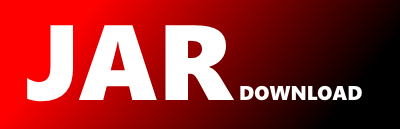
com.atlassian.bamboo.specs.api.builders.plan.branches.BranchIntegration Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.plan.branches;
import com.atlassian.bamboo.specs.api.builders.BambooKey;
import com.atlassian.bamboo.specs.api.builders.BambooOid;
import com.atlassian.bamboo.specs.api.builders.EntityPropertiesBuilder;
import com.atlassian.bamboo.specs.api.builders.plan.PlanBranchIdentifier;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.plan.branches.BranchIntegrationProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import org.jetbrains.annotations.NotNull;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotNull;
/**
* Represents branch merging strategy.
*/
public class BranchIntegration extends EntityPropertiesBuilder {
private boolean enabled = true;
private PlanBranchIdentifier integrationBranch;
private boolean gatekeeper;
private boolean pushOn;
/**
* Enables/disables automatic branch merging. Enabled by default.
*/
public BranchIntegration enabled(boolean enabled) throws PropertiesValidationException {
this.enabled = enabled;
return this;
}
/**
* Sets integration branch oid.
*
* If both oid and key is defined, key is ignored.
*/
public BranchIntegration integrationBranchOid(@NotNull String integrationBranchOid) throws PropertiesValidationException {
checkNotNull("integrationBranchOid", integrationBranchOid);
return integrationBranchOid(new BambooOid(integrationBranchOid));
}
/**
* Sets integration branch oid.
*
* If both oid and key is defined, key is ignored.
*/
public BranchIntegration integrationBranchOid(@NotNull BambooOid integrationBranchOid) throws PropertiesValidationException {
checkNotNull("integrationBranchOid", integrationBranchOid);
if (this.integrationBranch != null) {
this.integrationBranch.oid(integrationBranchOid);
} else {
this.integrationBranch = new PlanBranchIdentifier(integrationBranchOid);
}
return this;
}
/**
* Sets integration branch key.
*
* If both oid and key is defined, key is ignored.
*/
public BranchIntegration integrationBranchKey(@NotNull String integrationBranchKey) throws PropertiesValidationException {
checkNotNull("integrationBranchKey", integrationBranchKey);
return integrationBranchKey(new BambooKey(integrationBranchKey));
}
/**
* Sets integration branch key.
*
* If both oid and key is defined, key is ignored.
*/
public BranchIntegration integrationBranchKey(@NotNull BambooKey integrationBranchKey) throws PropertiesValidationException {
checkNotNull("integrationBranchKey", integrationBranchKey);
if (this.integrationBranch != null) {
this.integrationBranch.key(integrationBranchKey);
} else {
this.integrationBranch = new PlanBranchIdentifier(integrationBranchKey);
}
return this;
}
/**
* Sets integration branch identifier.
*
* If both oid and key is defined by the identifier, key is ignored.
*/
public BranchIntegration integrationBranch(@NotNull PlanBranchIdentifier integrationBranch) throws PropertiesValidationException {
checkNotNull("integrationBranch", integrationBranch);
this.integrationBranch = new PlanBranchIdentifier(integrationBranch);
return this;
}
/**
* Selects merging strategy. If true, the integration branch is the target branch of the merge and, possibly, the push.
* If false, the integration branch is the source branch of the merge, and current branch is the target.
*
* Default is false (current branch is the target of the merge).
*/
public BranchIntegration gatekeeper(boolean gatekeeper) throws PropertiesValidationException {
this.gatekeeper = gatekeeper;
return this;
}
/**
* Enables/disables executing push on successful build. The target branch is selected by setting {@link #gatekeeper(boolean)} option.
* Feature is turned off by default.
*/
public BranchIntegration pushOnSuccessfulBuild(boolean push) throws PropertiesValidationException {
this.pushOn = push;
return this;
}
protected BranchIntegrationProperties build() throws PropertiesValidationException {
if (enabled && integrationBranch != null) {
return new BranchIntegrationProperties(enabled, EntityPropertiesBuilders.build(integrationBranch), gatekeeper, pushOn);
} else {
return new BranchIntegrationProperties(enabled, null, gatekeeper, pushOn);
}
}
}