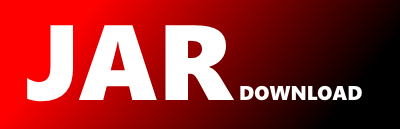
com.atlassian.bamboo.specs.api.model.plan.branches.PlanBranchManagementProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.plan.branches;
import com.atlassian.bamboo.specs.api.builders.plan.branches.PlanBranchManagement;
import com.atlassian.bamboo.specs.api.codegen.annotations.CodeGeneratorName;
import com.atlassian.bamboo.specs.api.codegen.annotations.DefaultFieldValues;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.EntityProperties;
import com.atlassian.bamboo.specs.api.model.trigger.TriggerProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import com.atlassian.bamboo.specs.api.validators.common.ValidationContext;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.Objects;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkRequired;
@Immutable
public final class PlanBranchManagementProperties implements EntityProperties {
public enum TriggeringOption {
INHERITED, MANUAL, CUSTOM;
}
public enum NotificationStrategy {
NOTIFY_COMMITTERS, INHERIT, NONE
}
@CodeGeneratorName("com.atlassian.bamboo.specs.codegen.emitters.plan.branches.CreatePlanBranchesEmitter")
private CreatePlanBranchesProperties createPlanBranch;
private BranchCleanupProperties deletePlanBranch;
@CodeGeneratorName("com.atlassian.bamboo.specs.codegen.emitters.plan.branches.TriggeringOptionEmitter")
private TriggeringOption triggeringOption;
private TriggerProperties defaultTrigger;
@CodeGeneratorName("com.atlassian.bamboo.specs.codegen.emitters.plan.branches.NotificationStrategyEmitter")
private NotificationStrategy notificationStrategy;
private BranchIntegrationProperties branchIntegrationProperties;
private boolean issueLinkingEnabled;
// result.put("merging", exportBranchIntegrationConfiguration(monitoringConfiguration.getDefaultBranchIntegrationConfiguration(), plan.getPlanKey()));
// SerializableConfigMapHelper.putIfNotEmpty(result, "customConfiguration", hierarchicalConfigurationExporter.generateDataMap(monitoringConfiguration.getCustomConfiguration()));
private PlanBranchManagementProperties() {
}
public PlanBranchManagementProperties(final CreatePlanBranchesProperties createPlanBranch,
final BranchCleanupProperties deletePlanBranch,
final TriggeringOption triggeringOption,
final TriggerProperties defaultTrigger,
final NotificationStrategy notificationStrategy,
final BranchIntegrationProperties branchIntegrationProperties,
final boolean issueLinkingEnabled) throws PropertiesValidationException {
this.createPlanBranch = createPlanBranch;
this.deletePlanBranch = deletePlanBranch;
this.issueLinkingEnabled = issueLinkingEnabled;
this.triggeringOption = triggeringOption;
this.defaultTrigger = defaultTrigger;
this.notificationStrategy = notificationStrategy;
this.branchIntegrationProperties = branchIntegrationProperties;
validate();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlanBranchManagementProperties that = (PlanBranchManagementProperties) o;
return isIssueLinkingEnabled() == that.isIssueLinkingEnabled() &&
Objects.equals(getCreatePlanBranch(), that.getCreatePlanBranch()) &&
Objects.equals(getDeletePlanBranch(), that.getDeletePlanBranch()) &&
getTriggeringOption() == that.getTriggeringOption() &&
getDefaultTrigger() == that.getDefaultTrigger() &&
getNotificationStrategy() == that.getNotificationStrategy() &&
Objects.equals(getBranchIntegrationProperties(), that.getBranchIntegrationProperties());
}
@Override
public int hashCode() {
return Objects.hash(getCreatePlanBranch(), getDeletePlanBranch(), getTriggeringOption(), getDefaultTrigger(), getNotificationStrategy(), getBranchIntegrationProperties(), isIssueLinkingEnabled());
}
@Nullable
public CreatePlanBranchesProperties getCreatePlanBranch() {
return createPlanBranch;
}
@Nullable
public BranchCleanupProperties getDeletePlanBranch() {
return deletePlanBranch;
}
public boolean isIssueLinkingEnabled() {
return issueLinkingEnabled;
}
@Nullable
public TriggeringOption getTriggeringOption() {
return triggeringOption;
}
@Nullable
public TriggerProperties getDefaultTrigger() {
return defaultTrigger;
}
@Nullable
public NotificationStrategy getNotificationStrategy() {
return notificationStrategy;
}
@Nullable
public BranchIntegrationProperties getBranchIntegrationProperties() {
return branchIntegrationProperties;
}
@Override
public void validate() {
final ValidationContext context = ValidationContext.of("Branch monitoring");
checkRequired(context.with("createPlanBranch"), createPlanBranch);
checkRequired(context.with("deletePlanBranch"), deletePlanBranch);
checkRequired(context.with("triggeringOption"), triggeringOption);
checkRequired(context.with("notificationStrategy"), notificationStrategy);
checkRequired(context.with("branchIntegrationProperties"), branchIntegrationProperties);
if (triggeringOption == TriggeringOption.CUSTOM) {
checkRequired(context.with("defaultTrigger"), defaultTrigger);
}
}
@DefaultFieldValues
private PlanBranchManagementProperties defaults() {
return EntityPropertiesBuilders.build(new PlanBranchManagement());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy