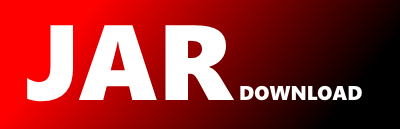
com.atlassian.asap.core.server.SimpleServerRunner Maven / Gradle / Ivy
package com.atlassian.asap.core.server;
import java.util.Set;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static org.apache.commons.lang3.StringUtils.defaultIfBlank;
/**
* A command line interface for {@link SimpleServer}.
*/
public class SimpleServerRunner
{
/**
* Port to listen on.
*/
public static final String PORT_SYSPROP = "asap.port";
/**
* System property that specifies the base URL of the public key server repository to use.
*/
public static final String PUBLIC_KEY_SERVER_URL_SYSPROP = "asap.public.key.repo.url";
/**
* System property that specifies the resource server audience.
*/
public static final String RESOURCE_SERVER_AUDIENCE_SYSPROP = "asap.resource.server.audience";
/**
* System property that specifies the authorized subjects for the resource.
* The authorized subjects must be a comma separated string of subject names without any whitespaces
*/
public static final String AUTHORIZED_SUBJECT_SYSPROP = "asap.resource.server.authorized.subjects";
/**
* System property that specifies the authorized issuers for the resource.
* The authorized issuers must be a comma separated string of issuer names without any whitespaces.
* If omitted or empty, the authorized issuers are equal to the authorized subjects.
*/
public static final String AUTHORIZED_ISSUER_SYSPROP = "asap.resource.server.authorized.issuer";
private static String DEFAULT_PUBLIC_KEY_REPO = "classpath://publickeyrepo/";
private static final Logger logger = LoggerFactory.getLogger(SimpleServerRunner.class);
/**
* Main function to run the server.
* @param args command line arguments
* @throws Exception if the server fails to start
*/
public static void main(String[] args) throws Exception
{
int port = Integer.getInteger(PORT_SYSPROP, 8080);
String publicKeyBaseUrl = defaultIfBlank(System.getProperty(PUBLIC_KEY_SERVER_URL_SYSPROP), DEFAULT_PUBLIC_KEY_REPO);
String audience = defaultIfBlank(System.getProperty(RESOURCE_SERVER_AUDIENCE_SYSPROP), "test-resource-server");
String authorizedSubjectsString = defaultIfBlank(System.getProperty(AUTHORIZED_SUBJECT_SYSPROP), "issuer1,issuer2,issuer3");
String authorizedIssuersString = defaultIfBlank(System.getProperty(AUTHORIZED_ISSUER_SYSPROP), authorizedSubjectsString);
Set authorizedSubjects = Pattern.compile(",").splitAsStream(authorizedSubjectsString).filter(StringUtils::isNotEmpty).collect(Collectors.toSet());
Set authorizedIssuers = Pattern.compile(",").splitAsStream(authorizedIssuersString).filter(StringUtils::isNotEmpty).collect(Collectors.toSet());
SimpleServer simpleServer = new SimpleServer(port, publicKeyBaseUrl, audience, authorizedSubjects, authorizedIssuers);
logger.info("Server initialized with: Audience: {}, pkPath: {}, authorized subjects: {}, authorized issuers: {}",
audience, publicKeyBaseUrl, authorizedSubjects, authorizedIssuers);
simpleServer.start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy