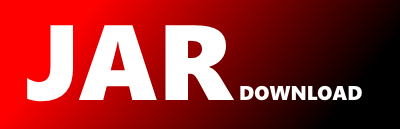
com.atlassian.bamboo.specs.api.builders.requirement.Requirement Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders.requirement;
import com.atlassian.bamboo.specs.api.builders.EntityPropertiesBuilder;
import com.atlassian.bamboo.specs.api.model.plan.requirement.RequirementProperties;
import java.util.regex.Pattern;
/**
* Represents a custom requirement.
*/
public class Requirement extends EntityPropertiesBuilder {
public enum MatchType {
EXISTS, EQUALS, MATCHES
}
private String key;
private String matchValue = ".*";
private MatchType matchType = MatchType.EXISTS;
/**
* Specifies an "exists" requirement with a specified key.
*
* @param key requirement key
*/
public Requirement(String key) {
this.key = key;
}
/**
* Specifies a requirement that a capability with matching key exists.
*
* @param key requirement key
*/
public static Requirement exists(String key) {
return new Requirement(key).matchType(MatchType.EXISTS);
}
/**
* Specifies a requirement that a capability with matching key has value equal to value of requirement.
*
* @param key requirement key
* @param value requirement value
*/
public static Requirement equals(String key, String value) {
return new Requirement(key)
.matchType(MatchType.EQUALS)
.matchValue(value);
}
/**
* Specifies a requirement that a capability with matching key has value that matches regexp provided in value of requirement.
*
* @param key requirement key
* @param regexp requirement value, in Java format
* @see Pattern
*/
public static Requirement matches(String key, String regexp) {
return new Requirement(key)
.matchType(MatchType.MATCHES)
.matchValue(regexp);
}
/**
* Sets a requirement value. Depending on the match type should be a string or a Java regular expression.
*/
public Requirement matchValue(final String value) {
this.matchValue = value;
return this;
}
/**
* Sets a match type. Possible values are:
*
* - EXISTS
* - A capability with a matching key must exist, the value is not checked
* - EQUALS
* - A capability with a matching key must exist and its value must be equal to value of requirement
* - MATCHES
* - A capability with a matching key must exist and its value must match the value of requirement interpreted as Java regular expression
*
*/
public Requirement matchType(final MatchType matchType) {
this.matchType = matchType;
return this;
}
protected RequirementProperties build() {
return new RequirementProperties(key, matchValue, matchType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy