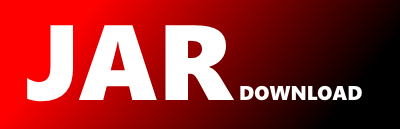
com.atlassian.bamboo.specs.api.model.deployment.DeploymentProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.deployment;
import com.atlassian.bamboo.specs.api.codegen.annotations.ConstructFrom;
import com.atlassian.bamboo.specs.api.codegen.annotations.SkipCodeGen;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.RootEntityProperties;
import com.atlassian.bamboo.specs.api.model.plan.PlanIdentifierProperties;
import com.atlassian.bamboo.specs.api.rsbs.RepositoryStoredSpecsData;
import com.atlassian.bamboo.specs.api.validators.common.ValidationContext;
import com.atlassian.bamboo.specs.api.validators.common.ValidationProblem;
import com.atlassian.bamboo.specs.api.validators.common.ValidationUtils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import static com.atlassian.bamboo.specs.api.builders.deployment.Deployment.TYPE;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNoErrors;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkRequired;
import static org.apache.commons.lang3.StringUtils.defaultString;
@ConstructFrom({"plan", "name"})
public class DeploymentProperties implements RootEntityProperties {
private BambooOidProperties oid;
private PlanIdentifierProperties plan;
private String name;
private String description;
private ReleaseNamingProperties releaseNaming;
private List environments = new ArrayList<>();
@Nullable
@SkipCodeGen
private final RepositoryStoredSpecsData repositoryStoredSpecsData;
private DeploymentProperties() {
environments = Collections.emptyList();
releaseNaming = new ReleaseNamingProperties();
repositoryStoredSpecsData = null;
}
public DeploymentProperties(@Nullable BambooOidProperties oid,
@NotNull PlanIdentifierProperties plan,
@NotNull String name,
@Nullable String description,
@NotNull ReleaseNamingProperties releaseNaming,
@NotNull List environments,
@Nullable final RepositoryStoredSpecsData repositoryStoredSpecsData) {
this.oid = oid;
this.plan = plan;
this.name = name;
this.description = description;
this.releaseNaming = releaseNaming;
this.environments = Collections.unmodifiableList(new ArrayList<>(environments));
this.repositoryStoredSpecsData = repositoryStoredSpecsData;
validate();
}
@Nullable
public BambooOidProperties getOid() {
return oid;
}
public PlanIdentifierProperties getPlan() {
return plan;
}
public String getName() {
return name;
}
public String getDescription() {
return description;
}
public ReleaseNamingProperties getReleaseNaming() {
return releaseNaming;
}
public List getEnvironments() {
return environments;
}
@Nullable
public RepositoryStoredSpecsData getRepositoryStoredSpecsData() {
return repositoryStoredSpecsData;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DeploymentProperties that = (DeploymentProperties) o;
return Objects.equals(getOid(), that.getOid()) &&
Objects.equals(getPlan(), that.getPlan()) &&
Objects.equals(getName(), that.getName()) &&
Objects.equals(getDescription(), that.getDescription()) &&
Objects.equals(getReleaseNaming(), that.getReleaseNaming()) &&
Objects.equals(getRepositoryStoredSpecsData(), that.getRepositoryStoredSpecsData()) &&
Objects.equals(getEnvironments(), that.getEnvironments());
}
@Override
public int hashCode() {
return Objects.hash(getOid(), getPlan(), getName(), getDescription(), getReleaseNaming(), getRepositoryStoredSpecsData(), getEnvironments());
}
@NotNull
@Override
public String humanReadableType() {
return TYPE;
}
@NotNull
@Override
public String humanReadableId() {
return String.format("%s %s", TYPE, defaultString(name, ""));
}
@Override
public void validate() {
final ValidationContext context = ValidationContext.of("Deployment");
ValidationUtils.validateName(context, name);
checkRequired(context.with("plan"), plan);
checkRequired(context.with("releaseNaming"), releaseNaming);
//validate environment names are unique
final Set environmentNames = new HashSet<>();
final ArrayList errors = new ArrayList<>();
for (EnvironmentProperties env : environments) {
if (environmentNames.contains(env.getName())) {
errors.add(new ValidationProblem("Duplicate environment name " + env.getName()));
}
environmentNames.add(env.getName());
}
checkNoErrors(errors);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy