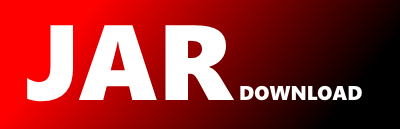
com.atlassian.bamboo.specs.api.builders.plan.Job Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.plan;
import com.atlassian.bamboo.specs.api.builders.Applicability;
import com.atlassian.bamboo.specs.api.builders.BambooKey;
import com.atlassian.bamboo.specs.api.builders.EntityPropertiesBuilder;
import com.atlassian.bamboo.specs.api.builders.docker.DockerConfiguration;
import com.atlassian.bamboo.specs.api.builders.plan.artifact.Artifact;
import com.atlassian.bamboo.specs.api.builders.plan.artifact.ArtifactSubscription;
import com.atlassian.bamboo.specs.api.builders.plan.configuration.AllOtherPluginsConfiguration;
import com.atlassian.bamboo.specs.api.builders.plan.configuration.PluginConfiguration;
import com.atlassian.bamboo.specs.api.builders.requirement.Requirement;
import com.atlassian.bamboo.specs.api.builders.task.Task;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooKeyProperties;
import com.atlassian.bamboo.specs.api.model.docker.DockerConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.plan.JobProperties;
import com.atlassian.bamboo.specs.api.model.plan.artifact.ArtifactProperties;
import com.atlassian.bamboo.specs.api.model.plan.artifact.ArtifactSubscriptionProperties;
import com.atlassian.bamboo.specs.api.model.plan.configuration.PluginConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.plan.requirement.RequirementProperties;
import com.atlassian.bamboo.specs.api.model.task.TaskProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import com.atlassian.bamboo.specs.api.util.PluginConfigurationHelper;
import com.atlassian.bamboo.specs.api.validators.common.ImporterUtils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotNull;
/**
* Represents a Bamboo job. Job is a unit of work within {@link Stage} that can be run parallel to other jobs if multiple
* agents are available. When executing several jobs on single agents, order in which jobs from single stage are executed is not defined.
*/
public class Job extends EntityPropertiesBuilder {
private BambooKeyProperties key;
private String name;
private String description = "";
private boolean enabled = true;
private boolean cleanWorkingDirectory = false;
private final List artifacts = new ArrayList<>();
private final List tasks = new ArrayList<>();
private final List finalTasks = new ArrayList<>();
private final List requirements = new ArrayList<>();
private final List subscriptions = new ArrayList<>();
private final Map pluginConfigurations = new LinkedHashMap<>();
private DockerConfigurationProperties dockerConfiguration;
public Job(@NotNull final Job copy) {
this.key = copy.key;
this.name = copy.name;
this.description = copy.description;
this.enabled = copy.enabled;
this.cleanWorkingDirectory = copy.cleanWorkingDirectory;
this.artifacts.addAll(copy.artifacts);
this.tasks.addAll(copy.tasks);
this.finalTasks.addAll(copy.finalTasks);
this.requirements.addAll(copy.requirements);
this.subscriptions.addAll(copy.subscriptions);
this.pluginConfigurations.putAll(copy.pluginConfigurations);
this.dockerConfiguration = copy.dockerConfiguration;
}
/**
* Specify job with given name and key.
*
* If oid is not specified, key serves as a job identifier.
* If a job with specified key does not exist, a new one is created. If it does exists it is updated, it can also be moved between stages.
*
* @param name job's name
* @param key job's short key, must be unique within the plan.
*/
public Job(@NotNull String name, @NotNull String key) throws PropertiesValidationException {
checkNotNull("name", name);
checkNotNull("key", key);
this.name(name);
this.key(key);
this.dockerConfiguration(new DockerConfiguration().enabled(false));
}
/**
* Specify job with given name and key.
*
* If oid is not specified, key serves as a job identifier.
* If a job with specified key does not exist, a new one is creted. If it does exists it is updated, it can also be moved between stages.
*
* @param name job's name
* @param key job's short key, must be unique within the plan.
*/
public Job(@NotNull String name, @NotNull BambooKey key) throws PropertiesValidationException {
checkNotNull("name", name);
checkNotNull("key", key);
this.name(name);
this.key(key);
this.dockerConfiguration(new DockerConfiguration().enabled(false));
}
/**
* Sets the job's name.
*/
public Job name(@NotNull String name) throws PropertiesValidationException {
checkNotNull("name", name);
this.name = name;
return this;
}
/**
* Sets the job's key.
*
* If oid is not specified, key serves as a job identifier.
* If a job with specified key does not exist, a new one is created. If it does exists it is updated, it can also be moved between stages.
*
* @param key job's short key, must be unique within the plan.
*/
public Job key(@NotNull String key) throws PropertiesValidationException {
checkNotNull("key", key);
return key(new BambooKey(key));
}
/**
* Sets the job's key.
*
* If oid is not specified, key serves as a job identifier.
* If a job with specified key does not exist, a new one is created. If it does exists it is updated, in particular
* it can be moved between stages.
*
* @param key job's short key, must be unique within the plan.
*/
public Job key(@NotNull BambooKey key) throws PropertiesValidationException {
checkNotNull("key", key);
this.key = EntityPropertiesBuilders.build(key);
return this;
}
/**
* Sets the job's description.
*/
public Job description(@Nullable String description) throws PropertiesValidationException {
this.description = description;
return this;
}
/**
* Enables/disables the job.
*/
public Job enabled(boolean enabled) throws PropertiesValidationException {
this.enabled = enabled;
return this;
}
/**
* Adds provided {@link Artifact}s to the list of artifacts produced by the job.
*/
public Job artifacts(@NotNull Artifact... artifacts) {
checkNotNull("artifacts", artifacts);
Arrays.stream(artifacts)
.map(EntityPropertiesBuilders::build)
.forEach(this.artifacts::add);
return this;
}
/**
* Add artifact subscriptions. Subscription can only be defined for shared artifacts.
*
* Artifact subscription specify which subsequent jobs rely on this artifact.
*/
public Job artifactSubscriptions(@NotNull ArtifactSubscription... subscriptions) {
checkNotNull("subscriptions", subscriptions);
Arrays.stream(subscriptions)
.map(EntityPropertiesBuilders::build)
.forEach(this.subscriptions::add);
return this;
}
/**
* Adds provided {@link Task}s to the list of tasks executed by the job.
*/
public Job tasks(@NotNull Task, ?>... tasks) {
checkNotNull("tasks", tasks);
for (Task, ?> t : tasks) {
TaskProperties task = EntityPropertiesBuilders.build(t);
if (!task.applicableTo().contains(Applicability.PLANS)) {
throw new PropertiesValidationException("Task " + t.getClass().getSimpleName() + " is not available in plans");
} else {
addTask(this.tasks, task);
}
}
return this;
}
/**
* Adds provided {@link Task}s to the list of the final tasks executed by the job.
*
Final tasks for a job are always executed, even if previous tasks in the job failed.
*/
public Job finalTasks(@NotNull Task, ?>... finalTasks) {
checkNotNull("finalTasks", finalTasks);
for (final Task, ?> t : finalTasks) {
TaskProperties task = EntityPropertiesBuilders.build(t);
if (!task.applicableTo().contains(Applicability.PLANS)) {
throw new PropertiesValidationException("Task " + t.getClass().getSimpleName() + " is not available in plans");
} else {
addTask(this.finalTasks, task);
}
}
return this;
}
private void addTask(final List tasks, final TaskProperties task) {
requirements.addAll(task.getRequirements());
tasks.add(task);
}
/**
* Adds custom requirements to the job.
*
* Requirements control which Bamboo agents are able to execute the job.
*/
public Job requirements(Requirement... requirements) {
checkNotNull("requirements", requirements);
Arrays.stream(requirements)
.map(EntityPropertiesBuilders::build)
.forEach(this.requirements::add);
return this;
}
/**
* Specifies if job should clean working directory after executing.
*/
public Job cleanWorkingDirectory(boolean cleanWorkingDirectory) {
this.cleanWorkingDirectory = cleanWorkingDirectory;
return this;
}
/**
* Appends plugin configuration to the plan. If the same plugin is specified second time, its configuration
* is overwritten.
*/
public Job pluginConfigurations(@NotNull final PluginConfiguration>... pluginConfigurations) {
ImporterUtils.checkNotNull("pluginConfigurations", pluginConfigurations);
for (PluginConfiguration> pluginConfiguration : pluginConfigurations) {
if (pluginConfiguration != null) {
PluginConfigurationHelper.putPluginConfiguration(this.pluginConfigurations, pluginConfiguration);
}
}
return this;
}
/**
* Use this method to specify that your job has default plugins configuration.
*/
@Deprecated
public Job noPluginConfigurations() {
return pluginConfigurations(new AllOtherPluginsConfiguration());
}
/**
* Configure Docker for this job.
*
* @see DockerConfiguration
*/
public Job dockerConfiguration(@NotNull DockerConfiguration dockerConfiguration) {
ImporterUtils.checkNotNull("dockerConfiguration", dockerConfiguration);
this.dockerConfiguration = EntityPropertiesBuilders.build(dockerConfiguration);
return this;
}
/**
* Returns job's key, which serves as identifier for this object.
*
* @throws IllegalStateException if key is undefined
*/
@NotNull
public BambooKey getKey() {
if (key == null) {
throw new IllegalStateException("Job key is undefined");
}
return new BambooKey(key.getKey());
}
protected JobProperties build() throws PropertiesValidationException {
return new JobProperties(
key,
name,
description,
enabled,
cleanWorkingDirectory,
pluginConfigurations.values(),
artifacts,
tasks,
finalTasks,
requirements,
subscriptions,
dockerConfiguration);
}
}