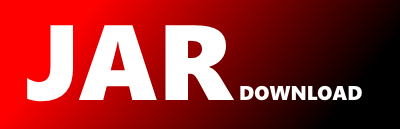
com.atlassian.bamboo.specs.api.model.plan.AbstractPlanIdentifierProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.plan;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooKeyProperties;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.EntityProperties;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.Objects;
@Immutable
public class AbstractPlanIdentifierProperties implements EntityProperties {
protected final BambooKeyProperties key;
protected final BambooOidProperties oid;
protected AbstractPlanIdentifierProperties() {
key = null;
oid = null;
}
public AbstractPlanIdentifierProperties(@Nullable final BambooKeyProperties key,
@Nullable final BambooOidProperties oid) throws PropertiesValidationException {
this.key = key;
this.oid = oid;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AbstractPlanIdentifierProperties that = (AbstractPlanIdentifierProperties) o;
return Objects.equals(getKey(), that.getKey()) &&
Objects.equals(getOid(), that.getOid());
}
@Override
public int hashCode() {
return Objects.hash(getKey(), getOid());
}
@Override
public String toString() {
return new ToStringBuilder(this, ToStringStyle.NO_CLASS_NAME_STYLE)
.append("oid", oid)
.append("key", key)
.build();
}
@Nullable
public BambooKeyProperties getKey() {
return key;
}
public boolean isKeyDefined() {
return key != null;
}
@Nullable
public BambooOidProperties getOid() {
return oid;
}
public boolean isOidDefined() {
return oid != null;
}
@Override
public void validate() {
if (key == null && oid == null) {
throw new PropertiesValidationException("Either key or oid need to be defined when referencing plan or job");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy