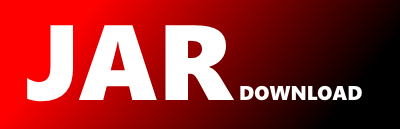
com.atlassian.bamboo.specs.api.builders.credentials.SharedCredentialsIdentifier Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.credentials;
import com.atlassian.bamboo.specs.api.builders.BambooOid;
import com.atlassian.bamboo.specs.api.builders.EntityPropertiesBuilder;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.credentials.SharedCredentialsIdentifierProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import org.jetbrains.annotations.NotNull;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotBlank;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotNull;
/**
* References a shared credential.
*/
public class SharedCredentialsIdentifier extends EntityPropertiesBuilder {
private String name;
private BambooOidProperties oid;
private SharedCredentialsScope scope = SharedCredentialsScope.GLOBAL;
private SharedCredentialsIdentifier() throws PropertiesValidationException {
}
/**
* Reference shared credential by name.
* Name of the credential is ignored if oid is defined.
*/
public SharedCredentialsIdentifier(@NotNull final String name) throws PropertiesValidationException {
checkNotBlank("name", name);
this.name = name;
}
/**
* Reference shared credential by oid.
*/
public SharedCredentialsIdentifier(@NotNull final BambooOid oid) throws PropertiesValidationException {
checkNotNull("oid", oid);
this.oid = EntityPropertiesBuilders.build(oid);
}
/**
* Reference shared credential by name.
* Name of the credential is ignored if oid is defined.
*/
public SharedCredentialsIdentifier name(@NotNull String name) throws PropertiesValidationException {
checkNotBlank("name", name);
this.name = name;
return this;
}
/**
* Reference shared credential by oid.
*/
public SharedCredentialsIdentifier oid(@NotNull final String oid) throws PropertiesValidationException {
checkNotNull("oid", oid);
return oid(new BambooOid(oid));
}
/**
* Reference shared credential by oid. Scope of shared credentials must matches, i.e. if shared credentials
* belongs to project then the scope {@link SharedCredentialsScope#PROJECT} must be set.
*/
public SharedCredentialsIdentifier oid(@NotNull final BambooOid oid) throws PropertiesValidationException {
checkNotNull("oid", oid);
this.oid = EntityPropertiesBuilders.build(oid);
return this;
}
/**
* Set scope for shared credentials. Default is {@link SharedCredentialsScope#GLOBAL}.
*/
public SharedCredentialsIdentifier scope(@NotNull final SharedCredentialsScope scope) throws PropertiesValidationException {
checkNotNull("scope", scope);
this.scope = scope;
return this;
}
protected SharedCredentialsIdentifierProperties build() throws PropertiesValidationException {
return new SharedCredentialsIdentifierProperties(name, oid, scope);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy