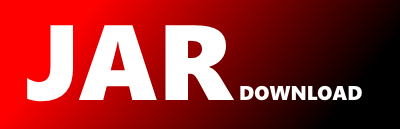
com.atlan.model.core.AtlanObject Maven / Gradle / Ivy
// Generated by delombok at Wed Oct 16 22:16:04 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.core;
/* Based on original code from https://github.com/stripe/stripe-java (under MIT license) */
import com.atlan.AtlanClient;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.databind.JsonNode;
import java.io.IOException;
import java.io.Serializable;
public abstract class AtlanObject implements Serializable {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(AtlanObject.class);
private static final long serialVersionUID = 2L;
@JsonIgnore
protected transient JsonNode rawJsonObject;
public AtlanObject() {
// Do nothing - needed for Lombok SuperBuilder generations...
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return String.format("<%s#%s>", this.getClass().getName(), this.hashCode());
}
/**
* Serialize the object to a JSON string.
* @param client the client through which to serialize the object
* @return the serialized JSON string
*/
public String toJson(AtlanClient client) {
try {
return client.writeValueAsString(this);
} catch (IOException e) {
log.error("Unable to serialize this object: {}", this.getClass().getName(), e);
}
return null;
}
/**
* Returns the raw JsonNode exposed by the Jackson library. This can be used to access properties
* that are not directly exposed by Atlan's Java library.
*
* Note: You should always prefer using the standard property accessors whenever possible.
* Because this method exposes Jackson's underlying API, it is not considered fully stable. Atlan's
* Java library might move off Jackson in the future and this method would be removed or change
* significantly.
*
* @return The raw JsonNode.
*/
@JsonIgnore
public JsonNode getRawJsonObject() {
return rawJsonObject;
}
/**
* Sets the raw response from the API. This is used to expose properties that are not
* directly exposed by Atlan's Java library.
*
* @param rawJsonObject the raw JSON from the API response
*/
@JsonIgnore
public void setRawJsonObject(JsonNode rawJsonObject) {
this.rawJsonObject = rawJsonObject;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class AtlanObjectBuilder> {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private JsonNode rawJsonObject;
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected B $fillValuesFrom(final C instance) {
AtlanObject.AtlanObjectBuilder.$fillValuesFromInstanceIntoBuilder(instance, this);
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static void $fillValuesFromInstanceIntoBuilder(final AtlanObject instance, final AtlanObject.AtlanObjectBuilder, ?> b) {
b.rawJsonObject(instance.rawJsonObject);
}
/**
* @return {@code this}.
*/
@JsonIgnore
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B rawJsonObject(final JsonNode rawJsonObject) {
this.rawJsonObject = rawJsonObject;
return self();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "AtlanObject.AtlanObjectBuilder(rawJsonObject=" + this.rawJsonObject + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected AtlanObject(final AtlanObject.AtlanObjectBuilder, ?> b) {
this.rawJsonObject = b.rawJsonObject;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AtlanObject)) return false;
final AtlanObject other = (AtlanObject) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof AtlanObject;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy