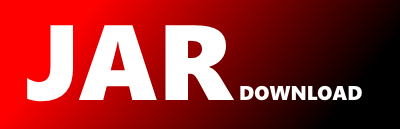
com.atlan.pkg.serde.cell.ConnectionXformer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of package-toolkit-runtime Show documentation
Show all versions of package-toolkit-runtime Show documentation
Atlan custom package runtime toolkit
/* SPDX-License-Identifier: Apache-2.0
Copyright 2023 Atlan Pte. Ltd. */
package com.atlan.pkg.serde.cell
import com.atlan.model.assets.Asset
import com.atlan.model.assets.Connection
import com.atlan.pkg.PackageContext
/**
* Static object to transform connection references.
*/
object ConnectionXformer {
const val CONNECTION_DELIMITER = "@@@"
/**
* Encodes (serializes) a connection reference into a string form.
*
* @param ctx context in which the package is running
* @param asset to be encoded
* @return the string-encoded form for that asset
*/
fun encode(
ctx: PackageContext<*>,
asset: Asset,
): String {
return when (asset) {
is Connection -> {
val connection = ctx.connectionCache.getByGuid(asset.guid)
if (connection is Connection) {
return encode(connection.name, connection.connectorType.value)
} else {
""
}
}
else -> AssetRefXformer.encode(ctx, asset)
}
}
/**
* Encodes (serializes) a connection reference into a string form.
*
* @param name of the connection
* @param type of the connector for the connection (string value)
* @return the string-encoded form for that asset
*/
fun encode(
name: String,
type: String,
): String {
return "$name$CONNECTION_DELIMITER$type"
}
/**
* Decodes (deserializes) a string form into a connection reference object.
*
* @param ctx context in which the package is running
* @param assetRef the string form to be decoded
* @param fieldName the name of the field containing the string-encoded value
* @return the connection reference represented by the string
*/
fun decode(
ctx: PackageContext<*>,
assetRef: String,
fieldName: String,
): Asset {
return when (fieldName) {
"connection" -> {
ctx.connectionCache.getByIdentity(assetRef)
?: throw NoSuchElementException("Connection $assetRef not found (in $fieldName).")
}
else -> AssetRefXformer.decode(ctx, assetRef, fieldName)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy