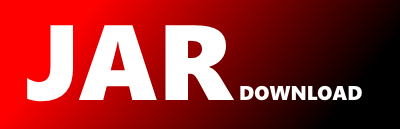
com.atlassian.bamboo.specs.api.builders.plan.configuration.ConcurrentBuilds Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.plan.configuration;
import com.atlassian.bamboo.specs.api.model.plan.configuration.ConcurrentBuildsProperties;
import com.atlassian.bamboo.specs.api.validators.common.ImporterUtils;
import com.atlassian.bamboo.specs.api.validators.common.ValidationContext;
import org.jetbrains.annotations.NotNull;
import java.util.Objects;
public class ConcurrentBuilds extends PluginConfiguration {
private boolean useSystemWideDefault = true;
private int maximumNumberOfConcurrentBuilds = 1;
private ConcurrentBuildsStrategy concurrentBuildsStrategy = ConcurrentBuildsStrategy.getDefault();
/**
* Specifies the strategy to use when the maximum number of concurrent builds is reached.
*
* @since 10.0
*/
public enum ConcurrentBuildsStrategy {
/**
* Blocks the subsequent build execution until a slot is available. This is the default strategy.
*/
BLOCK_TRIGGERING,
/**
* Always allows executing the fresh build and keeps only the latest builds by stopping the old ones that
* don't meet the concurrent builds number limit. The build priority is determined based on the build start time.
*/
STOP_OLDEST_BUILDS;
public static ConcurrentBuildsStrategy getDefault() {
return BLOCK_TRIGGERING;
}
}
/**
* Sets whether to use the system-wide default for concurrent builds.
*
* @param useSystemWideDefault if true, the system-wide default will be used. Otherwise, the maximumNumberOfConcurrentBuilds will be used.
*/
public ConcurrentBuilds useSystemWideDefault(boolean useSystemWideDefault) {
this.useSystemWideDefault = useSystemWideDefault;
return this;
}
/**
* Sets the maximum number of concurrent builds. Automatically set useSystemWideDefault to false.
*
* @param maximumNumberOfConcurrentBuilds the maximum number of concurrent builds.
*/
public ConcurrentBuilds maximumNumberOfConcurrentBuilds(int maximumNumberOfConcurrentBuilds) {
ImporterUtils.checkThat(ValidationContext.of("maximumNumberOfConcurrentBuilds"), maximumNumberOfConcurrentBuilds > 0, "Maximum number of concurrent builds must be greater than 0.");
this.maximumNumberOfConcurrentBuilds = maximumNumberOfConcurrentBuilds;
useSystemWideDefault(false);
return this;
}
/**
* Specifies the strategy to use when the maximum number of concurrent builds is reached.
*
* @param concurrentBuildsStrategy the strategy to use.
* @since 10.0
*/
public ConcurrentBuilds concurrentBuildsStrategy(ConcurrentBuildsStrategy concurrentBuildsStrategy) {
this.concurrentBuildsStrategy = concurrentBuildsStrategy;
return this;
}
/**
* Specifies the {@link ConcurrentBuilds.ConcurrentBuildsStrategy#BLOCK_TRIGGERING} strategy to be used when the
* maximum number of concurrent builds is reached.
* This strategy blocks the subsequent build execution until a slot is available. This is the default strategy.
*
* @since 10.0
*/
public ConcurrentBuilds blockTriggeringStrategy() {
return concurrentBuildsStrategy(ConcurrentBuildsStrategy.BLOCK_TRIGGERING);
}
/**
* Specifies the {@link ConcurrentBuilds.ConcurrentBuildsStrategy#STOP_OLDEST_BUILDS} strategy to be used when the
* maximum number of concurrent builds is reached.
* This strategy always allows executing the fresh build and keeps only the latest builds by stopping the old ones that
* don't meet the concurrent builds number limit. The build priority is determined based on the build start time.
*
* @since 10.0
*/
public ConcurrentBuilds stopOldestBuildsStrategy() {
return concurrentBuildsStrategy(ConcurrentBuildsStrategy.STOP_OLDEST_BUILDS);
}
@Override
@NotNull
protected ConcurrentBuildsProperties build() {
return new ConcurrentBuildsProperties(useSystemWideDefault, maximumNumberOfConcurrentBuilds, concurrentBuildsStrategy);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ConcurrentBuilds)) {
return false;
}
ConcurrentBuilds that = (ConcurrentBuilds) o;
return useSystemWideDefault == that.useSystemWideDefault
&& maximumNumberOfConcurrentBuilds == that.maximumNumberOfConcurrentBuilds
&& concurrentBuildsStrategy == that.concurrentBuildsStrategy;
}
@Override
public int hashCode() {
return Objects.hash(useSystemWideDefault, maximumNumberOfConcurrentBuilds, concurrentBuildsStrategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy