synchronized String generateQualifiedName(AtlanConnectorType connectorType) {
return generateQualifiedName(connectorType.getValue());
}
/**
* Generate a unique connection name.
*
* @param connectorType the name of the type of the connection's connector
* @return a unique name for the connection
*/
private static synchronized String generateQualifiedName(String connectorType) {
long now = System.currentTimeMillis() / 1000;
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
log.warn("Connection qualifiedName construction interrupted - exclusivity cannot be guaranteed.", e);
}
return "default/" + connectorType + "/" + now;
}
/**
* Builds the minimal object necessary to update a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the minimal request necessary to update the Connection, as a builder
*/
public static ConnectionBuilder, ?> updater(String qualifiedName, String name) {
return Connection._internal().guid("-" + ThreadLocalRandom.current().nextLong(0, Long.MAX_VALUE - 1)).qualifiedName(qualifiedName).name(name);
}
/**
* Builds the minimal object necessary to apply an update to a Connection, from a potentially
* more-complete Connection object.
*
* @return the minimal object necessary to update the Connection, as a builder
* @throws InvalidRequestException if any of the minimal set of required properties for Connection are not found in the initial object
*/
@Override
public ConnectionBuilder, ?> trimToRequired() throws InvalidRequestException {
validateRequired(TYPE_NAME, Map.of("qualifiedName", this.getQualifiedName(), "name", this.getName()));
return updater(this.getQualifiedName(), this.getName());
}
/**
* Retrieve the epoch component of the connection name from its qualifiedName.
*
* @param qualifiedName of the connection
* @return the epoch component of the qualifiedName
*/
@JsonIgnore
public static String getEpochFromQualifiedName(String qualifiedName) {
return qualifiedName.substring(qualifiedName.lastIndexOf("/") + 1);
}
/**
* Find a connection by its human-readable name and type. Only the bare minimum set of attributes and no
* relationships will be retrieved for the connection, if found.
*
* @param name of the connection
* @param type of the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(String name, AtlanConnectorType type) throws AtlanException {
return findByName(name, type, (List) null);
}
/**
* Find a connection by its human-readable name and type.
*
* @param name of the connection
* @param type of the connection
* @param attributes an optional collection of attributes (unchecked) to retrieve for the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(String name, AtlanConnectorType type, Collection attributes) throws AtlanException {
return findByName(Atlan.getDefaultClient(), name, type, attributes);
}
/**
* Find a connection by its human-readable name and type.
*
* @param name of the connection
* @param type of the connection
* @param attributes an optional collection of attributes (checked) to retrieve for the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(String name, AtlanConnectorType type, List attributes) throws AtlanException {
return findByName(Atlan.getDefaultClient(), name, type, attributes);
}
/**
* Find a connection by its human-readable name and type. Only the bare minimum set of attributes and no
* relationships will be retrieved for the connection, if found.
*
* @param client connectivity to the Atlan tenant in which to search for the connection
* @param name of the connection
* @param type of the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(AtlanClient client, String name, AtlanConnectorType type) throws AtlanException {
return findByName(client, name, type, (List) null);
}
/**
* Find a connection by its human-readable name and type.
*
* @param client connectivity to the Atlan tenant in which to search for the connection
* @param name of the connection
* @param type of the connection
* @param attributes an optional collection of attributes to retrieve for the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(AtlanClient client, String name, AtlanConnectorType type, Collection attributes) throws AtlanException {
List results = new ArrayList<>();
Connection.select(client).where(Connection.NAME.eq(name)).where(Connection.CONNECTOR_TYPE.eq(type.getValue()))._includesOnResults(attributes == null ? Collections.emptyList() : attributes).stream().filter(a -> a instanceof Connection).forEach(c -> results.add((Connection) c));
if (results.isEmpty()) {
throw new NotFoundException(ErrorCode.CONNECTION_NOT_FOUND_BY_NAME, name, type.getValue());
}
return results;
}
/**
* Find a connection by its human-readable name and type.
*
* @param client connectivity to the Atlan tenant in which to search for the connection
* @param name of the connection
* @param type of the connection
* @param attributes an optional collection of attributes (checked) to retrieve for the connection
* @return all connections with that name and type, if found
* @throws AtlanException on any API problems
* @throws NotFoundException if the connection does not exist
*/
public static List findByName(AtlanClient client, String name, AtlanConnectorType type, List attributes) throws AtlanException {
List results = new ArrayList<>();
Connection.select(client).where(Connection.NAME.eq(name)).where(Connection.CONNECTOR_TYPE.eq(type.getValue())).includesOnResults(attributes == null ? Collections.emptyList() : attributes).stream().filter(a -> a instanceof Connection).forEach(c -> results.add((Connection) c));
if (results.isEmpty()) {
throw new NotFoundException(ErrorCode.CONNECTION_NOT_FOUND_BY_NAME, name, type.getValue());
}
return results;
}
/**
* Retrieve the qualifiedNames of all connections that exist in Atlan.
*
* @return list of all connection qualifiedNames
* @throws AtlanException on any API problems
*/
public static List getAllQualifiedNames() throws AtlanException {
return getAllQualifiedNames(Atlan.getDefaultClient());
}
/**
* Retrieve the qualifiedNames of all connections that exist in Atlan.
*
* @param client connectivity to the Atlan tenant from which to retrieve the qualifiedNames
* @return list of all connection qualifiedNames
* @throws AtlanException on any API problems
*/
public static List getAllQualifiedNames(AtlanClient client) throws AtlanException {
return Connection.select(client).includeOnResults(Connection.QUALIFIED_NAME).pageSize(50).stream().map(Asset::getQualifiedName).collect(Collectors.toList());
}
/**
* Remove the system description from a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeDescription(String qualifiedName, String name) throws AtlanException {
return removeDescription(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the system description from a Connection.
*
* @param client connectivity to the Atlan tenant on which to remove the asset's description
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeDescription(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (Connection) Asset.removeDescription(client, updater(qualifiedName, name));
}
/**
* Remove the user's description from a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeUserDescription(String qualifiedName, String name) throws AtlanException {
return removeUserDescription(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the user's description from a Connection.
*
* @param client connectivity to the Atlan tenant on which to remove the asset's description
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeUserDescription(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (Connection) Asset.removeUserDescription(client, updater(qualifiedName, name));
}
/**
* Remove the owners from a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeOwners(String qualifiedName, String name) throws AtlanException {
return removeOwners(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the owners from a Connection.
*
* @param client connectivity to the Atlan tenant from which to remove the Connection's owners
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeOwners(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (Connection) Asset.removeOwners(client, updater(qualifiedName, name));
}
/**
* Update the certificate on a Connection.
*
* @param qualifiedName of the Connection
* @param certificate to use
* @param message (optional) message, or null if no message
* @return the updated Connection, or null if the update failed
* @throws AtlanException on any API problems
*/
public static Connection updateCertificate(String qualifiedName, CertificateStatus certificate, String message) throws AtlanException {
return updateCertificate(Atlan.getDefaultClient(), qualifiedName, certificate, message);
}
/**
* Update the certificate on a Connection.
*
* @param client connectivity to the Atlan tenant on which to update the Connection's certificate
* @param qualifiedName of the Connection
* @param certificate to use
* @param message (optional) message, or null if no message
* @return the updated Connection, or null if the update failed
* @throws AtlanException on any API problems
*/
public static Connection updateCertificate(AtlanClient client, String qualifiedName, CertificateStatus certificate, String message) throws AtlanException {
return (Connection) Asset.updateCertificate(client, _internal(), TYPE_NAME, qualifiedName, certificate, message);
}
/**
* Remove the certificate from a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeCertificate(String qualifiedName, String name) throws AtlanException {
return removeCertificate(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the certificate from a Connection.
*
* @param client connectivity to the Atlan tenant from which to remove the Connection's certificate
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeCertificate(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (Connection) Asset.removeCertificate(client, updater(qualifiedName, name));
}
/**
* Update the announcement on a Connection.
*
* @param qualifiedName of the Connection
* @param type type of announcement to set
* @param title (optional) title of the announcement to set (or null for no title)
* @param message (optional) message of the announcement to set (or null for no message)
* @return the result of the update, or null if the update failed
* @throws AtlanException on any API problems
*/
public static Connection updateAnnouncement(String qualifiedName, AtlanAnnouncementType type, String title, String message) throws AtlanException {
return updateAnnouncement(Atlan.getDefaultClient(), qualifiedName, type, title, message);
}
/**
* Update the announcement on a Connection.
*
* @param client connectivity to the Atlan tenant on which to update the Connection's announcement
* @param qualifiedName of the Connection
* @param type type of announcement to set
* @param title (optional) title of the announcement to set (or null for no title)
* @param message (optional) message of the announcement to set (or null for no message)
* @return the result of the update, or null if the update failed
* @throws AtlanException on any API problems
*/
public static Connection updateAnnouncement(AtlanClient client, String qualifiedName, AtlanAnnouncementType type, String title, String message) throws AtlanException {
return (Connection) Asset.updateAnnouncement(client, _internal(), TYPE_NAME, qualifiedName, type, title, message);
}
/**
* Remove the announcement from a Connection.
*
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeAnnouncement(String qualifiedName, String name) throws AtlanException {
return removeAnnouncement(Atlan.getDefaultClient(), qualifiedName, name);
}
/**
* Remove the announcement from a Connection.
*
* @param client connectivity to the Atlan client from which to remove the Connection's announcement
* @param qualifiedName of the Connection
* @param name of the Connection
* @return the updated Connection, or null if the removal failed
* @throws AtlanException on any API problems
*/
public static Connection removeAnnouncement(AtlanClient client, String qualifiedName, String name) throws AtlanException {
return (Connection) Asset.removeAnnouncement(client, updater(qualifiedName, name));
}
/**
* Replace the terms linked to the Connection.
*
* @param qualifiedName for the Connection
* @param name human-readable name of the Connection
* @param terms the list of terms to replace on the Connection, or null to remove all terms from the Connection
* @return the Connection that was updated (note that it will NOT contain details of the replaced terms)
* @throws AtlanException on any API problems
*/
public static Connection replaceTerms(String qualifiedName, String name, List terms) throws AtlanException {
return replaceTerms(Atlan.getDefaultClient(), qualifiedName, name, terms);
}
/**
* Replace the terms linked to the Connection.
*
* @param client connectivity to the Atlan tenant on which to replace the Connection's assigned terms
* @param qualifiedName for the Connection
* @param name human-readable name of the Connection
* @param terms the list of terms to replace on the Connection, or null to remove all terms from the Connection
* @return the Connection that was updated (note that it will NOT contain details of the replaced terms)
* @throws AtlanException on any API problems
*/
public static Connection replaceTerms(AtlanClient client, String qualifiedName, String name, List terms) throws AtlanException {
return (Connection) Asset.replaceTerms(client, updater(qualifiedName, name), terms);
}
/**
* Link additional terms to the Connection, without replacing existing terms linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing terms,
* and a second to append the new terms.
*
* @param qualifiedName for the Connection
* @param terms the list of terms to append to the Connection
* @return the Connection that was updated (note that it will NOT contain details of the appended terms)
* @throws AtlanException on any API problems
*/
public static Connection appendTerms(String qualifiedName, List terms) throws AtlanException {
return appendTerms(Atlan.getDefaultClient(), qualifiedName, terms);
}
/**
* Link additional terms to the Connection, without replacing existing terms linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing terms,
* and a second to append the new terms.
*
* @param client connectivity to the Atlan tenant on which to append terms to the Connection
* @param qualifiedName for the Connection
* @param terms the list of terms to append to the Connection
* @return the Connection that was updated (note that it will NOT contain details of the appended terms)
* @throws AtlanException on any API problems
*/
public static Connection appendTerms(AtlanClient client, String qualifiedName, List terms) throws AtlanException {
return (Connection) Asset.appendTerms(client, TYPE_NAME, qualifiedName, terms);
}
/**
* Remove terms from a Connection, without replacing all existing terms linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing terms,
* and a second to remove the provided terms.
*
* @param qualifiedName for the Connection
* @param terms the list of terms to remove from the Connection, which must be referenced by GUID
* @return the Connection that was updated (note that it will NOT contain details of the resulting terms)
* @throws AtlanException on any API problems
*/
public static Connection removeTerms(String qualifiedName, List terms) throws AtlanException {
return removeTerms(Atlan.getDefaultClient(), qualifiedName, terms);
}
/**
* Remove terms from a Connection, without replacing all existing terms linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing terms,
* and a second to remove the provided terms.
*
* @param client connectivity to the Atlan tenant from which to remove terms from the Connection
* @param qualifiedName for the Connection
* @param terms the list of terms to remove from the Connection, which must be referenced by GUID
* @return the Connection that was updated (note that it will NOT contain details of the resulting terms)
* @throws AtlanException on any API problems
*/
public static Connection removeTerms(AtlanClient client, String qualifiedName, List terms) throws AtlanException {
return (Connection) Asset.removeTerms(client, TYPE_NAME, qualifiedName, terms);
}
/**
* Add Atlan tags to a Connection, without replacing existing Atlan tags linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param qualifiedName of the Connection
* @param atlanTagNames human-readable names of the Atlan tags to add
* @throws AtlanException on any API problems
* @return the updated Connection
*/
public static Connection appendAtlanTags(String qualifiedName, List atlanTagNames) throws AtlanException {
return appendAtlanTags(Atlan.getDefaultClient(), qualifiedName, atlanTagNames);
}
/**
* Add Atlan tags to a Connection, without replacing existing Atlan tags linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param client connectivity to the Atlan tenant on which to append Atlan tags to the Connection
* @param qualifiedName of the Connection
* @param atlanTagNames human-readable names of the Atlan tags to add
* @throws AtlanException on any API problems
* @return the updated Connection
*/
public static Connection appendAtlanTags(AtlanClient client, String qualifiedName, List atlanTagNames) throws AtlanException {
return (Connection) Asset.appendAtlanTags(client, TYPE_NAME, qualifiedName, atlanTagNames);
}
/**
* Add Atlan tags to a Connection, without replacing existing Atlan tags linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param qualifiedName of the Connection
* @param atlanTagNames human-readable names of the Atlan tags to add
* @param propagate whether to propagate the Atlan tag (true) or not (false)
* @param removePropagationsOnDelete whether to remove the propagated Atlan tags when the Atlan tag is removed from this asset (true) or not (false)
* @param restrictLineagePropagation whether to avoid propagating through lineage (true) or do propagate through lineage (false)
* @throws AtlanException on any API problems
* @return the updated Connection
*/
public static Connection appendAtlanTags(String qualifiedName, List atlanTagNames, boolean propagate, boolean removePropagationsOnDelete, boolean restrictLineagePropagation) throws AtlanException {
return appendAtlanTags(Atlan.getDefaultClient(), qualifiedName, atlanTagNames, propagate, removePropagationsOnDelete, restrictLineagePropagation);
}
/**
* Add Atlan tags to a Connection, without replacing existing Atlan tags linked to the Connection.
* Note: this operation must make two API calls — one to retrieve the Connection's existing Atlan tags,
* and a second to append the new Atlan tags.
*
* @param client connectivity to the Atlan tenant on which to append Atlan tags to the Connection
* @param qualifiedName of the Connection
* @param atlanTagNames human-readable names of the Atlan tags to add
* @param propagate whether to propagate the Atlan tag (true) or not (false)
* @param removePropagationsOnDelete whether to remove the propagated Atlan tags when the Atlan tag is removed from this asset (true) or not (false)
* @param restrictLineagePropagation whether to avoid propagating through lineage (true) or do propagate through lineage (false)
* @throws AtlanException on any API problems
* @return the updated Connection
*/
public static Connection appendAtlanTags(AtlanClient client, String qualifiedName, List atlanTagNames, boolean propagate, boolean removePropagationsOnDelete, boolean restrictLineagePropagation) throws AtlanException {
return (Connection) Asset.appendAtlanTags(client, TYPE_NAME, qualifiedName, atlanTagNames, propagate, removePropagationsOnDelete, restrictLineagePropagation);
}
/**
* Remove an Atlan tag from a Connection.
*
* @param qualifiedName of the Connection
* @param atlanTagName human-readable name of the Atlan tag to remove
* @throws AtlanException on any API problems, or if the Atlan tag does not exist on the Connection
*/
public static void removeAtlanTag(String qualifiedName, String atlanTagName) throws AtlanException {
removeAtlanTag(Atlan.getDefaultClient(), qualifiedName, atlanTagName);
}
/**
* Remove an Atlan tag from a Connection.
*
* @param client connectivity to the Atlan tenant from which to remove an Atlan tag from a Connection
* @param qualifiedName of the Connection
* @param atlanTagName human-readable name of the Atlan tag to remove
* @throws AtlanException on any API problems, or if the Atlan tag does not exist on the Connection
*/
public static void removeAtlanTag(AtlanClient client, String qualifiedName, String atlanTagName) throws AtlanException {
Asset.removeAtlanTag(client, TYPE_NAME, qualifiedName, atlanTagName);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static String $default$typeName() {
return TYPE_NAME;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class ConnectionBuilder> extends Asset.AssetBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private boolean typeName$set;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String typeName$value;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean allowQuery;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean allowQueryPreview;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private AtlanConnectionCategory category;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList connectionDbtEnvironments;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String connectionSSOCredentialGuid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String connectorIcon;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String connectorImage;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String credentialStrategy;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String defaultCredentialGuid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean hasPopularityInsights;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String host;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean isSampleDataPreviewEnabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Long objectStorageUploadThreshold;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String policyStrategy;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String policyStrategyForSamplePreview;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Long popularityInsightsTimeframe;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer port;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String previewCredentialStrategy;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String queryConfig;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList queryPreviewConfig$key;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList queryPreviewConfig$value;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Long queryTimeout;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private QueryUsernameStrategy queryUsernameStrategy;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Long rowLimit;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String sourceLogo;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String subCategory;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean useObjectStorage;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean vectorEmbeddingsEnabled;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Long vectorEmbeddingsUpdatedAt;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected B $fillValuesFrom(final C instance) {
super.$fillValuesFrom(instance);
Connection.ConnectionBuilder.$fillValuesFromInstanceIntoBuilder(instance, this);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static void $fillValuesFromInstanceIntoBuilder(final Connection instance, final Connection.ConnectionBuilder, ?> b) {
b.typeName(instance.typeName);
b.allowQuery(instance.allowQuery);
b.allowQueryPreview(instance.allowQueryPreview);
b.category(instance.category);
b.connectionDbtEnvironments(instance.connectionDbtEnvironments == null ? java.util.Collections.emptySortedSet() : instance.connectionDbtEnvironments);
b.connectionSSOCredentialGuid(instance.connectionSSOCredentialGuid);
b.connectorIcon(instance.connectorIcon);
b.connectorImage(instance.connectorImage);
b.credentialStrategy(instance.credentialStrategy);
b.defaultCredentialGuid(instance.defaultCredentialGuid);
b.hasPopularityInsights(instance.hasPopularityInsights);
b.host(instance.host);
b.isSampleDataPreviewEnabled(instance.isSampleDataPreviewEnabled);
b.objectStorageUploadThreshold(instance.objectStorageUploadThreshold);
b.policyStrategy(instance.policyStrategy);
b.policyStrategyForSamplePreview(instance.policyStrategyForSamplePreview);
b.popularityInsightsTimeframe(instance.popularityInsightsTimeframe);
b.port(instance.port);
b.previewCredentialStrategy(instance.previewCredentialStrategy);
b.queryConfig(instance.queryConfig);
b.queryPreviewConfig(instance.queryPreviewConfig == null ? java.util.Collections.emptyMap() : instance.queryPreviewConfig);
b.queryTimeout(instance.queryTimeout);
b.queryUsernameStrategy(instance.queryUsernameStrategy);
b.rowLimit(instance.rowLimit);
b.sourceLogo(instance.sourceLogo);
b.subCategory(instance.subCategory);
b.useObjectStorage(instance.useObjectStorage);
b.vectorEmbeddingsEnabled(instance.vectorEmbeddingsEnabled);
b.vectorEmbeddingsUpdatedAt(instance.vectorEmbeddingsUpdatedAt);
}
/**
* Fixed typeName for Connections.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B typeName(final String typeName) {
this.typeName$value = typeName;
typeName$set = true;
return self();
}
/**
* Whether using this connection to run queries on the source is allowed (true) or not (false).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B allowQuery(final Boolean allowQuery) {
this.allowQuery = allowQuery;
return self();
}
/**
* Whether using this connection to run preview queries on the source is allowed (true) or not (false).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B allowQueryPreview(final Boolean allowQueryPreview) {
this.allowQueryPreview = allowQueryPreview;
return self();
}
/**
* Type of connection, for example WAREHOUSE, RDBMS, etc.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B category(final AtlanConnectionCategory category) {
this.category = category;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B connectionDbtEnvironment(final String connectionDbtEnvironment) {
if (this.connectionDbtEnvironments == null) this.connectionDbtEnvironments = new java.util.ArrayList();
this.connectionDbtEnvironments.add(connectionDbtEnvironment);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B connectionDbtEnvironments(final java.util.Collection extends String> connectionDbtEnvironments) {
if (connectionDbtEnvironments == null) {
throw new java.lang.NullPointerException("connectionDbtEnvironments cannot be null");
}
if (this.connectionDbtEnvironments == null) this.connectionDbtEnvironments = new java.util.ArrayList();
this.connectionDbtEnvironments.addAll(connectionDbtEnvironments);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearConnectionDbtEnvironments() {
if (this.connectionDbtEnvironments != null) this.connectionDbtEnvironments.clear();
return self();
}
/**
* Unique identifier (GUID) for the SSO credentials to use for this connection.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B connectionSSOCredentialGuid(final String connectionSSOCredentialGuid) {
this.connectionSSOCredentialGuid = connectionSSOCredentialGuid;
return self();
}
/**
* Unused. Only the value of connectorType impacts icons.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B connectorIcon(final String connectorIcon) {
this.connectorIcon = connectorIcon;
return self();
}
/**
* Unused. Only the value of connectorType impacts icons.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B connectorImage(final String connectorImage) {
this.connectorImage = connectorImage;
return self();
}
/**
* Credential strategy to use for this connection for queries.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B credentialStrategy(final String credentialStrategy) {
this.credentialStrategy = credentialStrategy;
return self();
}
/**
* Unique identifier (GUID) for the default credentials to use for this connection.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B defaultCredentialGuid(final String defaultCredentialGuid) {
this.defaultCredentialGuid = defaultCredentialGuid;
return self();
}
/**
* Whether this connection has popularity insights (true) or not (false).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B hasPopularityInsights(final Boolean hasPopularityInsights) {
this.hasPopularityInsights = hasPopularityInsights;
return self();
}
/**
* Host name of this connection's source.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B host(final String host) {
this.host = host;
return self();
}
/**
* Whether sample data can be previewed for this connection (true) or not (false).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B isSampleDataPreviewEnabled(final Boolean isSampleDataPreviewEnabled) {
this.isSampleDataPreviewEnabled = isSampleDataPreviewEnabled;
return self();
}
/**
* Number of rows after which results should be uploaded to storage.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B objectStorageUploadThreshold(final Long objectStorageUploadThreshold) {
this.objectStorageUploadThreshold = objectStorageUploadThreshold;
return self();
}
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten, applicable for stream api call made from insight screen
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B policyStrategy(final String policyStrategy) {
this.policyStrategy = policyStrategy;
return self();
}
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten. policyStrategyForSamplePreview config is applicable for sample preview call from assets screen
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B policyStrategyForSamplePreview(final String policyStrategyForSamplePreview) {
this.policyStrategyForSamplePreview = policyStrategyForSamplePreview;
return self();
}
/**
* Number of days over which popularity is calculated, for example 30 days.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B popularityInsightsTimeframe(final Long popularityInsightsTimeframe) {
this.popularityInsightsTimeframe = popularityInsightsTimeframe;
return self();
}
/**
* Port number to this connection's source.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B port(final Integer port) {
this.port = port;
return self();
}
/**
* Credential strategy to use for this connection for preview queries.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B previewCredentialStrategy(final String previewCredentialStrategy) {
this.previewCredentialStrategy = previewCredentialStrategy;
return self();
}
/**
* Query config for this connection.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B queryConfig(final String queryConfig) {
this.queryConfig = queryConfig;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B putQueryPreviewConfig(final String putQueryPreviewConfigKey, final String putQueryPreviewConfigValue) {
if (this.queryPreviewConfig$key == null) {
this.queryPreviewConfig$key = new java.util.ArrayList();
this.queryPreviewConfig$value = new java.util.ArrayList();
}
this.queryPreviewConfig$key.add(putQueryPreviewConfigKey);
this.queryPreviewConfig$value.add(putQueryPreviewConfigValue);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B queryPreviewConfig(final java.util.Map extends String, ? extends String> queryPreviewConfig) {
if (queryPreviewConfig == null) {
throw new java.lang.NullPointerException("queryPreviewConfig cannot be null");
}
if (this.queryPreviewConfig$key == null) {
this.queryPreviewConfig$key = new java.util.ArrayList();
this.queryPreviewConfig$value = new java.util.ArrayList();
}
for (final java.util.Map.Entry extends String, ? extends String> $lombokEntry : queryPreviewConfig.entrySet()) {
this.queryPreviewConfig$key.add($lombokEntry.getKey());
this.queryPreviewConfig$value.add($lombokEntry.getValue());
}
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearQueryPreviewConfig() {
if (this.queryPreviewConfig$key != null) {
this.queryPreviewConfig$key.clear();
this.queryPreviewConfig$value.clear();
}
return self();
}
/**
* Maximum time a query should be allowed to run before timing out.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B queryTimeout(final Long queryTimeout) {
this.queryTimeout = queryTimeout;
return self();
}
/**
* Username strategy to use for this connection for queries.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B queryUsernameStrategy(final QueryUsernameStrategy queryUsernameStrategy) {
this.queryUsernameStrategy = queryUsernameStrategy;
return self();
}
/**
* Maximum number of rows that can be returned for the source.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B rowLimit(final Long rowLimit) {
this.rowLimit = rowLimit;
return self();
}
/**
* Unused. Only the value of connectorType impacts icons.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B sourceLogo(final String sourceLogo) {
this.sourceLogo = sourceLogo;
return self();
}
/**
* Subcategory of this connection.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B subCategory(final String subCategory) {
this.subCategory = subCategory;
return self();
}
/**
* Whether to upload to S3, GCP, or another storage location (true) or not (false).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B useObjectStorage(final Boolean useObjectStorage) {
this.useObjectStorage = useObjectStorage;
return self();
}
/**
* TBC
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B vectorEmbeddingsEnabled(final Boolean vectorEmbeddingsEnabled) {
this.vectorEmbeddingsEnabled = vectorEmbeddingsEnabled;
return self();
}
/**
* TBC
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B vectorEmbeddingsUpdatedAt(final Long vectorEmbeddingsUpdatedAt) {
this.vectorEmbeddingsUpdatedAt = vectorEmbeddingsUpdatedAt;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "Connection.ConnectionBuilder(super=" + super.toString() + ", typeName$value=" + this.typeName$value + ", allowQuery=" + this.allowQuery + ", allowQueryPreview=" + this.allowQueryPreview + ", category=" + this.category + ", connectionDbtEnvironments=" + this.connectionDbtEnvironments + ", connectionSSOCredentialGuid=" + this.connectionSSOCredentialGuid + ", connectorIcon=" + this.connectorIcon + ", connectorImage=" + this.connectorImage + ", credentialStrategy=" + this.credentialStrategy + ", defaultCredentialGuid=" + this.defaultCredentialGuid + ", hasPopularityInsights=" + this.hasPopularityInsights + ", host=" + this.host + ", isSampleDataPreviewEnabled=" + this.isSampleDataPreviewEnabled + ", objectStorageUploadThreshold=" + this.objectStorageUploadThreshold + ", policyStrategy=" + this.policyStrategy + ", policyStrategyForSamplePreview=" + this.policyStrategyForSamplePreview + ", popularityInsightsTimeframe=" + this.popularityInsightsTimeframe + ", port=" + this.port + ", previewCredentialStrategy=" + this.previewCredentialStrategy + ", queryConfig=" + this.queryConfig + ", queryPreviewConfig$key=" + this.queryPreviewConfig$key + ", queryPreviewConfig$value=" + this.queryPreviewConfig$value + ", queryTimeout=" + this.queryTimeout + ", queryUsernameStrategy=" + this.queryUsernameStrategy + ", rowLimit=" + this.rowLimit + ", sourceLogo=" + this.sourceLogo + ", subCategory=" + this.subCategory + ", useObjectStorage=" + this.useObjectStorage + ", vectorEmbeddingsEnabled=" + this.vectorEmbeddingsEnabled + ", vectorEmbeddingsUpdatedAt=" + this.vectorEmbeddingsUpdatedAt + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class ConnectionBuilderImpl extends Connection.ConnectionBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private ConnectionBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected Connection.ConnectionBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Connection build() {
return new Connection(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected Connection(final Connection.ConnectionBuilder, ?> b) {
super(b);
if (b.typeName$set) this.typeName = b.typeName$value;
else this.typeName = Connection.$default$typeName();
this.allowQuery = b.allowQuery;
this.allowQueryPreview = b.allowQueryPreview;
this.category = b.category;
java.util.SortedSet connectionDbtEnvironments = new java.util.TreeSet();
if (b.connectionDbtEnvironments != null) connectionDbtEnvironments.addAll(b.connectionDbtEnvironments);
connectionDbtEnvironments = java.util.Collections.unmodifiableSortedSet(connectionDbtEnvironments);
this.connectionDbtEnvironments = connectionDbtEnvironments;
this.connectionSSOCredentialGuid = b.connectionSSOCredentialGuid;
this.connectorIcon = b.connectorIcon;
this.connectorImage = b.connectorImage;
this.credentialStrategy = b.credentialStrategy;
this.defaultCredentialGuid = b.defaultCredentialGuid;
this.hasPopularityInsights = b.hasPopularityInsights;
this.host = b.host;
this.isSampleDataPreviewEnabled = b.isSampleDataPreviewEnabled;
this.objectStorageUploadThreshold = b.objectStorageUploadThreshold;
this.policyStrategy = b.policyStrategy;
this.policyStrategyForSamplePreview = b.policyStrategyForSamplePreview;
this.popularityInsightsTimeframe = b.popularityInsightsTimeframe;
this.port = b.port;
this.previewCredentialStrategy = b.previewCredentialStrategy;
this.queryConfig = b.queryConfig;
java.util.Map queryPreviewConfig;
switch (b.queryPreviewConfig$key == null ? 0 : b.queryPreviewConfig$key.size()) {
case 0:
queryPreviewConfig = java.util.Collections.emptyMap();
break;
case 1:
queryPreviewConfig = java.util.Collections.singletonMap(b.queryPreviewConfig$key.get(0), b.queryPreviewConfig$value.get(0));
break;
default:
queryPreviewConfig = new java.util.LinkedHashMap(b.queryPreviewConfig$key.size() < 1073741824 ? 1 + b.queryPreviewConfig$key.size() + (b.queryPreviewConfig$key.size() - 3) / 3 : java.lang.Integer.MAX_VALUE);
for (int $i = 0; $i < b.queryPreviewConfig$key.size(); $i++) queryPreviewConfig.put(b.queryPreviewConfig$key.get($i), (String) b.queryPreviewConfig$value.get($i));
queryPreviewConfig = java.util.Collections.unmodifiableMap(queryPreviewConfig);
}
this.queryPreviewConfig = queryPreviewConfig;
this.queryTimeout = b.queryTimeout;
this.queryUsernameStrategy = b.queryUsernameStrategy;
this.rowLimit = b.rowLimit;
this.sourceLogo = b.sourceLogo;
this.subCategory = b.subCategory;
this.useObjectStorage = b.useObjectStorage;
this.vectorEmbeddingsEnabled = b.vectorEmbeddingsEnabled;
this.vectorEmbeddingsUpdatedAt = b.vectorEmbeddingsUpdatedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static Connection.ConnectionBuilder, ?> _internal() {
return new Connection.ConnectionBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Connection.ConnectionBuilder, ?> toBuilder() {
return new Connection.ConnectionBuilderImpl().$fillValuesFrom(this);
}
/**
* Whether using this connection to run queries on the source is allowed (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAllowQuery() {
return this.allowQuery;
}
/**
* Whether using this connection to run preview queries on the source is allowed (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAllowQueryPreview() {
return this.allowQueryPreview;
}
/**
* Type of connection, for example WAREHOUSE, RDBMS, etc.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AtlanConnectionCategory getCategory() {
return this.category;
}
/**
* TBC
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SortedSet getConnectionDbtEnvironments() {
return this.connectionDbtEnvironments;
}
/**
* Unique identifier (GUID) for the SSO credentials to use for this connection.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getConnectionSSOCredentialGuid() {
return this.connectionSSOCredentialGuid;
}
/**
* Unused. Only the value of connectorType impacts icons.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getConnectorIcon() {
return this.connectorIcon;
}
/**
* Unused. Only the value of connectorType impacts icons.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getConnectorImage() {
return this.connectorImage;
}
/**
* Credential strategy to use for this connection for queries.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCredentialStrategy() {
return this.credentialStrategy;
}
/**
* Unique identifier (GUID) for the default credentials to use for this connection.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultCredentialGuid() {
return this.defaultCredentialGuid;
}
/**
* Whether this connection has popularity insights (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getHasPopularityInsights() {
return this.hasPopularityInsights;
}
/**
* Host name of this connection's source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHost() {
return this.host;
}
/**
* Whether sample data can be previewed for this connection (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getIsSampleDataPreviewEnabled() {
return this.isSampleDataPreviewEnabled;
}
/**
* Number of rows after which results should be uploaded to storage.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getObjectStorageUploadThreshold() {
return this.objectStorageUploadThreshold;
}
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten, applicable for stream api call made from insight screen
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPolicyStrategy() {
return this.policyStrategy;
}
/**
* Policy strategy is a configuration that determines whether the Atlan policy will be applied to the results of insight queries and whether the query will be rewritten. policyStrategyForSamplePreview config is applicable for sample preview call from assets screen
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPolicyStrategyForSamplePreview() {
return this.policyStrategyForSamplePreview;
}
/**
* Number of days over which popularity is calculated, for example 30 days.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPopularityInsightsTimeframe() {
return this.popularityInsightsTimeframe;
}
/**
* Port number to this connection's source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getPort() {
return this.port;
}
/**
* Credential strategy to use for this connection for preview queries.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPreviewCredentialStrategy() {
return this.previewCredentialStrategy;
}
/**
* Query config for this connection.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getQueryConfig() {
return this.queryConfig;
}
/**
* Configuration for preview queries.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getQueryPreviewConfig() {
return this.queryPreviewConfig;
}
/**
* Maximum time a query should be allowed to run before timing out.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQueryTimeout() {
return this.queryTimeout;
}
/**
* Username strategy to use for this connection for queries.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public QueryUsernameStrategy getQueryUsernameStrategy() {
return this.queryUsernameStrategy;
}
/**
* Maximum number of rows that can be returned for the source.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRowLimit() {
return this.rowLimit;
}
/**
* Unused. Only the value of connectorType impacts icons.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSourceLogo() {
return this.sourceLogo;
}
/**
* Subcategory of this connection.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSubCategory() {
return this.subCategory;
}
/**
* Whether to upload to S3, GCP, or another storage location (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getUseObjectStorage() {
return this.useObjectStorage;
}
/**
* TBC
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getVectorEmbeddingsEnabled() {
return this.vectorEmbeddingsEnabled;
}
/**
* TBC
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getVectorEmbeddingsUpdatedAt() {
return this.vectorEmbeddingsUpdatedAt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Connection)) return false;
final Connection other = (Connection) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$allowQuery = this.getAllowQuery();
final java.lang.Object other$allowQuery = other.getAllowQuery();
if (this$allowQuery == null ? other$allowQuery != null : !this$allowQuery.equals(other$allowQuery)) return false;
final java.lang.Object this$allowQueryPreview = this.getAllowQueryPreview();
final java.lang.Object other$allowQueryPreview = other.getAllowQueryPreview();
if (this$allowQueryPreview == null ? other$allowQueryPreview != null : !this$allowQueryPreview.equals(other$allowQueryPreview)) return false;
final java.lang.Object this$hasPopularityInsights = this.getHasPopularityInsights();
final java.lang.Object other$hasPopularityInsights = other.getHasPopularityInsights();
if (this$hasPopularityInsights == null ? other$hasPopularityInsights != null : !this$hasPopularityInsights.equals(other$hasPopularityInsights)) return false;
final java.lang.Object this$isSampleDataPreviewEnabled = this.getIsSampleDataPreviewEnabled();
final java.lang.Object other$isSampleDataPreviewEnabled = other.getIsSampleDataPreviewEnabled();
if (this$isSampleDataPreviewEnabled == null ? other$isSampleDataPreviewEnabled != null : !this$isSampleDataPreviewEnabled.equals(other$isSampleDataPreviewEnabled)) return false;
final java.lang.Object this$objectStorageUploadThreshold = this.getObjectStorageUploadThreshold();
final java.lang.Object other$objectStorageUploadThreshold = other.getObjectStorageUploadThreshold();
if (this$objectStorageUploadThreshold == null ? other$objectStorageUploadThreshold != null : !this$objectStorageUploadThreshold.equals(other$objectStorageUploadThreshold)) return false;
final java.lang.Object this$popularityInsightsTimeframe = this.getPopularityInsightsTimeframe();
final java.lang.Object other$popularityInsightsTimeframe = other.getPopularityInsightsTimeframe();
if (this$popularityInsightsTimeframe == null ? other$popularityInsightsTimeframe != null : !this$popularityInsightsTimeframe.equals(other$popularityInsightsTimeframe)) return false;
final java.lang.Object this$port = this.getPort();
final java.lang.Object other$port = other.getPort();
if (this$port == null ? other$port != null : !this$port.equals(other$port)) return false;
final java.lang.Object this$queryTimeout = this.getQueryTimeout();
final java.lang.Object other$queryTimeout = other.getQueryTimeout();
if (this$queryTimeout == null ? other$queryTimeout != null : !this$queryTimeout.equals(other$queryTimeout)) return false;
final java.lang.Object this$rowLimit = this.getRowLimit();
final java.lang.Object other$rowLimit = other.getRowLimit();
if (this$rowLimit == null ? other$rowLimit != null : !this$rowLimit.equals(other$rowLimit)) return false;
final java.lang.Object this$useObjectStorage = this.getUseObjectStorage();
final java.lang.Object other$useObjectStorage = other.getUseObjectStorage();
if (this$useObjectStorage == null ? other$useObjectStorage != null : !this$useObjectStorage.equals(other$useObjectStorage)) return false;
final java.lang.Object this$vectorEmbeddingsEnabled = this.getVectorEmbeddingsEnabled();
final java.lang.Object other$vectorEmbeddingsEnabled = other.getVectorEmbeddingsEnabled();
if (this$vectorEmbeddingsEnabled == null ? other$vectorEmbeddingsEnabled != null : !this$vectorEmbeddingsEnabled.equals(other$vectorEmbeddingsEnabled)) return false;
final java.lang.Object this$vectorEmbeddingsUpdatedAt = this.getVectorEmbeddingsUpdatedAt();
final java.lang.Object other$vectorEmbeddingsUpdatedAt = other.getVectorEmbeddingsUpdatedAt();
if (this$vectorEmbeddingsUpdatedAt == null ? other$vectorEmbeddingsUpdatedAt != null : !this$vectorEmbeddingsUpdatedAt.equals(other$vectorEmbeddingsUpdatedAt)) return false;
final java.lang.Object this$typeName = this.getTypeName();
final java.lang.Object other$typeName = other.getTypeName();
if (this$typeName == null ? other$typeName != null : !this$typeName.equals(other$typeName)) return false;
final java.lang.Object this$category = this.getCategory();
final java.lang.Object other$category = other.getCategory();
if (this$category == null ? other$category != null : !this$category.equals(other$category)) return false;
final java.lang.Object this$connectionDbtEnvironments = this.getConnectionDbtEnvironments();
final java.lang.Object other$connectionDbtEnvironments = other.getConnectionDbtEnvironments();
if (this$connectionDbtEnvironments == null ? other$connectionDbtEnvironments != null : !this$connectionDbtEnvironments.equals(other$connectionDbtEnvironments)) return false;
final java.lang.Object this$connectionSSOCredentialGuid = this.getConnectionSSOCredentialGuid();
final java.lang.Object other$connectionSSOCredentialGuid = other.getConnectionSSOCredentialGuid();
if (this$connectionSSOCredentialGuid == null ? other$connectionSSOCredentialGuid != null : !this$connectionSSOCredentialGuid.equals(other$connectionSSOCredentialGuid)) return false;
final java.lang.Object this$connectorIcon = this.getConnectorIcon();
final java.lang.Object other$connectorIcon = other.getConnectorIcon();
if (this$connectorIcon == null ? other$connectorIcon != null : !this$connectorIcon.equals(other$connectorIcon)) return false;
final java.lang.Object this$connectorImage = this.getConnectorImage();
final java.lang.Object other$connectorImage = other.getConnectorImage();
if (this$connectorImage == null ? other$connectorImage != null : !this$connectorImage.equals(other$connectorImage)) return false;
final java.lang.Object this$credentialStrategy = this.getCredentialStrategy();
final java.lang.Object other$credentialStrategy = other.getCredentialStrategy();
if (this$credentialStrategy == null ? other$credentialStrategy != null : !this$credentialStrategy.equals(other$credentialStrategy)) return false;
final java.lang.Object this$defaultCredentialGuid = this.getDefaultCredentialGuid();
final java.lang.Object other$defaultCredentialGuid = other.getDefaultCredentialGuid();
if (this$defaultCredentialGuid == null ? other$defaultCredentialGuid != null : !this$defaultCredentialGuid.equals(other$defaultCredentialGuid)) return false;
final java.lang.Object this$host = this.getHost();
final java.lang.Object other$host = other.getHost();
if (this$host == null ? other$host != null : !this$host.equals(other$host)) return false;
final java.lang.Object this$policyStrategy = this.getPolicyStrategy();
final java.lang.Object other$policyStrategy = other.getPolicyStrategy();
if (this$policyStrategy == null ? other$policyStrategy != null : !this$policyStrategy.equals(other$policyStrategy)) return false;
final java.lang.Object this$policyStrategyForSamplePreview = this.getPolicyStrategyForSamplePreview();
final java.lang.Object other$policyStrategyForSamplePreview = other.getPolicyStrategyForSamplePreview();
if (this$policyStrategyForSamplePreview == null ? other$policyStrategyForSamplePreview != null : !this$policyStrategyForSamplePreview.equals(other$policyStrategyForSamplePreview)) return false;
final java.lang.Object this$previewCredentialStrategy = this.getPreviewCredentialStrategy();
final java.lang.Object other$previewCredentialStrategy = other.getPreviewCredentialStrategy();
if (this$previewCredentialStrategy == null ? other$previewCredentialStrategy != null : !this$previewCredentialStrategy.equals(other$previewCredentialStrategy)) return false;
final java.lang.Object this$queryConfig = this.getQueryConfig();
final java.lang.Object other$queryConfig = other.getQueryConfig();
if (this$queryConfig == null ? other$queryConfig != null : !this$queryConfig.equals(other$queryConfig)) return false;
final java.lang.Object this$queryPreviewConfig = this.getQueryPreviewConfig();
final java.lang.Object other$queryPreviewConfig = other.getQueryPreviewConfig();
if (this$queryPreviewConfig == null ? other$queryPreviewConfig != null : !this$queryPreviewConfig.equals(other$queryPreviewConfig)) return false;
final java.lang.Object this$queryUsernameStrategy = this.getQueryUsernameStrategy();
final java.lang.Object other$queryUsernameStrategy = other.getQueryUsernameStrategy();
if (this$queryUsernameStrategy == null ? other$queryUsernameStrategy != null : !this$queryUsernameStrategy.equals(other$queryUsernameStrategy)) return false;
final java.lang.Object this$sourceLogo = this.getSourceLogo();
final java.lang.Object other$sourceLogo = other.getSourceLogo();
if (this$sourceLogo == null ? other$sourceLogo != null : !this$sourceLogo.equals(other$sourceLogo)) return false;
final java.lang.Object this$subCategory = this.getSubCategory();
final java.lang.Object other$subCategory = other.getSubCategory();
if (this$subCategory == null ? other$subCategory != null : !this$subCategory.equals(other$subCategory)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Connection;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final java.lang.Object $allowQuery = this.getAllowQuery();
result = result * PRIME + ($allowQuery == null ? 43 : $allowQuery.hashCode());
final java.lang.Object $allowQueryPreview = this.getAllowQueryPreview();
result = result * PRIME + ($allowQueryPreview == null ? 43 : $allowQueryPreview.hashCode());
final java.lang.Object $hasPopularityInsights = this.getHasPopularityInsights();
result = result * PRIME + ($hasPopularityInsights == null ? 43 : $hasPopularityInsights.hashCode());
final java.lang.Object $isSampleDataPreviewEnabled = this.getIsSampleDataPreviewEnabled();
result = result * PRIME + ($isSampleDataPreviewEnabled == null ? 43 : $isSampleDataPreviewEnabled.hashCode());
final java.lang.Object $objectStorageUploadThreshold = this.getObjectStorageUploadThreshold();
result = result * PRIME + ($objectStorageUploadThreshold == null ? 43 : $objectStorageUploadThreshold.hashCode());
final java.lang.Object $popularityInsightsTimeframe = this.getPopularityInsightsTimeframe();
result = result * PRIME + ($popularityInsightsTimeframe == null ? 43 : $popularityInsightsTimeframe.hashCode());
final java.lang.Object $port = this.getPort();
result = result * PRIME + ($port == null ? 43 : $port.hashCode());
final java.lang.Object $queryTimeout = this.getQueryTimeout();
result = result * PRIME + ($queryTimeout == null ? 43 : $queryTimeout.hashCode());
final java.lang.Object $rowLimit = this.getRowLimit();
result = result * PRIME + ($rowLimit == null ? 43 : $rowLimit.hashCode());
final java.lang.Object $useObjectStorage = this.getUseObjectStorage();
result = result * PRIME + ($useObjectStorage == null ? 43 : $useObjectStorage.hashCode());
final java.lang.Object $vectorEmbeddingsEnabled = this.getVectorEmbeddingsEnabled();
result = result * PRIME + ($vectorEmbeddingsEnabled == null ? 43 : $vectorEmbeddingsEnabled.hashCode());
final java.lang.Object $vectorEmbeddingsUpdatedAt = this.getVectorEmbeddingsUpdatedAt();
result = result * PRIME + ($vectorEmbeddingsUpdatedAt == null ? 43 : $vectorEmbeddingsUpdatedAt.hashCode());
final java.lang.Object $typeName = this.getTypeName();
result = result * PRIME + ($typeName == null ? 43 : $typeName.hashCode());
final java.lang.Object $category = this.getCategory();
result = result * PRIME + ($category == null ? 43 : $category.hashCode());
final java.lang.Object $connectionDbtEnvironments = this.getConnectionDbtEnvironments();
result = result * PRIME + ($connectionDbtEnvironments == null ? 43 : $connectionDbtEnvironments.hashCode());
final java.lang.Object $connectionSSOCredentialGuid = this.getConnectionSSOCredentialGuid();
result = result * PRIME + ($connectionSSOCredentialGuid == null ? 43 : $connectionSSOCredentialGuid.hashCode());
final java.lang.Object $connectorIcon = this.getConnectorIcon();
result = result * PRIME + ($connectorIcon == null ? 43 : $connectorIcon.hashCode());
final java.lang.Object $connectorImage = this.getConnectorImage();
result = result * PRIME + ($connectorImage == null ? 43 : $connectorImage.hashCode());
final java.lang.Object $credentialStrategy = this.getCredentialStrategy();
result = result * PRIME + ($credentialStrategy == null ? 43 : $credentialStrategy.hashCode());
final java.lang.Object $defaultCredentialGuid = this.getDefaultCredentialGuid();
result = result * PRIME + ($defaultCredentialGuid == null ? 43 : $defaultCredentialGuid.hashCode());
final java.lang.Object $host = this.getHost();
result = result * PRIME + ($host == null ? 43 : $host.hashCode());
final java.lang.Object $policyStrategy = this.getPolicyStrategy();
result = result * PRIME + ($policyStrategy == null ? 43 : $policyStrategy.hashCode());
final java.lang.Object $policyStrategyForSamplePreview = this.getPolicyStrategyForSamplePreview();
result = result * PRIME + ($policyStrategyForSamplePreview == null ? 43 : $policyStrategyForSamplePreview.hashCode());
final java.lang.Object $previewCredentialStrategy = this.getPreviewCredentialStrategy();
result = result * PRIME + ($previewCredentialStrategy == null ? 43 : $previewCredentialStrategy.hashCode());
final java.lang.Object $queryConfig = this.getQueryConfig();
result = result * PRIME + ($queryConfig == null ? 43 : $queryConfig.hashCode());
final java.lang.Object $queryPreviewConfig = this.getQueryPreviewConfig();
result = result * PRIME + ($queryPreviewConfig == null ? 43 : $queryPreviewConfig.hashCode());
final java.lang.Object $queryUsernameStrategy = this.getQueryUsernameStrategy();
result = result * PRIME + ($queryUsernameStrategy == null ? 43 : $queryUsernameStrategy.hashCode());
final java.lang.Object $sourceLogo = this.getSourceLogo();
result = result * PRIME + ($sourceLogo == null ? 43 : $sourceLogo.hashCode());
final java.lang.Object $subCategory = this.getSubCategory();
result = result * PRIME + ($subCategory == null ? 43 : $subCategory.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "Connection(super=" + super.toString() + ", typeName=" + this.getTypeName() + ", allowQuery=" + this.getAllowQuery() + ", allowQueryPreview=" + this.getAllowQueryPreview() + ", category=" + this.getCategory() + ", connectionDbtEnvironments=" + this.getConnectionDbtEnvironments() + ", connectionSSOCredentialGuid=" + this.getConnectionSSOCredentialGuid() + ", connectorIcon=" + this.getConnectorIcon() + ", connectorImage=" + this.getConnectorImage() + ", credentialStrategy=" + this.getCredentialStrategy() + ", defaultCredentialGuid=" + this.getDefaultCredentialGuid() + ", hasPopularityInsights=" + this.getHasPopularityInsights() + ", host=" + this.getHost() + ", isSampleDataPreviewEnabled=" + this.getIsSampleDataPreviewEnabled() + ", objectStorageUploadThreshold=" + this.getObjectStorageUploadThreshold() + ", policyStrategy=" + this.getPolicyStrategy() + ", policyStrategyForSamplePreview=" + this.getPolicyStrategyForSamplePreview() + ", popularityInsightsTimeframe=" + this.getPopularityInsightsTimeframe() + ", port=" + this.getPort() + ", previewCredentialStrategy=" + this.getPreviewCredentialStrategy() + ", queryConfig=" + this.getQueryConfig() + ", queryPreviewConfig=" + this.getQueryPreviewConfig() + ", queryTimeout=" + this.getQueryTimeout() + ", queryUsernameStrategy=" + this.getQueryUsernameStrategy() + ", rowLimit=" + this.getRowLimit() + ", sourceLogo=" + this.getSourceLogo() + ", subCategory=" + this.getSubCategory() + ", useObjectStorage=" + this.getUseObjectStorage() + ", vectorEmbeddingsEnabled=" + this.getVectorEmbeddingsEnabled() + ", vectorEmbeddingsUpdatedAt=" + this.getVectorEmbeddingsUpdatedAt() + ")";
}
/**
* Fixed typeName for Connections.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTypeName() {
return this.typeName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy