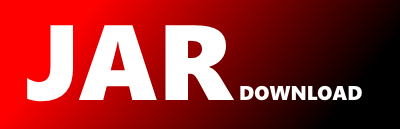
com.atlan.api.AbstractEndpoint Maven / Gradle / Ivy
// Generated by delombok at Wed Oct 16 22:16:02 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2023 Atlan Pte. Ltd. */
package com.atlan.api;
import com.atlan.AtlanClient;
import com.atlan.exception.ApiConnectionException;
import com.atlan.net.ApiResource;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import java.io.IOException;
public abstract class AbstractEndpoint {
protected final AtlanClient client;
protected AbstractEndpoint(AtlanClient client) {
this.client = client;
}
/**
* Retrieve the base URL to use to access the endpoint.
*
* @param service the internal service used to access the endpoint
* @param prefix the prefix used for routing external access to the endpoint
* @return the base URL (including any prefix) to use to access the API endpoint
* @throws ApiConnectionException if no base URL has been defined, and the SDK has not been configured for internal access
*/
protected String getBaseUrl(String service, String prefix) throws ApiConnectionException {
return client.isInternal() ? service : client.getBaseUrl() + prefix;
}
/**
* Utility class for introspecting raw responses from API endpoints.
*/
@JsonDeserialize(using = RawResponseDeserializer.class)
public static final class RawResponse extends ApiResource {
private static final long serialVersionUID = 2L;
String payload;
public RawResponse(String payload) {
this.payload = payload;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPayload() {
return this.payload;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AbstractEndpoint.RawResponse)) return false;
final AbstractEndpoint.RawResponse other = (AbstractEndpoint.RawResponse) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$payload = this.getPayload();
final java.lang.Object other$payload = other.getPayload();
if (this$payload == null ? other$payload != null : !this$payload.equals(other$payload)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof AbstractEndpoint.RawResponse;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $payload = this.getPayload();
result = result * PRIME + ($payload == null ? 43 : $payload.hashCode());
return result;
}
}
private static class RawResponseDeserializer extends StdDeserializer {
private static final long serialVersionUID = 2L;
public RawResponseDeserializer() {
this(RawResponse.class);
}
public RawResponseDeserializer(Class> t) {
super(t);
}
/**
* {@inheritDoc}
*/
@Override
public RawResponse deserialize(JsonParser parser, DeserializationContext context) throws IOException {
return new RawResponse(parser.getCodec().readTree(parser).toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy