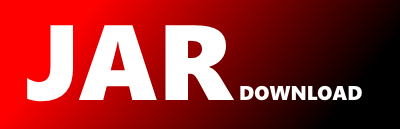
com.atlan.model.search.SearchLogEntry Maven / Gradle / Ivy
// Generated by delombok at Wed Oct 16 22:16:04 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.search;
import com.atlan.model.core.AtlanObject;
import com.atlan.model.fields.BooleanField;
import com.atlan.model.fields.KeywordField;
import com.atlan.model.fields.NumericField;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import lombok.*;
/**
* Base class for entries in the search log.
*/
@SuppressWarnings("cast")
public class SearchLogEntry extends AtlanObject {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(SearchLogEntry.class);
private static final long serialVersionUID = 2L;
private static final String UNMAPPED = "__NON_ASSET__";
/**
* Details of the browser or other client used to make the request.
*/
public static final KeywordField USER_AGENT = new KeywordField(UNMAPPED, "userAgent");
/**
* Hostname of the tenant against which the search was run.
*/
public static final KeywordField HOST = new KeywordField(UNMAPPED, "host");
/**
* IP address from which the search was run.
*/
public static final KeywordField IP_ADDRESS = new KeywordField(UNMAPPED, "ipAddress");
/**
* Username of the user who ran the search.
*/
public static final KeywordField USER = new KeywordField(UNMAPPED, "userName");
/**
* GUID(s) of asset(s) that were in the results of the search.
*/
public static final KeywordField ENTITY_ID = new KeywordField("guid", "entityGuidsAll");
/**
* Unique name(s) of asset(s) that were in the results of the search.
*/
public static final KeywordField QUALIFIED_NAME = new KeywordField("qualifiedName", "entityQFNamesAll");
/**
* Name(s) of the types of assets that were in the results of the search.
*/
public static final KeywordField TYPE_NAME = new KeywordField("typeName", "entityTypeNamesAll");
/**
* Tag(s) that were sent in the search request.
*/
public static final KeywordField UTM_TAGS = new KeywordField(UNMAPPED, "utmTags");
/**
* Text a user entered for their search.
*/
public static final KeywordField SEARCH_INPUT = new KeywordField(UNMAPPED, "searchInput");
/**
* Whether the search had any results (true) or not (false).
*/
public static final BooleanField HAS_RESULT = new BooleanField(UNMAPPED, "hasResult");
/**
* Number of results for the search.
*/
public static final NumericField RESULTS_COUNT = new NumericField(UNMAPPED, "resultsCount");
/**
* Elapsed time to produce the results for the search, in milliseconds.
*/
public static final NumericField RESPONSE_TIME = new NumericField(UNMAPPED, "responseTime");
/**
* Time (epoch-style) at which the search was logged, in milliseconds.
*/
public static final NumericField LOGGED_AT = new NumericField(UNMAPPED, "createdAt");
/**
* Time (epoch-style) at which the search was initiated, in milliseconds.
*/
public static final NumericField SEARCHED_AT = new NumericField(UNMAPPED, "timestamp");
/**
* Whether the search was successful (false) or not (true).
*/
public static final BooleanField FAILED = new BooleanField(UNMAPPED, "failed");
/**
* Details of the browser or other client used to make the request.
*/
String userAgent;
/**
* Hostname of the tenant against which the search was run.
*/
String host;
/**
* IP address from which the search was run.
*/
String ipAddress;
/**
* Username of the user who ran the search.
*/
String userName;
/**
* List of all GUIDs for assets that were results of the search.
*/
@JsonProperty("entityGuidsAll")
List resultGuids;
/**
* List of all qualifiedNames for assets that were results of the search.
*/
@JsonProperty("entityQFNamesAll")
List resultQualifiedNames;
/**
* List of all GUIDs for assets that were results of the search, that the user is permitted to see.
*/
@JsonProperty("entityGuidsAllowed")
List resultGuidsAllowed;
/**
* List of all qualifiedNames for assets that were results of the search, that the user is permitted to see.
*/
@JsonProperty("entityQFNamesAllowed")
List resultQualifiedNamesAllowed;
/**
* List of all typeNames for assets that were results of the search.
*/
@JsonProperty("entityTypeNamesAll")
List resultTypeNames;
/**
* List of all typeNames for assets that were results of the search, that the user is permitted to see.
*/
@JsonProperty("entityTypeNamesAllowed")
List resultTypeNamesAllowed;
/**
* List of the UTM tags that were sent in the search request.
*/
List utmTags;
/**
* Text a user entered for their search.
*/
String searchInput;
/**
* Whether the search had any results (true) or not (false).
*/
Boolean hasResult;
/**
* Number of results for the search.
*/
Long resultsCount;
/**
* Elapsed time to produce the results for the search, in milliseconds.
*/
Long responseTime;
/**
* Time (epoch-style) at which the search was logged, in milliseconds.
*/
Long createdAt;
/**
* Time (epoch-style) at which the search was run, in milliseconds.
*/
Long timestamp;
/**
* Whether the search was successful (false) or not (true).
*/
Boolean failed;
/**
* DSL of the full search request that was made.
*/
@JsonProperty("request.dsl")
IndexSearchDSL requestDsl;
/**
* DSL of the full search request that was made, as a string.
*/
@JsonProperty("request.dslText")
String requestDslText;
/**
* List of attribute (names) that were requested as part of the search.
*/
@JsonProperty("request.attributes")
List requestAttributes;
/**
* List of relationship attribute (names) that were requested as part of the search.
*/
@JsonProperty("request.relationAttributes")
List requestRelationAttributes;
/**
* Details of the browser or other client used to make the request.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserAgent() {
return this.userAgent;
}
/**
* Hostname of the tenant against which the search was run.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHost() {
return this.host;
}
/**
* IP address from which the search was run.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIpAddress() {
return this.ipAddress;
}
/**
* Username of the user who ran the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserName() {
return this.userName;
}
/**
* List of all GUIDs for assets that were results of the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultGuids() {
return this.resultGuids;
}
/**
* List of all qualifiedNames for assets that were results of the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultQualifiedNames() {
return this.resultQualifiedNames;
}
/**
* List of all GUIDs for assets that were results of the search, that the user is permitted to see.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultGuidsAllowed() {
return this.resultGuidsAllowed;
}
/**
* List of all qualifiedNames for assets that were results of the search, that the user is permitted to see.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultQualifiedNamesAllowed() {
return this.resultQualifiedNamesAllowed;
}
/**
* List of all typeNames for assets that were results of the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultTypeNames() {
return this.resultTypeNames;
}
/**
* List of all typeNames for assets that were results of the search, that the user is permitted to see.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getResultTypeNamesAllowed() {
return this.resultTypeNamesAllowed;
}
/**
* List of the UTM tags that were sent in the search request.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getUtmTags() {
return this.utmTags;
}
/**
* Text a user entered for their search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSearchInput() {
return this.searchInput;
}
/**
* Whether the search had any results (true) or not (false).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getHasResult() {
return this.hasResult;
}
/**
* Number of results for the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getResultsCount() {
return this.resultsCount;
}
/**
* Elapsed time to produce the results for the search, in milliseconds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getResponseTime() {
return this.responseTime;
}
/**
* Time (epoch-style) at which the search was logged, in milliseconds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreatedAt() {
return this.createdAt;
}
/**
* Time (epoch-style) at which the search was run, in milliseconds.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTimestamp() {
return this.timestamp;
}
/**
* Whether the search was successful (false) or not (true).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getFailed() {
return this.failed;
}
/**
* DSL of the full search request that was made.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public IndexSearchDSL getRequestDsl() {
return this.requestDsl;
}
/**
* DSL of the full search request that was made, as a string.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRequestDslText() {
return this.requestDslText;
}
/**
* List of attribute (names) that were requested as part of the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getRequestAttributes() {
return this.requestAttributes;
}
/**
* List of relationship attribute (names) that were requested as part of the search.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getRequestRelationAttributes() {
return this.requestRelationAttributes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SearchLogEntry)) return false;
final SearchLogEntry other = (SearchLogEntry) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$hasResult = this.getHasResult();
final java.lang.Object other$hasResult = other.getHasResult();
if (this$hasResult == null ? other$hasResult != null : !this$hasResult.equals(other$hasResult)) return false;
final java.lang.Object this$resultsCount = this.getResultsCount();
final java.lang.Object other$resultsCount = other.getResultsCount();
if (this$resultsCount == null ? other$resultsCount != null : !this$resultsCount.equals(other$resultsCount)) return false;
final java.lang.Object this$responseTime = this.getResponseTime();
final java.lang.Object other$responseTime = other.getResponseTime();
if (this$responseTime == null ? other$responseTime != null : !this$responseTime.equals(other$responseTime)) return false;
final java.lang.Object this$createdAt = this.getCreatedAt();
final java.lang.Object other$createdAt = other.getCreatedAt();
if (this$createdAt == null ? other$createdAt != null : !this$createdAt.equals(other$createdAt)) return false;
final java.lang.Object this$timestamp = this.getTimestamp();
final java.lang.Object other$timestamp = other.getTimestamp();
if (this$timestamp == null ? other$timestamp != null : !this$timestamp.equals(other$timestamp)) return false;
final java.lang.Object this$failed = this.getFailed();
final java.lang.Object other$failed = other.getFailed();
if (this$failed == null ? other$failed != null : !this$failed.equals(other$failed)) return false;
final java.lang.Object this$userAgent = this.getUserAgent();
final java.lang.Object other$userAgent = other.getUserAgent();
if (this$userAgent == null ? other$userAgent != null : !this$userAgent.equals(other$userAgent)) return false;
final java.lang.Object this$host = this.getHost();
final java.lang.Object other$host = other.getHost();
if (this$host == null ? other$host != null : !this$host.equals(other$host)) return false;
final java.lang.Object this$ipAddress = this.getIpAddress();
final java.lang.Object other$ipAddress = other.getIpAddress();
if (this$ipAddress == null ? other$ipAddress != null : !this$ipAddress.equals(other$ipAddress)) return false;
final java.lang.Object this$userName = this.getUserName();
final java.lang.Object other$userName = other.getUserName();
if (this$userName == null ? other$userName != null : !this$userName.equals(other$userName)) return false;
final java.lang.Object this$resultGuids = this.getResultGuids();
final java.lang.Object other$resultGuids = other.getResultGuids();
if (this$resultGuids == null ? other$resultGuids != null : !this$resultGuids.equals(other$resultGuids)) return false;
final java.lang.Object this$resultQualifiedNames = this.getResultQualifiedNames();
final java.lang.Object other$resultQualifiedNames = other.getResultQualifiedNames();
if (this$resultQualifiedNames == null ? other$resultQualifiedNames != null : !this$resultQualifiedNames.equals(other$resultQualifiedNames)) return false;
final java.lang.Object this$resultGuidsAllowed = this.getResultGuidsAllowed();
final java.lang.Object other$resultGuidsAllowed = other.getResultGuidsAllowed();
if (this$resultGuidsAllowed == null ? other$resultGuidsAllowed != null : !this$resultGuidsAllowed.equals(other$resultGuidsAllowed)) return false;
final java.lang.Object this$resultQualifiedNamesAllowed = this.getResultQualifiedNamesAllowed();
final java.lang.Object other$resultQualifiedNamesAllowed = other.getResultQualifiedNamesAllowed();
if (this$resultQualifiedNamesAllowed == null ? other$resultQualifiedNamesAllowed != null : !this$resultQualifiedNamesAllowed.equals(other$resultQualifiedNamesAllowed)) return false;
final java.lang.Object this$resultTypeNames = this.getResultTypeNames();
final java.lang.Object other$resultTypeNames = other.getResultTypeNames();
if (this$resultTypeNames == null ? other$resultTypeNames != null : !this$resultTypeNames.equals(other$resultTypeNames)) return false;
final java.lang.Object this$resultTypeNamesAllowed = this.getResultTypeNamesAllowed();
final java.lang.Object other$resultTypeNamesAllowed = other.getResultTypeNamesAllowed();
if (this$resultTypeNamesAllowed == null ? other$resultTypeNamesAllowed != null : !this$resultTypeNamesAllowed.equals(other$resultTypeNamesAllowed)) return false;
final java.lang.Object this$utmTags = this.getUtmTags();
final java.lang.Object other$utmTags = other.getUtmTags();
if (this$utmTags == null ? other$utmTags != null : !this$utmTags.equals(other$utmTags)) return false;
final java.lang.Object this$searchInput = this.getSearchInput();
final java.lang.Object other$searchInput = other.getSearchInput();
if (this$searchInput == null ? other$searchInput != null : !this$searchInput.equals(other$searchInput)) return false;
final java.lang.Object this$requestDsl = this.getRequestDsl();
final java.lang.Object other$requestDsl = other.getRequestDsl();
if (this$requestDsl == null ? other$requestDsl != null : !this$requestDsl.equals(other$requestDsl)) return false;
final java.lang.Object this$requestDslText = this.getRequestDslText();
final java.lang.Object other$requestDslText = other.getRequestDslText();
if (this$requestDslText == null ? other$requestDslText != null : !this$requestDslText.equals(other$requestDslText)) return false;
final java.lang.Object this$requestAttributes = this.getRequestAttributes();
final java.lang.Object other$requestAttributes = other.getRequestAttributes();
if (this$requestAttributes == null ? other$requestAttributes != null : !this$requestAttributes.equals(other$requestAttributes)) return false;
final java.lang.Object this$requestRelationAttributes = this.getRequestRelationAttributes();
final java.lang.Object other$requestRelationAttributes = other.getRequestRelationAttributes();
if (this$requestRelationAttributes == null ? other$requestRelationAttributes != null : !this$requestRelationAttributes.equals(other$requestRelationAttributes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SearchLogEntry;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final java.lang.Object $hasResult = this.getHasResult();
result = result * PRIME + ($hasResult == null ? 43 : $hasResult.hashCode());
final java.lang.Object $resultsCount = this.getResultsCount();
result = result * PRIME + ($resultsCount == null ? 43 : $resultsCount.hashCode());
final java.lang.Object $responseTime = this.getResponseTime();
result = result * PRIME + ($responseTime == null ? 43 : $responseTime.hashCode());
final java.lang.Object $createdAt = this.getCreatedAt();
result = result * PRIME + ($createdAt == null ? 43 : $createdAt.hashCode());
final java.lang.Object $timestamp = this.getTimestamp();
result = result * PRIME + ($timestamp == null ? 43 : $timestamp.hashCode());
final java.lang.Object $failed = this.getFailed();
result = result * PRIME + ($failed == null ? 43 : $failed.hashCode());
final java.lang.Object $userAgent = this.getUserAgent();
result = result * PRIME + ($userAgent == null ? 43 : $userAgent.hashCode());
final java.lang.Object $host = this.getHost();
result = result * PRIME + ($host == null ? 43 : $host.hashCode());
final java.lang.Object $ipAddress = this.getIpAddress();
result = result * PRIME + ($ipAddress == null ? 43 : $ipAddress.hashCode());
final java.lang.Object $userName = this.getUserName();
result = result * PRIME + ($userName == null ? 43 : $userName.hashCode());
final java.lang.Object $resultGuids = this.getResultGuids();
result = result * PRIME + ($resultGuids == null ? 43 : $resultGuids.hashCode());
final java.lang.Object $resultQualifiedNames = this.getResultQualifiedNames();
result = result * PRIME + ($resultQualifiedNames == null ? 43 : $resultQualifiedNames.hashCode());
final java.lang.Object $resultGuidsAllowed = this.getResultGuidsAllowed();
result = result * PRIME + ($resultGuidsAllowed == null ? 43 : $resultGuidsAllowed.hashCode());
final java.lang.Object $resultQualifiedNamesAllowed = this.getResultQualifiedNamesAllowed();
result = result * PRIME + ($resultQualifiedNamesAllowed == null ? 43 : $resultQualifiedNamesAllowed.hashCode());
final java.lang.Object $resultTypeNames = this.getResultTypeNames();
result = result * PRIME + ($resultTypeNames == null ? 43 : $resultTypeNames.hashCode());
final java.lang.Object $resultTypeNamesAllowed = this.getResultTypeNamesAllowed();
result = result * PRIME + ($resultTypeNamesAllowed == null ? 43 : $resultTypeNamesAllowed.hashCode());
final java.lang.Object $utmTags = this.getUtmTags();
result = result * PRIME + ($utmTags == null ? 43 : $utmTags.hashCode());
final java.lang.Object $searchInput = this.getSearchInput();
result = result * PRIME + ($searchInput == null ? 43 : $searchInput.hashCode());
final java.lang.Object $requestDsl = this.getRequestDsl();
result = result * PRIME + ($requestDsl == null ? 43 : $requestDsl.hashCode());
final java.lang.Object $requestDslText = this.getRequestDslText();
result = result * PRIME + ($requestDslText == null ? 43 : $requestDslText.hashCode());
final java.lang.Object $requestAttributes = this.getRequestAttributes();
result = result * PRIME + ($requestAttributes == null ? 43 : $requestAttributes.hashCode());
final java.lang.Object $requestRelationAttributes = this.getRequestRelationAttributes();
result = result * PRIME + ($requestRelationAttributes == null ? 43 : $requestRelationAttributes.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy