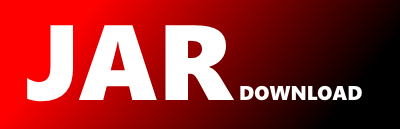
com.atlassian.bamboo.specs.api.model.plan.AbstractPlanProperties Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.api.model.plan;
import com.atlassian.bamboo.specs.api.builders.BambooKey;
import com.atlassian.bamboo.specs.api.exceptions.PropertiesValidationException;
import com.atlassian.bamboo.specs.api.model.BambooKeyProperties;
import com.atlassian.bamboo.specs.api.model.BambooOidProperties;
import com.atlassian.bamboo.specs.api.model.EntityProperties;
import com.atlassian.bamboo.specs.api.model.plan.configuration.PluginConfigurationProperties;
import com.atlassian.bamboo.specs.api.util.EntityPropertiesBuilders;
import com.atlassian.bamboo.specs.api.validators.common.ValidationProblem;
import com.atlassian.bamboo.specs.api.validators.plan.AbstractPlanValidator;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
import static com.atlassian.bamboo.specs.api.validators.common.ImporterUtils.checkNoErrors;
@Immutable
public abstract class AbstractPlanProperties implements EntityProperties {
private BambooKeyProperties key;
private BambooOidProperties oid;
private String name;
private String description;
private boolean enabled;
private List pluginConfigurations;
AbstractPlanProperties() {
enabled = true;
description = "";
pluginConfigurations = Collections.emptyList();
}
public AbstractPlanProperties(final BambooOidProperties oid,
final BambooKeyProperties key,
final String name,
final String description,
final boolean enabled,
final Collection pluginConfigurations) throws PropertiesValidationException {
this.key = key;
this.oid = oid;
this.name = name;
this.description = description;
this.enabled = enabled;
this.pluginConfigurations = Collections.unmodifiableList(new ArrayList(pluginConfigurations));
}
@NotNull
private BambooKeyProperties toPlanIdentifierUnsafe() throws PropertiesValidationException {
final BambooKey jobIdentifier = new BambooKey(key.getKey());
return EntityPropertiesBuilders.build(jobIdentifier);
}
@NotNull
public BambooKeyProperties toPlanIdentifier() {
try {
return toPlanIdentifierUnsafe();
} catch (PropertiesValidationException e) {
final String errors = e.getErrors().stream()
.map(ValidationProblem::getMessage)
.collect(Collectors.joining(", "));
throw new IllegalStateException(errors);
}
}
@Nullable
public BambooKeyProperties getKey() {
return key;
}
public boolean isKeyDefined() {
return key != null;
}
@Nullable
public BambooOidProperties getOid() {
return oid;
}
public boolean isOidDefined() {
return oid != null;
}
@NotNull
public String getName() {
return name;
}
@Nullable
public String getDescription() {
return description;
}
public boolean isEnabled() {
return enabled;
}
@NotNull
public List getPluginConfigurations() {
return pluginConfigurations;
}
@Override
public void validate() {
checkNoErrors(AbstractPlanValidator.validate(this));
toPlanIdentifierUnsafe();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy