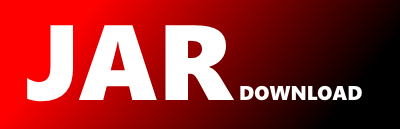
com.atlassian.bamboo.specs.api.builders.Variable Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.builders;
import com.atlassian.bamboo.specs.api.model.VariableProperties;
import com.atlassian.bamboo.specs.api.validators.common.ImporterUtils;
import org.jetbrains.annotations.NotNull;
/**
* Represents a Bamboo variable.
*/
public class Variable extends EntityPropertiesBuilder {
private String name;
private String value;
private boolean createOnly;
/**
* Specifies a variable with given name and value.
*
* @param name name of the variable
* @param value value of the variable
*/
public Variable(@NotNull String name, @NotNull String value) {
ImporterUtils.checkNotNull("name", name);
ImporterUtils.checkNotNull("value", value);
this.name = name;
this.value = value;
}
/**
* Sets variable name.
*
* Variables with names containing words like 'password', 'secret', 'passphrase' are considered 'secret' and are
* encrypted when stored by Bamboo.
*/
public Variable name(@NotNull String name) {
ImporterUtils.checkNotNull("name", name);
this.name = name;
return this;
}
/**
* Sets variable value.
*
* In case of 'secret' variables, both encrypted and unencrypted forms are valid.
*
* @see Encryption in Bamboo Specs
*/
public Variable value(@NotNull String value) {
ImporterUtils.checkNotNull("value", value);
this.value = value;
return this;
}
/**
* Indicates that variable is automatically updated by build or deployment process. In such a case, the
* variable is created and it's value set only if it doesn't already exist.
*
* @since 6.10
*/
public Variable createOnly() {
this.createOnly = true;
return this;
}
protected VariableProperties build() {
return new VariableProperties(name, value, createOnly);
}
}