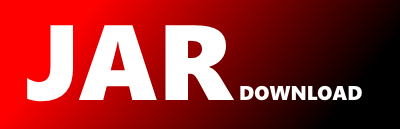
com.atlassian.bamboo.specs.api.model.permission.DeploymentPermissionsProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.permission;
import com.atlassian.bamboo.specs.api.builders.permission.DeploymentPermissions;
import com.atlassian.bamboo.specs.api.codegen.annotations.ConstructFrom;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.RootEntityProperties;
import com.atlassian.bamboo.specs.api.validators.permission.PermissionValidator;
import org.jetbrains.annotations.NotNull;
import javax.annotation.concurrent.Immutable;
import java.util.Objects;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNoErrors;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNotNull;
@Immutable
@ConstructFrom({"deploymentName"})
public class DeploymentPermissionsProperties implements RootEntityProperties {
private final BambooOidProperties deploymentOid;
private final String deploymentName;
private final PermissionsProperties permissions;
private DeploymentPermissionsProperties() {
this.deploymentOid = null;
this.deploymentName = null;
this.permissions = null;
}
public DeploymentPermissionsProperties(@NotNull final String deploymentName,
@NotNull final PermissionsProperties permissions)
throws PropertiesValidationException {
this.deploymentOid = null;
this.deploymentName = deploymentName;
this.permissions = permissions;
validate();
}
public DeploymentPermissionsProperties(@NotNull final BambooOidProperties deploymentOid,
final @NotNull PermissionsProperties permissions)
throws PropertiesValidationException {
this.deploymentOid = deploymentOid;
this.deploymentName = null;
this.permissions = permissions;
validate();
}
public void validate() throws PropertiesValidationException {
if (deploymentName == null && deploymentOid == null) {
throw new PropertiesValidationException("deployment oid or name should be not null");
}
checkNotNull("permissions", permissions);
checkNoErrors(PermissionValidator.validatePermissions(permissions, PermissionValidator.PermissionTarget.DEPLOYMENT));
}
public BambooOidProperties getDeploymentOid() {
return deploymentOid;
}
public String getDeploymentName() {
return deploymentName;
}
public PermissionsProperties getPermissions() {
return permissions;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DeploymentPermissionsProperties that = (DeploymentPermissionsProperties) o;
return Objects.equals(deploymentOid, that.deploymentOid) &&
Objects.equals(deploymentName, that.deploymentName) &&
Objects.equals(permissions, that.permissions);
}
@Override
public int hashCode() {
return Objects.hash(deploymentOid, deploymentName, permissions);
}
@NotNull
@Override
public String humanReadableType() {
return DeploymentPermissions.TYPE;
}
@Override
public String humanReadableId() {
final StringBuilder id = new StringBuilder();
id.append(DeploymentPermissions.TYPE);
id.append(" for deployment ");
if (deploymentOid != null) {
id.append(deploymentOid);
} else {
id.append(deploymentName);
}
return id.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy