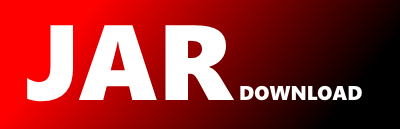
com.atlassian.bamboo.specs.api.model.plan.PlanProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.plan;
import com.atlassian.bamboo.specs.api.builders.plan.Plan;
import com.atlassian.bamboo.specs.api.builders.plan.branches.PlanBranchManagement;
import com.atlassian.bamboo.specs.api.builders.plan.dependencies.Dependencies;
import com.atlassian.bamboo.specs.api.codegen.annotations.Builder;
import com.atlassian.bamboo.specs.api.codegen.annotations.CodeGeneratorName;
import com.atlassian.bamboo.specs.api.codegen.annotations.ConstructFrom;
import com.atlassian.bamboo.specs.api.codegen.annotations.SkipCodeGen;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooKeyProperties;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.RootEntityProperties;
import com.atlassian.bamboo.specs.api.model.VariableProperties;
import com.atlassian.bamboo.specs.api.model.label.LabelProperties;
import com.atlassian.bamboo.specs.api.model.notification.EmptyNotificationsListProperties;
import com.atlassian.bamboo.specs.api.model.notification.NotificationProperties;
import com.atlassian.bamboo.specs.api.model.plan.branches.PlanBranchConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.plan.branches.PlanBranchManagementProperties;
import com.atlassian.bamboo.specs.api.model.plan.configuration.PluginConfigurationProperties;
import com.atlassian.bamboo.specs.api.model.plan.dependencies.DependenciesProperties;
import com.atlassian.bamboo.specs.api.model.project.ProjectProperties;
import com.atlassian.bamboo.specs.api.model.repository.PlanRepositoryLinkProperties;
import com.atlassian.bamboo.specs.api.model.repository.VcsRepositoryBranchProperties;
import com.atlassian.bamboo.specs.api.model.trigger.TriggerProperties;
import com.atlassian.bamboo.specs.api.rsbs.RepositoryStoredSpecsData;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import com.atlassian.bamboo.specs.api.validators.common.ValidationContext;
import com.atlassian.bamboo.specs.api.validators.common.ValidationProblem;
import com.atlassian.bamboo.specs.api.validators.plan.PlanValidator;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
import static com.atlassian.bamboo.specs.api.builders.plan.Plan.TYPE;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNoErrors;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkRequired;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkThat;
@Builder(Plan.class)
@ConstructFrom({"project", "name", "key"})
@Immutable
public final class PlanProperties extends AbstractPlanProperties implements RootEntityProperties {
private final ProjectProperties project;
private final List stages;
@CodeGeneratorName("com.atlassian.bamboo.specs.codegen.emitters.plan.PlanRepositoriesEmitter")
private final List repositories;
private final List repositoryBranches;
private final List triggers;
private final List variables;
private final PlanBranchManagementProperties planBranchManagementProperties;
private final DependenciesProperties dependenciesProperties;
@Nullable
@SkipCodeGen
private final RepositoryStoredSpecsData repositoryStoredSpecsData;
private final List notifications;
@CodeGeneratorName(value = "com.atlassian.bamboo.specs.codegen.emitters.plan.PlanLabelsEmitter")
private final List labels;
private final PlanBranchConfigurationProperties planBranchConfiguration;
private PlanProperties() {
project = null;
stages = Collections.emptyList();
repositories = Collections.emptyList();
triggers = Collections.emptyList();
variables = Collections.emptyList();
planBranchManagementProperties = EntityPropertiesBuilders.build(new PlanBranchManagement());
dependenciesProperties = EntityPropertiesBuilders.build(new Dependencies());
repositoryStoredSpecsData = null;
notifications = Collections.emptyList();
repositoryBranches = Collections.emptyList();
labels = Collections.emptyList();
planBranchConfiguration = null;
}
public PlanProperties(final BambooOidProperties oid,
final BambooKeyProperties key,
final String name,
final String description,
final ProjectProperties project,
final List stages,
final List repositories,
final List triggers,
final List variables,
final boolean enabled,
final Collection pluginConfigurations,
final PlanBranchManagementProperties planBranchManagementProperties,
final DependenciesProperties dependenciesProperties,
@Nullable final RepositoryStoredSpecsData repositoryStoredSpecsData,
@NotNull final List notifications,
final List repositoryBranches,
final List labels,
@Nullable PlanBranchConfigurationProperties planBranchConfiguration) throws PropertiesValidationException {
super(oid, key, name, description, enabled, pluginConfigurations);
this.triggers = Collections.unmodifiableList(new ArrayList<>(triggers));
this.variables = Collections.unmodifiableList(new ArrayList<>(variables));
this.repositories = Collections.unmodifiableList(new ArrayList<>(repositories));
this.project = project;
this.stages = Collections.unmodifiableList(new ArrayList<>(stages));
this.planBranchManagementProperties = planBranchManagementProperties;
this.dependenciesProperties = dependenciesProperties;
this.repositoryStoredSpecsData = repositoryStoredSpecsData;
this.notifications = Collections.unmodifiableList(new ArrayList<>(notifications));
this.repositoryBranches = Collections.unmodifiableList(new ArrayList<>(repositoryBranches));
this.labels = Collections.unmodifiableList(new ArrayList<>(labels));
this.planBranchConfiguration = planBranchConfiguration;
validate();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlanProperties that = (PlanProperties) o;
return Objects.equals(getOid(), that.getOid()) &&
Objects.equals(getKey(), that.getKey()) &&
Objects.equals(getName(), that.getName()) &&
Objects.equals(getDescription(), that.getDescription()) &&
isEnabled() == that.isEnabled() &&
Objects.equals(getPluginConfigurations(), that.getPluginConfigurations()) &&
Objects.equals(getProject(), that.getProject()) &&
Objects.equals(getStages(), that.getStages()) &&
Objects.equals(getRepositories(), that.getRepositories()) &&
Objects.equals(getTriggers(), that.getTriggers()) &&
Objects.equals(getVariables(), that.getVariables()) &&
Objects.equals(getPlanBranchManagementProperties(), that.getPlanBranchManagementProperties()) &&
Objects.equals(getDependenciesProperties(), that.getDependenciesProperties()) &&
Objects.equals(getRepositoryStoredSpecsData(), that.getRepositoryStoredSpecsData()) &&
Objects.equals(getNotifications(), that.getNotifications()) &&
Objects.equals(getRepositoryBranches(), that.getRepositoryBranches()) &&
Objects.equals(getLabels(), that.getLabels()) &&
Objects.equals(getPlanBranchConfiguration(), that.getPlanBranchConfiguration());
}
@Override
public int hashCode() {
return Objects.hashCode(getKey());
}
@NotNull
public ProjectProperties getProject() {
return project;
}
@NotNull
public List getStages() {
return stages;
}
@NotNull
public PlanBranchManagementProperties getPlanBranchManagementProperties() {
return planBranchManagementProperties;
}
@NotNull
public DependenciesProperties getDependenciesProperties() {
return dependenciesProperties;
}
@NotNull
public List getVariables() {
return variables != null ? variables : Collections.emptyList();
}
@NotNull
public List getTriggers() {
return triggers != null ? triggers : Collections.emptyList();
}
@NotNull
public List getRepositories() {
return repositories;
}
@NotNull
public List getRepositoryBranches() {
return repositoryBranches;
}
@Nullable
public RepositoryStoredSpecsData getRepositoryStoredSpecsData() {
return repositoryStoredSpecsData;
}
public List getNotifications() {
return notifications;
}
@NotNull
public List getLabels() {
return labels;
}
@Nullable
public PlanBranchConfigurationProperties getPlanBranchConfiguration() {
return planBranchConfiguration;
}
@Override
public void validate() {
super.validate();
final ValidationContext context = ValidationContext.of("Plan");
checkRequired(context.with("project"), project);
checkRequired(context.with("stages"), stages);
checkRequired(context.with("planBranchManagementProperties"), planBranchManagementProperties);
checkRequired(context.with("dependenciesProperties"), dependenciesProperties);
checkRequired(context.with("notifications"), notifications);
checkRequired(context.with("labels"), labels);
checkNoErrors(PlanValidator.validate(this));
checkThat("EmptyNotificationsList must be the only element on the notifications list",
emptyListMustBeTheOnlyElement(notifications, EmptyNotificationsListProperties.class));
validateRepositoryBranches(context);
}
@NotNull
@Override
public String humanReadableType() {
return TYPE;
}
@NotNull
@Override
public String humanReadableId() {
if (getKey() != null) {
if (project != null && project.getKey() != null) {
return String.format("%s %s-%s", TYPE, project.getKey().getKey(), getKey().getKey());
} else {
return String.format("%s %s", TYPE, getKey().getKey());
}
} else {
return String.format("%s ", TYPE);
}
}
private void validateRepositoryBranches(final ValidationContext context) {
final Set repositoryNames = repositories.stream()
.map(r -> {
if (r.getRepositoryDefinition().hasParent()) {
return r.getRepositoryDefinition().getParentName();
} else {
return r.getRepositoryDefinition().getName();
}
}).collect(Collectors.toSet());
final Set usedNames = new HashSet<>();
final List problems = new ArrayList<>();
for (final VcsRepositoryBranchProperties branch : getRepositoryBranches()) {
if (usedNames.contains(branch.getRepositoryName())) {
problems.add(new ValidationProblem(context, "Duplicate branch definition for repository " + branch.getRepositoryName()));
}
usedNames.add(branch.getRepositoryName());
if (!repositoryNames.contains(branch.getRepositoryName())) {
String availableRepositories;
if(repositoryNames.isEmpty()) {
availableRepositories = " No repositories defined.";
} else {
availableRepositories = " Should be one from list: " + String.join(",", repositoryNames);
}
problems.add(new ValidationProblem(context, "Branch defined for unknown repository '" + branch.getRepositoryName() + "'." + availableRepositories));
}
}
if (!problems.isEmpty()) {
throw new PropertiesValidationException(problems);
}
}
private boolean emptyListMustBeTheOnlyElement(List list, Class> aClass) {
return list.size()==1 || list.stream().noneMatch(aClass::isInstance);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy