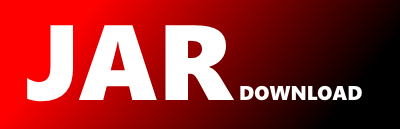
com.atlassian.bamboo.specs.api.model.docker.DockerConfigurationProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.docker;
import com.atlassian.bamboo.specs.api.builders.docker.DockerConfiguration;
import com.atlassian.bamboo.specs.api.codegen.annotations.CodeGeneratorName;
import com.atlassian.bamboo.specs.api.codegen.annotations.DefaultFieldValues;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.EntityProperties;
import com.atlassian.bamboo.specs.api.model.deployment.EnvironmentProperties;
import com.atlassian.bamboo.specs.api.model.plan.JobProperties;
import com.atlassian.bamboo.specs.api.validators.common.ImporterUtils;
import com.atlassian.bamboo.specs.api.validators.common.ValidationContext;
import org.apache.commons.lang3.StringUtils;
import javax.annotation.concurrent.Immutable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
@Immutable
public class DockerConfigurationProperties implements EntityProperties {
public static final ValidationContext VALIDATION_CONTEXT = ValidationContext.of("Docker configuration");
public static final Map DEFAULT_VOLUMES;
private final boolean enabled;
private final String image;
@CodeGeneratorName("com.atlassian.bamboo.specs.codegen.emitters.docker.DockerConfigurationVolumesEmitter")
private final Map volumes;
private final List dockerRunArguments;
static {
final Map defaultVolumes = new LinkedHashMap<>();
defaultVolumes.put("${bamboo.working.directory}", "${bamboo.working.directory}");
defaultVolumes.put("${bamboo.tmp.directory}", "${bamboo.tmp.directory}");
DEFAULT_VOLUMES = Collections.unmodifiableMap(defaultVolumes);
}
/**
* This constructor needs to create a Docker config that is equal to the default values of
* {@link JobProperties#dockerConfiguration} and {@link EnvironmentProperties#dockerConfiguration}
* for Specs export to work properly.
*
* It should also be equal to the default value that is used for jobs and environments in Bamboo (for example, for
* those created using the UI).
*/
private DockerConfigurationProperties() {
this(false, Collections.emptyMap(), Collections.emptyList());
}
private DockerConfigurationProperties(boolean enabled, Map volumes, List dockerRunArguments) {
this.enabled = enabled;
this.image = null;
this.volumes = volumes;
this.dockerRunArguments = dockerRunArguments;
}
public DockerConfigurationProperties(boolean enabled, String image, Map volumes, List dockerRunArguments) {
this.enabled = enabled;
this.image = image;
this.volumes = volumes != null ? Collections.unmodifiableMap(new LinkedHashMap<>(volumes)) : Collections.emptyMap();
this.dockerRunArguments = dockerRunArguments != null ? Collections.unmodifiableList(new ArrayList<>(dockerRunArguments)) : Collections.emptyList();
validate();
}
/**
* This factory method needs to create a Docker config which is equal to the result of building a new instance of
* {@link DockerConfiguration}, for Specs export to work properly. Such instance doesn't have to be valid.
*
* We can't simply call {@link DockerConfiguration#build()}, because such call performs validation.
*/
@DefaultFieldValues
private static DockerConfigurationProperties defaults() {
return new DockerConfigurationProperties(true, DEFAULT_VOLUMES, Collections.emptyList());
}
@Override
public void validate() {
if (enabled) {
ImporterUtils.checkNotBlank(VALIDATION_CONTEXT, "image", image);
final Set occupiedContainerVolumes = new HashSet<>();
volumes.forEach((key, value) -> {
ImporterUtils.checkNotBlank(VALIDATION_CONTEXT, "volume container directory", StringUtils.trimToNull(value));
ImporterUtils.checkNotBlank(VALIDATION_CONTEXT, "volume host directory", StringUtils.trimToNull(key));
if (!occupiedContainerVolumes.add(value)) {
throw new PropertiesValidationException(VALIDATION_CONTEXT, "Volume " + value + " is already defined.");
}
});
dockerRunArguments.forEach(arg -> {
ImporterUtils.checkNotBlank(VALIDATION_CONTEXT, "argument", arg);
});
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof DockerConfigurationProperties)) {
return false;
}
final DockerConfigurationProperties that = (DockerConfigurationProperties) o;
return enabled == that.enabled &&
Objects.equals(image, that.image) &&
Objects.equals(volumes, that.volumes) &&
Objects.equals(dockerRunArguments, that.dockerRunArguments);
}
@Override
public int hashCode() {
return Objects.hash(enabled, image, volumes, dockerRunArguments);
}
public boolean isEnabled() {
return enabled;
}
public String getImage() {
return image;
}
public Map getVolumes() {
return volumes;
}
public List getDockerRunArguments() {
return dockerRunArguments;
}
}