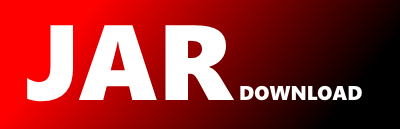
com.atlan.model.search.SuggestionResponse Maven / Gradle / Ivy
// Generated by delombok at Wed Oct 16 22:16:04 UTC 2024
/* SPDX-License-Identifier: Apache-2.0
Copyright 2022 Atlan Pte. Ltd. */
package com.atlan.model.search;
import com.atlan.model.assets.GlossaryTerm;
import com.atlan.net.ApiResource;
import java.util.List;
/**
* Captures the response from a search for suggestions on an asset's metadata against Atlan.
*/
public class SuggestionResponse extends ApiResource {
private static final long serialVersionUID = 2L;
/**
* Suggested system-level descriptions.
*/
List systemDescriptions;
/**
* Suggested user-provided descriptions.
*/
List userDescriptions;
/**
* Suggested individual user owners.
*/
List ownerUsers;
/**
* Suggested group owners.
*/
List ownerGroups;
/**
* Suggested tags.
*/
List atlanTags;
/**
* Suggested terms.
*/
List assignedTerms;
public static final class SuggestedItem {
/**
* Number of other assets on which the suggestion appears.
*/
long count;
/**
* Value of the suggestion.
*/
String value;
public SuggestedItem(long count, String value) {
this.count = count;
this.value = value;
}
/**
* Number of other assets on which the suggestion appears.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public long getCount() {
return this.count;
}
/**
* Value of the suggestion.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public static final class SuggestedTerm {
/** Number of other assets on which the suggestion appears. */
long count;
/** Reference to the suggested term. */
GlossaryTerm value;
public SuggestedTerm(long count, String qualifiedName) {
this.count = count;
this.value = GlossaryTerm.refByQualifiedName(qualifiedName);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public long getCount() {
return this.count;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public GlossaryTerm getValue() {
return this.value;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
SuggestionResponse(final List systemDescriptions, final List userDescriptions, final List ownerUsers, final List ownerGroups, final List atlanTags, final List assignedTerms) {
this.systemDescriptions = systemDescriptions;
this.userDescriptions = userDescriptions;
this.ownerUsers = ownerUsers;
this.ownerGroups = ownerGroups;
this.atlanTags = atlanTags;
this.assignedTerms = assignedTerms;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static class SuggestionResponseBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List systemDescriptions;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List userDescriptions;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List ownerUsers;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List ownerGroups;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List atlanTags;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List assignedTerms;
@java.lang.SuppressWarnings("all")
@lombok.Generated
SuggestionResponseBuilder() {
}
/**
* Suggested system-level descriptions.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder systemDescriptions(final List systemDescriptions) {
this.systemDescriptions = systemDescriptions;
return this;
}
/**
* Suggested user-provided descriptions.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder userDescriptions(final List userDescriptions) {
this.userDescriptions = userDescriptions;
return this;
}
/**
* Suggested individual user owners.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder ownerUsers(final List ownerUsers) {
this.ownerUsers = ownerUsers;
return this;
}
/**
* Suggested group owners.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder ownerGroups(final List ownerGroups) {
this.ownerGroups = ownerGroups;
return this;
}
/**
* Suggested tags.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder atlanTags(final List atlanTags) {
this.atlanTags = atlanTags;
return this;
}
/**
* Suggested terms.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse.SuggestionResponseBuilder assignedTerms(final List assignedTerms) {
this.assignedTerms = assignedTerms;
return this;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SuggestionResponse build() {
return new SuggestionResponse(this.systemDescriptions, this.userDescriptions, this.ownerUsers, this.ownerGroups, this.atlanTags, this.assignedTerms);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "SuggestionResponse.SuggestionResponseBuilder(systemDescriptions=" + this.systemDescriptions + ", userDescriptions=" + this.userDescriptions + ", ownerUsers=" + this.ownerUsers + ", ownerGroups=" + this.ownerGroups + ", atlanTags=" + this.atlanTags + ", assignedTerms=" + this.assignedTerms + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static SuggestionResponse.SuggestionResponseBuilder builder() {
return new SuggestionResponse.SuggestionResponseBuilder();
}
/**
* Suggested system-level descriptions.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getSystemDescriptions() {
return this.systemDescriptions;
}
/**
* Suggested user-provided descriptions.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getUserDescriptions() {
return this.userDescriptions;
}
/**
* Suggested individual user owners.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getOwnerUsers() {
return this.ownerUsers;
}
/**
* Suggested group owners.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getOwnerGroups() {
return this.ownerGroups;
}
/**
* Suggested tags.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAtlanTags() {
return this.atlanTags;
}
/**
* Suggested terms.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAssignedTerms() {
return this.assignedTerms;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SuggestionResponse)) return false;
final SuggestionResponse other = (SuggestionResponse) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$systemDescriptions = this.getSystemDescriptions();
final java.lang.Object other$systemDescriptions = other.getSystemDescriptions();
if (this$systemDescriptions == null ? other$systemDescriptions != null : !this$systemDescriptions.equals(other$systemDescriptions)) return false;
final java.lang.Object this$userDescriptions = this.getUserDescriptions();
final java.lang.Object other$userDescriptions = other.getUserDescriptions();
if (this$userDescriptions == null ? other$userDescriptions != null : !this$userDescriptions.equals(other$userDescriptions)) return false;
final java.lang.Object this$ownerUsers = this.getOwnerUsers();
final java.lang.Object other$ownerUsers = other.getOwnerUsers();
if (this$ownerUsers == null ? other$ownerUsers != null : !this$ownerUsers.equals(other$ownerUsers)) return false;
final java.lang.Object this$ownerGroups = this.getOwnerGroups();
final java.lang.Object other$ownerGroups = other.getOwnerGroups();
if (this$ownerGroups == null ? other$ownerGroups != null : !this$ownerGroups.equals(other$ownerGroups)) return false;
final java.lang.Object this$atlanTags = this.getAtlanTags();
final java.lang.Object other$atlanTags = other.getAtlanTags();
if (this$atlanTags == null ? other$atlanTags != null : !this$atlanTags.equals(other$atlanTags)) return false;
final java.lang.Object this$assignedTerms = this.getAssignedTerms();
final java.lang.Object other$assignedTerms = other.getAssignedTerms();
if (this$assignedTerms == null ? other$assignedTerms != null : !this$assignedTerms.equals(other$assignedTerms)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SuggestionResponse;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $systemDescriptions = this.getSystemDescriptions();
result = result * PRIME + ($systemDescriptions == null ? 43 : $systemDescriptions.hashCode());
final java.lang.Object $userDescriptions = this.getUserDescriptions();
result = result * PRIME + ($userDescriptions == null ? 43 : $userDescriptions.hashCode());
final java.lang.Object $ownerUsers = this.getOwnerUsers();
result = result * PRIME + ($ownerUsers == null ? 43 : $ownerUsers.hashCode());
final java.lang.Object $ownerGroups = this.getOwnerGroups();
result = result * PRIME + ($ownerGroups == null ? 43 : $ownerGroups.hashCode());
final java.lang.Object $atlanTags = this.getAtlanTags();
result = result * PRIME + ($atlanTags == null ? 43 : $atlanTags.hashCode());
final java.lang.Object $assignedTerms = this.getAssignedTerms();
result = result * PRIME + ($assignedTerms == null ? 43 : $assignedTerms.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "SuggestionResponse(super=" + super.toString() + ", systemDescriptions=" + this.getSystemDescriptions() + ", userDescriptions=" + this.getUserDescriptions() + ", ownerUsers=" + this.getOwnerUsers() + ", ownerGroups=" + this.getOwnerGroups() + ", atlanTags=" + this.getAtlanTags() + ", assignedTerms=" + this.getAssignedTerms() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy