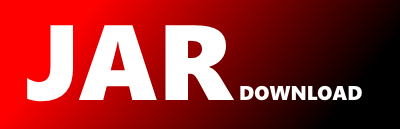
com.atlassian.asap.core.server.AsapServerConfiguration Maven / Gradle / Ivy
package com.atlassian.asap.core.server;
import java.security.PublicKey;
import java.time.Clock;
import com.atlassian.asap.api.server.http.RequestAuthenticator;
import com.atlassian.asap.core.keys.KeyProvider;
import com.atlassian.asap.core.keys.PemReader;
import com.atlassian.asap.core.keys.publickey.HttpPublicKeyProvider;
import com.atlassian.asap.core.keys.publickey.PublicKeyProviderFactory;
import com.atlassian.asap.core.parser.JwtParser;
import com.atlassian.asap.core.server.http.RequestAuthenticatorImpl;
import com.atlassian.asap.core.validator.JwtClaimsValidator;
import com.atlassian.asap.core.validator.JwtValidator;
import com.atlassian.asap.core.validator.JwtValidatorImpl;
import com.atlassian.asap.nimbus.parser.NimbusJwtParser;
import org.apache.http.client.HttpClient;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Lazy;
/**
* Server-side ASAP configuration.
*/
@Configuration
public class AsapServerConfiguration
{
@Value("${asap.audience}")
private String audience;
/**
* Definition of the {@link JwtValidator} bean.
*
* @param publicKeyProvider the public key provider to look up public keys
* @param jwtParser the parser to use for JWT token parsing
* @param jwtClaimsValidator the validator for the claims in the JWT token
* @return a token validator for the given audience and public key repository
*/
@Bean
public JwtValidator jwtValidator(KeyProvider publicKeyProvider,
JwtParser jwtParser,
JwtClaimsValidator jwtClaimsValidator)
{
return new JwtValidatorImpl(publicKeyProvider, jwtParser, jwtClaimsValidator, audience);
}
/**
* Definition of the {@link JwtClaimsValidator} bean.
*
* @return a validator of JWT claims
*/
@Bean
public JwtClaimsValidator jwtClaimsValidator()
{
return new JwtClaimsValidator(Clock.systemUTC());
}
/**
* Definition of the {@link JwtParser} bean.
*
* @return a parser of JWT tokens
*/
@Bean
public JwtParser jwtParser()
{
return new NimbusJwtParser();
}
/**
* Definition of the {@link KeyProvider} bean.
*
* @param publicKeyRepoBaseUrl base URL of the public key repository. It may be a composite URL.
* @return a public key provider
*/
@Bean
public KeyProvider publicKeyProvider(@Value("${asap.public_key_repository.url}") String publicKeyRepoBaseUrl)
{
return new PublicKeyProviderFactory(asapHttpClient(), new PemReader())
.createPublicKeyProvider(publicKeyRepoBaseUrl);
}
/**
* Definition of the {@link HttpClient} bean.
*
* @return a HTTP client
*/
@Bean
@Lazy
@Qualifier("asap")
public HttpClient asapHttpClient()
{
return HttpPublicKeyProvider.defaultHttpClient();
}
/**
* Definition of the {@link RequestAuthenticator} bean.
*
* @param jwtValidator the validator of tokens
* @return a request authenticator that authenticates valid tokens
*/
@Bean
public RequestAuthenticator requestAuthenticator(JwtValidator jwtValidator)
{
return new RequestAuthenticatorImpl(jwtValidator);
}
/**
* @return the audience that the server accepts requests for
*/
public String getAudience()
{
return audience;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy