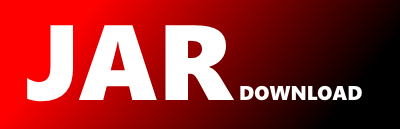
com.atlassian.bamboo.specs.api.model.project.ProjectProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.project;
import com.atlassian.bamboo.specs.api.builders.project.Project;
import com.atlassian.bamboo.specs.api.codegen.annotations.Builder;
import com.atlassian.bamboo.specs.api.codegen.annotations.SkipCodeGen;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooKeyProperties;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.RootEntityProperties;
import com.atlassian.bamboo.specs.api.model.VariableProperties;
import com.atlassian.bamboo.specs.api.model.credentials.SharedCredentialsProperties;
import com.atlassian.bamboo.specs.api.model.repository.VcsRepositoryProperties;
import com.atlassian.bamboo.specs.api.rsbs.RepositoryStoredSpecsData;
import com.atlassian.bamboo.specs.api.validators.project.ProjectValidator;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import static com.atlassian.bamboo.specs.api.builders.project.Project.TYPE;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNoErrors;
@Builder(Project.class)
@Immutable
public final class ProjectProperties implements RootEntityProperties {
private BambooOidProperties oid;
private BambooKeyProperties key;
private String name;
private String description;
private final List variables;
private final List sharedCredentials;
private final List repositories;
@Nullable
@SkipCodeGen
private final RepositoryStoredSpecsData repositoryStoredSpecsData;
private ProjectProperties() {
variables = Collections.emptyList();
sharedCredentials = Collections.emptyList();
repositories = Collections.emptyList();
repositoryStoredSpecsData = null;
}
public ProjectProperties(final BambooOidProperties oid,
final BambooKeyProperties key,
final String name,
final String description,
@NotNull final List variables,
@NotNull final List sharedCredentials,
@NotNull final List repositories,
@Nullable final RepositoryStoredSpecsData repositoryStoredSpecsData) throws PropertiesValidationException {
this.oid = oid;
this.key = key;
this.name = name;
this.description = description;
this.variables = Collections.unmodifiableList(new ArrayList<>(variables));
this.sharedCredentials = Collections.unmodifiableList(new ArrayList<>(sharedCredentials));
this.repositories = Collections.unmodifiableList(new ArrayList<>(repositories));
this.repositoryStoredSpecsData = repositoryStoredSpecsData;
validate();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProjectProperties that = (ProjectProperties) o;
return Objects.equals(getOid(), that.getOid()) &&
Objects.equals(getKey(), that.getKey()) &&
Objects.equals(getName(), that.getName()) &&
Objects.equals(getDescription(), that.getDescription()) &&
Objects.equals(getVariables(), that.getVariables()) &&
Objects.equals(getSharedCredentials(), that.getSharedCredentials()) &&
Objects.equals(getRepositories(), that.getRepositories()) &&
Objects.equals(getRepositoryStoredSpecsData(), that.getRepositoryStoredSpecsData());
}
@Override
public int hashCode() {
return Objects.hash(getOid(), getKey(), getName(), getDescription(), getRepositoryStoredSpecsData());
}
@Nullable
public BambooOidProperties getOid() {
return oid;
}
@Nullable
public BambooKeyProperties getKey() {
return key;
}
@NotNull
public String getName() {
return name;
}
@Nullable
public String getDescription() {
return description;
}
@NotNull
public List getVariables() {
return variables != null ? variables : Collections.emptyList();
}
@NotNull
public List getSharedCredentials() {
return sharedCredentials != null ? sharedCredentials : Collections.emptyList();
}
@NotNull
public List getRepositories() {
return repositories != null ? repositories : Collections.emptyList();
}
@Nullable
public RepositoryStoredSpecsData getRepositoryStoredSpecsData() {
return repositoryStoredSpecsData;
}
@Override
public void validate() {
checkNoErrors(ProjectValidator.validate(this));
}
@NotNull
@Override
public String humanReadableType() {
return TYPE;
}
@NotNull
@Override
public String humanReadableId() {
if (key != null) {
return String.format("%s %s", TYPE, key.getKey());
} else {
return String.format("%s ", TYPE);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy