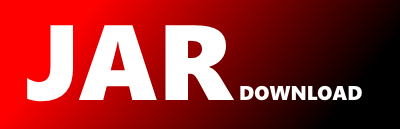
com.atlassian.bamboo.specs.api.builders.deployment.Environment Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.deployment;
import com.atlassian.bamboo.specs.api.builders.Applicability;
import com.atlassian.bamboo.specs.api.builders.EntityPropertiesBuilder;
import com.atlassian.bamboo.specs.api.builders.Variable;
import com.atlassian.bamboo.specs.api.builders.deployment.configuration.EnvironmentPluginConfiguration;
import com.atlassian.bamboo.specs.api.builders.docker.DockerConfiguration;
import com.atlassian.bamboo.specs.api.builders.notification.Notification;
import com.atlassian.bamboo.specs.api.builders.requirement.Requirement;
import com.atlassian.bamboo.specs.api.builders.task.Task;
import com.atlassian.bamboo.specs.api.builders.trigger.Trigger;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.VariableProperties;
import com.atlassian.bamboo.specs.api.model.deployment.EnvironmentProperties;
import com.atlassian.bamboo.specs.api.model.deployment.configuration.EnvironmentPluginConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.docker.DockerConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.notification.NotificationProperties;
import com.atlassian.bamboo.specs.api.model.notification.NotificationRecipientProperties;
import com.atlassian.bamboo.specs.api.model.plan.requirement.RequirementProperties;
import com.atlassian.bamboo.specs.api.model.task.TaskProperties;
import com.atlassian.bamboo.specs.api.model.trigger.TriggerProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import com.atlassian.bamboo.specs.api.validators.common.ImporterUtils;
import com.atlassian.bamboo.specs.api.validators.common.ValidationProblem;
import org.jetbrains.annotations.NotNull;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotNull;
/**
* Represents a deployment environment.
*/
public class Environment extends EntityPropertiesBuilder {
/**
* Specifies whether the release must be approved before deploying to the environment and if broken releases are allowed.
*
* @since 9.4
*/
public enum ReleaseApprovalPrerequisite {
/**
* There are no requirements. Any release can be deployed to the environment.
*/
NONE,
/**
* The release can be deployed to the environment if it's not marked as broken by anyone. This is the default prerequisite.
*/
NOT_BROKEN,
/**
* The release can be deployed to the environment if there is at least one approval and the release is not marked as broken by anyone.
*/
APPROVED;
public static ReleaseApprovalPrerequisite getDefault() {
return NOT_BROKEN;
}
}
private String name;
private String description;
private List tasks = new ArrayList<>();
private List finalTasks = new ArrayList<>();
private List triggers = new ArrayList<>();
private List variables = new ArrayList<>();
private List requirements = new ArrayList<>();
private List notifications = new ArrayList<>();
private DockerConfigurationProperties dockerConfiguration;
private Map pluginConfigurations = new LinkedHashMap<>();
// environment prerequisites:
private ReleaseApprovalPrerequisite releaseApprovalPrerequisite = ReleaseApprovalPrerequisite.getDefault();
/**
* Specifies environment.
*
* @param name name of the environment.
*/
public Environment(@NotNull String name) {
ImporterUtils.checkNotNull("name", name);
this.name = name;
this.dockerConfiguration(new DockerConfiguration().enabled(false));
}
/**
* Sets the environment description.
*/
public Environment description(String description) {
this.description = description;
return this;
}
/**
* Sets release approval prerequisite the environment requires to be met by deployment project release
* before the deployment can be launched. The deployment project release is the context the environment is executed in.
* The default prerequisite is {@link ReleaseApprovalPrerequisite#NOT_BROKEN}.
*
* @param releaseApprovalPrerequisite the prerequisite to set
* @since 9.4
*/
public Environment releaseApprovalPrerequisite(@NotNull ReleaseApprovalPrerequisite releaseApprovalPrerequisite) {
this.releaseApprovalPrerequisite = releaseApprovalPrerequisite;
return this;
}
/**
* Sets release approval prerequisite the environment requires to be met by deployment project release
* to {@link Environment.ReleaseApprovalPrerequisite#NONE}.
* That means there are no requirements. Any release can be deployed to the environment.
*
* @since 10.0
*/
public Environment noneReleaseApprovalPrerequisite() {
return releaseApprovalPrerequisite(ReleaseApprovalPrerequisite.NONE);
}
/**
* Sets release approval prerequisite the environment requires to be met by deployment project release
* to {@link Environment.ReleaseApprovalPrerequisite#NOT_BROKEN}.
* That means the release can be deployed to the environment if it's not marked as broken by anyone.
* This is the default prerequisite.
*
* @since 10.0
*/
public Environment notBrokenReleaseApprovalPrerequisite() {
return releaseApprovalPrerequisite(ReleaseApprovalPrerequisite.NOT_BROKEN);
}
/**
* Sets release approval prerequisite the environment requires to be met by deployment project release
* to {@link Environment.ReleaseApprovalPrerequisite#APPROVED}.
* That means the release can be deployed to the environment if there is at least one approval and the release
* is not marked as broken by anyone.
*
* @since 10.0
*/
public Environment approvedReleaseApprovalPrerequisite() {
return releaseApprovalPrerequisite(ReleaseApprovalPrerequisite.APPROVED);
}
/**
* Adds provided {@link Task}s to the list of tasks executed by the environment.
*/
public Environment tasks(@NotNull Task, ?>... tasks) {
checkNotNull("tasks", tasks);
for (Task, ?> t : tasks) {
TaskProperties task = EntityPropertiesBuilders.build(t);
if (!task.applicableTo().contains(Applicability.DEPLOYMENTS)) {
throw new PropertiesValidationException("Task " + t.getClass().getSimpleName() + " is not available in deployments");
} else {
addTask(this.tasks, task);
}
}
return this;
}
/**
* Adds provided {@link Task}s to the list of the final tasks executed by the environment.
* Final tasks for a environment are always executed, even if previous tasks in the environment failed.
*/
public Environment finalTasks(@NotNull Task, ?>... finalTasks) {
checkNotNull("finalTasks", finalTasks);
for (Task, ?> t : finalTasks) {
TaskProperties task = EntityPropertiesBuilders.build(t);
if (!task.applicableTo().contains(Applicability.DEPLOYMENTS)) {
throw new PropertiesValidationException("Task " + t.getClass().getSimpleName() + " is not available in deployments");
} else {
addTask(this.finalTasks, task);
}
}
return this;
}
private void addTask(final List tasks, final TaskProperties task) {
requirements.addAll(task.getRequirements());
tasks.add(task);
}
/**
* Adds triggers to this environment.
*/
public Environment triggers(@NotNull Trigger, ?>... triggers) {
checkNotNull("triggers", triggers);
for (Trigger, ?> t : triggers) {
TriggerProperties trigger = EntityPropertiesBuilders.build(t);
if (!trigger.applicableTo().contains(Applicability.DEPLOYMENTS)) {
throw new PropertiesValidationException("Trigger " + t.getClass().getSimpleName() + " is not available in deployments");
} else {
this.triggers.add(trigger);
}
}
return this;
}
/**
* Adds deployment environment variables.
*/
public Environment variables(@NotNull Variable... variables) {
checkNotNull("variables", variables);
Arrays.stream(variables)
.map(EntityPropertiesBuilders::build)
.forEach(this.variables::add);
return this;
}
/**
* Adds custom requirements to the environment.
*
* Requirements control which Bamboo agents are able to execute deployments to the environment.
*/
public Environment requirements(Requirement... requirements) {
checkNotNull("requirements", requirements);
Arrays.stream(requirements)
.map(EntityPropertiesBuilders::build)
.forEach(this.requirements::add);
return this;
}
/**
* Appends a notification rule to the environment.
*/
public Environment notifications(@NotNull final Notification... notifications) {
ImporterUtils.checkNotNull("notifications", notifications);
Arrays.stream(notifications)
.map(EntityPropertiesBuilders::build)
.forEach(notification -> {
List problems = new ArrayList<>();
if (!notification.getType().applicableTo().contains(Applicability.DEPLOYMENTS)) {
problems.add(new ValidationProblem(String.format("Can't add notification because notification type (%s) is not available in deployments",
notification.getType().getAtlassianPlugin().getCompleteModuleKey())));
}
for (NotificationRecipientProperties recipient : notification.getRecipients()) {
if (!recipient.applicableTo().contains(Applicability.DEPLOYMENTS)) {
problems.add(new ValidationProblem(String.format("Can't add notification because notification recipient (%s) is not available in deployments",
recipient.getAtlassianPlugin().getCompleteModuleKey())));
}
}
if (!problems.isEmpty()) {
throw new PropertiesValidationException(problems);
} else {
this.notifications.add(notification);
}
});
return this;
}
/**
* Configure Docker for this environment.
*
* @see DockerConfiguration
*/
public Environment dockerConfiguration(@NotNull DockerConfiguration dockerConfiguration) {
ImporterUtils.checkNotNull("dockerConfiguration", dockerConfiguration);
this.dockerConfiguration = EntityPropertiesBuilders.build(dockerConfiguration);
return this;
}
/**
* Appends plugin configuration to the environment. If the same plugin is specified second time, its configuration
* is overwritten.
*/
public Environment pluginConfigurations(@NotNull final EnvironmentPluginConfiguration extends EnvironmentPluginConfigurationProperties>... pluginConfigurations) {
ImporterUtils.checkNotNull("pluginConfigurations", pluginConfigurations);
for (final EnvironmentPluginConfiguration extends EnvironmentPluginConfigurationProperties> pluginConfiguration : pluginConfigurations) {
if (pluginConfiguration != null) {
putPluginConfiguration(pluginConfiguration);
}
}
return this;
}
private void putPluginConfiguration(final EnvironmentPluginConfiguration> pluginConfiguration) {
EnvironmentPluginConfigurationProperties pluginConfigurationProperties = EntityPropertiesBuilders.build(pluginConfiguration);
pluginConfigurations.put(pluginConfigurationProperties.getAtlassianPlugin().getCompleteModuleKey(), pluginConfigurationProperties);
}
public String getName() {
return name;
}
@Override
protected EnvironmentProperties build() {
return new EnvironmentProperties(
name,
description,
tasks,
finalTasks,
triggers,
variables,
requirements,
notifications,
dockerConfiguration,
pluginConfigurations.values(),
releaseApprovalPrerequisite);
}
}