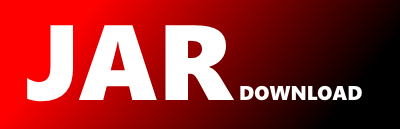
com.atlan.model.relations.RelationshipAttributes Maven / Gradle / Ivy
// Generated by delombok at Thu Oct 10 18:56:32 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.relations;
import com.atlan.model.core.AtlanObject;
import com.atlan.serde.RelationshipAttributesDeserializer;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
@JsonDeserialize(using = RelationshipAttributesDeserializer.class)
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.EXISTING_PROPERTY, property = "typeName", defaultImpl = IndistinctRelationship.class)
public abstract class RelationshipAttributes extends AtlanObject {
private static final long serialVersionUID = 2L;
/**
* Name of the relationship type that defines the relationship.
*/
String typeName;
/**
* Internal tracking of fields that should be serialized with null values.
*/
@JsonIgnore
transient Set nullFields;
/**
* Retrieve the list of fields to be serialized with null values.
*/
@JsonIgnore
public Set getNullFields() {
if (nullFields == null) {
return Collections.emptySet();
}
return Collections.unmodifiableSet(nullFields);
}
@JsonIgnore
public abstract Map getAll();
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class RelationshipAttributesBuilder> extends AtlanObject.AtlanObjectBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String typeName;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private java.util.ArrayList nullFields;
/**
* Name of the relationship type that defines the relationship.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B typeName(final String typeName) {
this.typeName = typeName;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B nullField(final String nullField) {
if (this.nullFields == null) this.nullFields = new java.util.ArrayList();
this.nullFields.add(nullField);
return self();
}
@JsonIgnore
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B nullFields(final java.util.Collection extends String> nullFields) {
if (nullFields == null) {
throw new java.lang.NullPointerException("nullFields cannot be null");
}
if (this.nullFields == null) this.nullFields = new java.util.ArrayList();
this.nullFields.addAll(nullFields);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B clearNullFields() {
if (this.nullFields != null) this.nullFields.clear();
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "RelationshipAttributes.RelationshipAttributesBuilder(super=" + super.toString() + ", typeName=" + this.typeName + ", nullFields=" + this.nullFields + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected RelationshipAttributes(final RelationshipAttributes.RelationshipAttributesBuilder, ?> b) {
super(b);
this.typeName = b.typeName;
java.util.Set nullFields;
switch (b.nullFields == null ? 0 : b.nullFields.size()) {
case 0:
nullFields = java.util.Collections.emptySet();
break;
case 1:
nullFields = java.util.Collections.singleton(b.nullFields.get(0));
break;
default:
nullFields = new java.util.LinkedHashSet(b.nullFields.size() < 1073741824 ? 1 + b.nullFields.size() + (b.nullFields.size() - 3) / 3 : java.lang.Integer.MAX_VALUE);
nullFields.addAll(b.nullFields);
nullFields = java.util.Collections.unmodifiableSet(nullFields);
}
this.nullFields = nullFields;
}
/**
* Name of the relationship type that defines the relationship.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTypeName() {
return this.typeName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof RelationshipAttributes)) return false;
final RelationshipAttributes other = (RelationshipAttributes) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$typeName = this.getTypeName();
final java.lang.Object other$typeName = other.getTypeName();
if (this$typeName == null ? other$typeName != null : !this$typeName.equals(other$typeName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof RelationshipAttributes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $typeName = this.getTypeName();
result = result * PRIME + ($typeName == null ? 43 : $typeName.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "RelationshipAttributes(super=" + super.toString() + ", typeName=" + this.getTypeName() + ", nullFields=" + this.getNullFields() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy